How to log uncaught exceptions in Java
In Java, uncaught exceptions can be logged using a combination of the Thread.UncaughtExceptionHandler
interface and the setDefaultUncaughtExceptionHandler
method. This allows you to define a global handler for uncaught exceptions that occur in your Java application. Here's a step-by-step guide on how to set up logging for uncaught exceptions:
Create a custom UncaughtExceptionHandler
class that implements the Thread.UncaughtExceptionHandler
interface. This class will define how uncaught exceptions are handled, such as logging the exception details.
import java.util.logging.Level;
import java.util.logging.Logger;
public class CustomUncaughtExceptionHandler implements Thread.UncaughtExceptionHandler {
private static final Logger LOGGER = Logger.getLogger(CustomUncaughtExceptionHandler.class.getName());
@Override
public void uncaughtException(Thread t, Throwable e) {
LOGGER.log(Level.SEVERE, "Uncaught exception in thread: " + t.getName(), e);
}
}
Set the custom UncaughtExceptionHandler
as the default handler for uncaught exceptions using the setDefaultUncaughtExceptionHandler
method. You can do this in the main method of your Java application or at an appropriate location where you want to set the global handler.
public class Main {
public static void main(String[] args) {
// Set custom uncaught exception handler
Thread.setDefaultUncaughtExceptionHandler(new CustomUncaughtExceptionHandler());
// Rest of your application code...
}
}
Now, if an uncaught exception occurs in any thread of your Java application, the CustomUncaughtExceptionHandler
class's uncaughtException
method will be called, and it will log the exception details using a logger (in this case, the Java Logger
class).
Make sure to configure the logger to write the logs to a file or another appropriate destination based on your logging requirements. You can configure the logger's level, format, and other settings to suit your needs.
Note: This approach will handle uncaught exceptions in non-daemon threads. If you have daemon threads in your application that might throw uncaught exceptions, you should handle them explicitly within those threads or use a separate mechanism to catch and handle them.
To learn more about logging, visit Better Stack Community.
-
How to run Cron jobs in Java?
To run Cron jobs in Java, you can use the Quartz Scheduler library. Here are the steps to create and schedule a Java program using Quartz Scheduler: 🔠Want to get alerted when your Cron doesn’t run...
Questions -
Java Logging Best Practices
In this comprehensive tutorial, we will delve into the realm of best practices for creating a robust logging system specifically tailored for Java applications
Guides
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us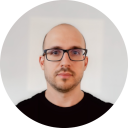
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github