How to log to file and console in Ruby
To log to both a file and the console (standard output) in Ruby, you can create two logger instances: one for the file and another for the console. Both loggers will be configured to log messages at the desired log level and will use different log files for file logging and the console for console logging. Here's an example of how to achieve this:
require 'logger'
# Create a logger instance for file logging
file_logger = Logger.new('application.log', 'daily') # Log will be rotated daily
file_logger.level = Logger::INFO
# Customize the log message format for file_logger (optional)
file_logger.formatter = proc do |severity, datetime, progname, msg|
"#{datetime.strftime('%Y-%m-%d %H:%M:%S')} [#{severity}] #{msg}\n"
end
# Create a logger instance for console logging
console_logger = Logger.new(STDOUT)
console_logger.level = Logger::DEBUG
# Customize the log message format for console_logger (optional)
console_logger.formatter = proc do |severity, datetime, progname, msg|
"#{severity} - #{msg}\n"
end
# Log some messages
file_logger.info("This is an info message") # Will be logged to the file
file_logger.warn("This is a warning message") # Will be logged to the file
console_logger.debug("This is a debug message") # Will be logged to the console
console_logger.info("This is another info message") # Will be logged to the console
In this example, we create two logger instances: file_logger
and console_logger
. The file_logger
is configured to log messages to a file named 'application.log', and the log file will be rotated daily.
The console_logger
is set to log messages to the console using STDOUT
. This means log messages sent to console_logger
will be displayed on the standard output (usually the terminal or console window).
Each logger can have its own log level and log message format, allowing you to customize the behavior for each destination independently.
Please note that the log message format and log rotation settings are optional and can be adjusted based on your specific requirements. The above example is a basic illustration of how to log to both a file and the console in Ruby.
To learn more about logging in Ruby, visit Better Stack Community.
-
How to change log level in Ruby
In Ruby, the logging level determines which log messages get recorded based on their severity. Ruby's built-in Logger class provides a simple way to handle logging and allows you to change the log ...
Questions -
How to format log message in Ruby
In Ruby, you can format log messages using the built-in Logger class. The Logger class provides various options for customizing the log message format. You can include timestamps, log levels, and o...
Questions -
How to log rotate in Ruby
In Ruby, you can implement log rotation by using a combination of the Logger class and external tools or libraries. Log rotation involves managing log files to prevent them from becoming too large ...
Questions -
Logging in Ruby
Learn how to start logging with Ruby and go from basics to best practices in no time.
Guides
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us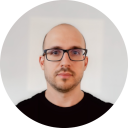
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github