How to color logging output in Go
In Go, you can colorize logging output by using third-party libraries that support colored logging. One popular library for this purpose is "logrus," which provides a flexible logging framework with color support. Here's how you can use it to add colors to your logging output:
Install the logrus package using go get
:
go get github.com/sirupsen/logrus
Import the logrus package in your Go code:
import (
"github.com/sirupsen/logrus"
)
Set up the logrus logger:
func setupLogger() *logrus.Logger {
logger := logrus.New()
logger.SetFormatter(&logrus.TextFormatter{
ForceColors: true, // Enable colors in the output
})
return logger
}
func main() {
logger := setupLogger()
// Now, you can use the logger to print colored output
logger.WithFields(logrus.Fields{
"animal": "walrus",
"size": 10,
}).Info("A group of walrus emerges from the ocean")
}
The ForceColors: true
option in the TextFormatter
ensures that the output is colored, regardless of the environment.
Keep in mind that the actual appearance of the colored output may vary depending on the terminal or console you are using to view the logs. Some terminals may not support colors or may need additional configuration.
If you want to use custom colors for different log levels or fields, logrus allows you to customize the color and appearance of the logs further. You can refer to the logrus documentation for more information: https://github.com/sirupsen/logrus
To learn more about logging in Go, visit Better Stack Community.
-
Scaling Go Applications
Learn everything you need to know about building, deploying and scaling Go applications in production.
Guides -
9 Best Go Logging Libraries
This article compares 9 Go logging libraries, discussing and comparing their features, performance, pros and cons, to determine which library is right for you
Guides
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us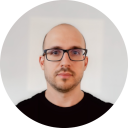
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github