How to log to file and console simultaneously in Python
To log to both a file and the console simultaneously in Python, you need to set up two handlers for the logger: one for writing logs to a file and another for printing logs to the console. The logging
module in Python makes it straightforward to achieve this. Here's an example of how to log to both file and console:
import logging
# Create a logger
logger = logging.getLogger('my_logger')
logger.setLevel(logging.DEBUG)
# Create a formatter to define the log format
formatter = logging.Formatter('%(asctime)s - %(levelname)s - %(message)s')
# Create a file handler to write logs to a file
file_handler = logging.FileHandler('app.log')
file_handler.setLevel(logging.DEBUG)
file_handler.setFormatter(formatter)
# Create a stream handler to print logs to the console
console_handler = logging.StreamHandler()
console_handler.setLevel(logging.INFO) # You can set the desired log level for console output
console_handler.setFormatter(formatter)
# Add the handlers to the logger
logger.addHandler(file_handler)
logger.addHandler(console_handler)
# Now you can log messages with different levels
logger.debug('This is a debug message')
logger.info('This is an info message')
logger.warning('This is a warning message')
logger.error('This is an error message')
In this example, we create two handlers, file_handler
and console_handler
, with different log levels. The file_handler
is set to log all messages with level DEBUG and above to the file "app.log." The console_handler
is set to log messages with level INFO and above to the console. You can adjust the log levels and formats according to your preferences.
With this setup, logs of the specified severity levels will be written to both the file "app.log" and the console simultaneously.
To learn more about logging in Python, visit Better Stack Community.
-
How do I pad a string with zeroes in Python?
You can pad a string with zeros in Python by using the zfill() method. This method adds zeros to the left of the string until it reaches the specified length. Here's an example: s = '123' padded = ...
Questions -
How do I profile a Python script?
By default, Python contains a profiler called cProfile. It not only gives the total running time, but also times each function separately, and tells you how many times each function was called, mak...
Questions -
How do I concatenate two lists in Python?
You can concatenate two lists in Python by using the + operator or the extend() method. Here is an example using the + operator: list1 = [1, 2, 3] list2 = [4, 5, 6] list3 = list1 + list2 print(list...
Questions -
How do I delete a file or folder in Python?
You can use the os module to delete a file or folder in Python. To delete a file, you can use the os.remove() function. This function takes the file path as an argument and deletes the file at that...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us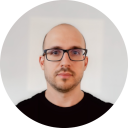
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github