How to log a Python error with debug information (stack trace)
To log a Python error with debug information, including the stack trace, you can use the logging module. The logging module provides a flexible way to record messages from your Python code, including errors, warnings, and debug information. Here's a step-by-step guide on how to achieve this:
Import the logging module:
import logging
Configure the logging settings (optional): Before logging any messages, you can configure the logging settings. This step is optional, but it allows you to customize how the logs are handled, such as specifying the log level, setting the output format, or sending logs to a specific file. For example:
logging.basicConfig(level=logging.DEBUG, format='%(asctime)s - %(levelname)s - %(message)s')
This configuration sets the log level to DEBUG, which will capture all log messages of severity DEBUG and above. The log format includes the timestamp, log level, and log message.
Log the error with the stack trace:
When you encounter an error in your code, you can log it along with its stack trace using the
logging.exception()
method. This method logs the error message along with the traceback. Here's an example:try: # Your code that may raise an error result = 10 / 0 # This will raise a ZeroDivisionError except Exception as e: logging.exception("An error occurred:")
In this example, any exception raised within the try block will be caught, and the error message along with the stack trace will be logged.
Save logs to a file (optional):
If you want to save the logs to a file instead of printing them to the console, you can add a file handler to the logger. Here's how you can do it:
# Configure logging to a file logging.basicConfig(filename='app.log', level=logging.DEBUG, format='%(asctime)s - %(levelname)s - %(message)s') # Continue with the same try-except block to log errors
This will create a file called "app.log" in the same directory as your script and store the log messages in it.
With these steps, you can log Python errors with debug information and have a better understanding of what went wrong in your code, including the stack trace leading up to the error.
To learn more about logging in Python, visit Better Stack Community.
-
How to start an HTTP server in Python?
You can create an HTTP server in python using the http.server module. Starting server from the command line To start a server from a command line, navigate to your projects directory cd /dev/myproj...
Questions -
How to Log to Stdout with Python?
If you are new to logging in Python, please feel free to start with our Introduction to Python logging to get started smoothly. Otherwise, here is how to log to Stdout with Python: Using Basic Conf...
Questions -
How Do I Measure Elapsed Time in Python?
There are several ways to measure elapsed time in Python. Here are a few options: You can use the time module to get the current time in seconds since the epoch (the epoch is a predefined point in ...
Questions -
How can I add new keys to Python dictionary?
You can add a new key-value pair to a dictionary in Python by using the square brackets [] to access the key you want to add and then assigning it a value using the assignment operator =. For examp...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us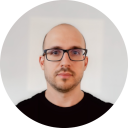
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github