How to change log level in Ruby
In Ruby, the logging level determines which log messages get recorded based on their severity. Ruby's built-in Logger class provides a simple way to handle logging and allows you to change the log level dynamically. Here's how you can change the log level:
require 'logger'
# Create a logger instance
logger = Logger.new(STDOUT)
# Set the initial log level (e.g., INFO)
logger.level = Logger::INFO
# Log some messages at different levels
logger.debug("This is a debug message") # This won't be printed because the log level is set to INFO
logger.info("This is an info message") # This will be printed
logger.warn("This is a warning message") # This will be printed
logger.error("This is an error message") # This will be printed
logger.fatal("This is a fatal message") # This will be printed
# Change the log level to a different level (e.g., DEBUG)
logger.level = Logger::DEBUG
# Log some messages again
logger.debug("This is a debug message") # This will be printed now
logger.info("This is an info message") # This will be printed
logger.warn("This is a warning message") # This will be printed
logger.error("This is an error message") # This will be printed
logger.fatal("This is a fatal message") # This will be printed
In the example above, we create a logger instance and set its log level to Logger::INFO
, which means only messages with severity INFO and above (WARNING, ERROR, FATAL) will be printed. If you change the log level to Logger::DEBUG
, it will include all log messages, including DEBUG level messages.
You can set the log level to any of the following constants:
Logger::DEBUG
Logger::INFO
Logger::WARN
Logger::ERROR
Logger::FATAL
Choose the appropriate log level based on the desired verbosity of your application's logs. It's a good practice to set the log level according to the environment or configuration to control the amount of logging information displayed in different scenarios.
To learn more about logging in Ruby, visit Better Stack Community.
-
Logging in Ruby
Learn how to start logging with Ruby and go from basics to best practices in no time.
Guides -
Best Ruby Application Monitoring Tools in 2023
Ruby APM tools collect key data about the performance bottlenecks, errors and unexpected behavior of the application and provide actionable insights in a human-readable manner.
Comparisons -
Logging in Ruby on Rails
Learn how to start logging with Ruby on Rails and go from basics to best practices in no time.
Guides
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us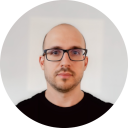
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github