How to log rotate in Ruby
In Ruby, you can implement log rotation by using a combination of the Logger class and external tools or libraries. Log rotation involves managing log files to prevent them from becoming too large and unmanageable. It typically includes creating new log files when the current one reaches a certain size or at specific time intervals.
Here's a basic example of log rotation using the Logger class and a simple custom implementation:
require 'logger'
require 'fileutils'
LOG_FILE = 'application.log'
# Create a logger instance and specify the log file
logger = Logger.new(LOG_FILE, 'daily') # Log will be rotated daily
# Customize the log message format (optional)
logger.formatter = proc do |severity, datetime, progname, msg|
"#{datetime.strftime('%Y-%m-%d %H:%M:%S')} [#{severity}] #{msg}\n"
end
# Set the log level (e.g., INFO)
logger.level = Logger::INFO
# Log some messages
logger.info("This is an info message")
logger.warn("This is a warning message")
logger.error("This is an error message")
logger.fatal("This is a fatal message")
# Rotate the log manually (example, you can implement more sophisticated rules)
def rotate_log(file)
return unless File.exist?(file)
timestamp = Time.now.strftime('%Y%m%d%H%M%S')
rotated_file = "#{file}.#{timestamp}"
FileUtils.mv(file, rotated_file)
end
# Perform log rotation (example, you can implement your own rotation logic)
rotate_log(LOG_FILE)
In the example above, we create a logger instance with the option 'daily'
, which means the log file will be rotated daily. You can use other options for rotation, such as 'weekly'
, 'monthly'
, or you can implement a custom rotation logic by providing a frequency (in seconds) as the second argument to Logger.new
.
For more complex log rotation strategies, you may want to consider using a dedicated log rotation library or a system-level log rotation tool (such as logrotate
on Unix-like systems). These external tools typically offer more advanced features like compression, log retention policies, and log archiving. For instance, on Unix-like systems, you can use logrotate
by creating a configuration file for your application's log file and configuring the rotation rules as needed.
Keep in mind that the above example is a basic illustration, and actual log rotation requirements may vary depending on the specific needs of your application and the environment in which it runs.
To learn more about logging in Ruby, visit Better Stack Community.
-
How to change log level in Ruby
In Ruby, the logging level determines which log messages get recorded based on their severity. Ruby's built-in Logger class provides a simple way to handle logging and allows you to change the log ...
Questions -
Logging in Ruby
Learn how to start logging with Ruby and go from basics to best practices in no time.
Guides -
How to format log message in Ruby
In Ruby, you can format log messages using the built-in Logger class. The Logger class provides various options for customizing the log message format. You can include timestamps, log levels, and o...
Questions -
Logging in Ruby on Rails
Learn how to start logging with Ruby on Rails and go from basics to best practices in no time.
Guides
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us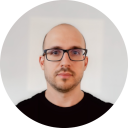
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github