How to format log message in Ruby
In Ruby, you can format log messages using the built-in Logger class. The Logger class provides various options for customizing the log message format. You can include timestamps, log levels, and other relevant information in the log message format. Here's how you can format log messages:
require 'logger'
# Create a logger instance and specify the log file
logger = Logger.new('application.log')
# Customize the log message format
logger.formatter = proc do |severity, datetime, progname, msg|
"#{datetime.strftime('%Y-%m-%d %H:%M:%S')} [#{severity}] #{msg}\n"
end
# Set the log level (e.g., INFO)
logger.level = Logger::INFO
# Log some messages
logger.debug("This is a debug message") # This won't be printed because the log level is set to INFO
logger.info("This is an info message") # This will be printed with the custom format
logger.warn("This is a warning message") # This will be printed with the custom format
logger.error("This is an error message") # This will be printed with the custom format
logger.fatal("This is a fatal message") # This will be printed with the custom format
In this example, we create a logger instance and set it to log messages to a file called application.log. The logger.formatter
attribute is assigned a proc
(a block of code) that customizes the log message format. The proc
takes four arguments:
severity
: The log level of the message (e.g., "DEBUG," "INFO," "WARN," "ERROR," "FATAL").datetime
: The timestamp of when the log message is created.progname
: The program name, which can be set when logging the message (not used in this example).msg
: The actual log message.
In the proc
, we use datetime.strftime
to format the timestamp in the desired way. %Y-%m-%d %H:%M:%S
represents the format "year-month-day hour:minute:second." You can customize the format according to your preferences.
The logger.level
is set to Logger::INFO
, which means only messages with severity INFO and above will be printed. If you want to include DEBUG level messages in the log, you can change the log level to Logger::DEBUG
.
The resulting log messages will be formatted as follows:
2023-07-28 12:34:56 [INFO] This is an info message
2023-07-28 12:34:57 [WARN] This is a warning message
2023-07-28 12:34:58 [ERROR] This is an error message
2023-07-28 12:34:59 [FATAL] This is a fatal message
You can modify the logger.formatter
block to customize the log message format further as per your requirements.
To learn more about logging in Ruby, visit Better Stack Community.
-
How to change log level in Ruby
In Ruby, the logging level determines which log messages get recorded based on their severity. Ruby's built-in Logger class provides a simple way to handle logging and allows you to change the log ...
Questions -
Logging in Ruby
Learn how to start logging with Ruby and go from basics to best practices in no time.
Guides -
Best Ruby Application Monitoring Tools in 2023
Ruby APM tools collect key data about the performance bottlenecks, errors and unexpected behavior of the application and provide actionable insights in a human-readable manner.
Comparisons -
Logging in Ruby on Rails
Learn how to start logging with Ruby on Rails and go from basics to best practices in no time.
Guides
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us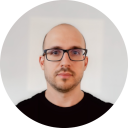
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github