Questions
Find answers to frequently asked development questions. For information about Better Stack products, explore our docs.
How to View PostgreSQL logs?
Viewing PostgreSQL logs can help you monitor and troubleshoot your database server. The steps to view the logs may vary depending on your operating system and PostgreSQL installation method. Here a...
What are Syslog formats?
Syslog is a standard protocol for logging and sending messages between network devices, including servers, routers, switches, and other networking equipment. Syslog messages typically contain infor...
How to disable logging when running tests in Python
To disable logging when running tests in Python, you can temporarily adjust the log level or remove the log handlers used during the test execution. This will prevent logs from being displayed or s...
How to log uncaught exceptions in C#
In C#, you can log uncaught exceptions using the AppDomain.UnhandledException event and a logging framework like log4net or NLog. The AppDomain.UnhandledException event allows you to handle uncaugh...
How to log uncaught exceptions in Java
In Java, uncaught exceptions can be logged using a combination of the Thread.UncaughtExceptionHandler interface and the setDefaultUncaughtExceptionHandler method. This allows you to define a global...
How to color logging output in Go
In Go, you can colorize logging output by using third-party libraries that support colored logging. One popular library for this purpose is "logrus," which provides a flexible logging framework wit...
How to log to file and console simultaneously in Python
To log to both a file and the console simultaneously in Python, you need to set up two handlers for the logger: one for writing logs to a file and another for printing logs to the console. The logg...
How to log a Python error with debug information (stack trace)
To log a Python error with debug information, including the stack trace, you can use the logging module. The logging module provides a flexible way to record messages from your Python code, includi...
How to log to file and console in Ruby
To log to both a file and the console (standard output) in Ruby, you can create two logger instances: one for the file and another for the console. Both loggers will be configured to log messages a...
How to log rotate in Ruby
In Ruby, you can implement log rotation by using a combination of the Logger class and external tools or libraries. Log rotation involves managing log files to prevent them from becoming too large ...
How to format log message in Ruby
In Ruby, you can format log messages using the built-in Logger class. The Logger class provides various options for customizing the log message format. You can include timestamps, log levels, and o...
How to change log level in Ruby
In Ruby, the logging level determines which log messages get recorded based on their severity. Ruby's built-in Logger class provides a simple way to handle logging and allows you to change the log ...
How to setup a cron job in Windows?
To set up a Cron job in Windows, you can use the Task Scheduler utility. Here are the steps to create and schedule a Cron job using Task Scheduler: 🔠Want to get alerted when your Cron doesn’t run ...
How to run Cron jobs in Go?
To run Cron jobs in Go, you can use a Go package called robfig/cron. Here are the steps to create and schedule a Go program using robfig/cron: 🔠Want to get alerted when your Cron doesn’t run corre...
How to run Cron jobs in Node.js?
To run Cron jobs in Node.js, you can use a Node.js package called node-cron. Here are the steps to create and schedule a Node.js script using node-cron: Install node-cron You can install node-cron ...
How to run Cron jobs in Python?
To run Cron jobs in Python, you can create a Python script with the code you want to run and schedule it to run using Cron. Here are the steps to create and schedule a Python script: 🔠Want to get ...
How to run Cron jobs in Java?
To run Cron jobs in Java, you can use the Quartz Scheduler library. Here are the steps to create and schedule a Java program using Quartz Scheduler: 🔠Want to get alerted when your Cron doesn’t run...
How to run Cron jobs in PHP?
To run Cron jobs in PHP, you can create a PHP script with the code you want to run and schedule it to run using Cron. Here are the steps to create and schedule a PHP script: 🔠Want to get alerted w...
How to run Cron jobs in Rails?
To run Cron jobs in a Rails application, you can use a gem called whenever. This gem allows you to define your Cron jobs in Ruby syntax and generates a crontab file for you. Here are the steps to u...
How to fix cron jobs that don't run?
If your Cron Jobs are not running, there could be several reasons why they are failing. Here are some common issues and solutions that you can try: 🔠Want to get alerted when your Cron doesn’t run ...
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us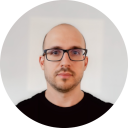
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github
Thank you to everyone who
Here is to all the fantastic people that are contributing and sharing their amazing projects: Thank you!