Questions
Find answers to frequently asked development questions. For information about Better Stack products, explore our docs.
SSL Certificate Location on UNIX/Linux
In UNIX/Linux systems, SSL certificate files are often stored in specific directories. The exact location can vary based on the distribution and configuration. Commonly, SSL certificates on UNIX/Li...
How to display a remote SSL certificate details using CLI tools?
You can use various command-line tools to display details of a remote SSL certificate. One common tool is openssl, which provides commands to fetch and examine SSL certificates. Here's an example o...
Must CSRs be generated on the server that will host the SSL certificate?
CSRs (Certificate Signing Requests) are generated for SSL certificates and are used by Certificate Authorities (CAs) to create the SSL certificate. They contain information about the entity request...
Best location to keep SSL certificates and private keys on Ubuntu servers?
On Ubuntu servers, the best practice for storing SSL certificates and private keys is to place them in a directory with restricted access. The standard directory for these files is typically within...
Nginx as Reverse Proxy With Upstream SSL
When using Nginx as a reverse proxy with SSL for upstream servers, it's a common scenario to secure the communication between Nginx and the upstream servers while also handling SSL termination at t...
Remove "www" and redirect to "https" with nginx
To remove the "www" from URLs and redirect all incoming traffic to HTTPS in Nginx, you can use a server block that handles both scenarios. Here's an example configuration: Open your Nginx configura...
How to force or redirect to SSL in nginx?
To force or redirect all incoming traffic to SSL (HTTPS) in Nginx, you can use a server block that handles HTTP requests on port 80 and redirect them to HTTPS. Here's an example configuration: Open...
Multiple domains with SSL on same IP?
Yes, you can host multiple domains with SSL on the same IP address using Server Name Indication (SNI). SNI is an extension of the TLS protocol that allows a server to present multiple SSL certifica...
Generating a self-signed cert with openssl that works in Chrome 58
To create a self-signed certificate that works with Chrome 58 using OpenSSL, you can follow these steps: Generate a private key: Use the following command to generate a private key. This example us...
Multiple SSL domains on the same IP address and same port?
It's possible to host multiple SSL (Secure Socket Layer) domains on the same IP address and port using Server Name Indication (SNI). SNI is an extension to the Transport Layer Security (TLS) protoc...
How to use MDC with thread pools in Java?
In Java, MDC (Mapped Diagnostic Context) is a feature provided by logging frameworks like Log4j and Logback. It allows you to associate key-value pairs with the current thread's execution context, ...
Configuring Log4j Loggers Programmatically
In Log4j, you can configure loggers programmatically using Java code. This allows you to define the logging configuration dynamically at runtime. To configure Log4j loggers programmatically, follow...
How to redirect Docker logs to file?
To redirect Docker container logs to a file, you can use the --log-driver and --log-opt options when running the container. Docker provides several logging drivers, and you can choose one that suit...
How to Customize the time format for Python logging?
To customize the time format for Python logging, you need to modify the formatter used by the logging module. Python's logging module provides a powerful mechanism to control the log message format...
Why you should not use java.util.logging
java.util.logging (JUL) is one of the built-in logging frameworks available in Java. While it's not inherently "bad," there are some reasons why developers may prefer using other logging libraries ...
How to disable Request logs in Python?
To disable request logs in Python, you need to configure your web server or web application framework to suppress logging of HTTP request information. The specific method to disable request logs ca...
How to log all or slow MongoDB queries?
To log all or slow MongoDB queries, you can use MongoDB's built-in logging capabilities and monitoring tools. MongoDB provides various options for capturing query performance and activity. Here's h...
How to log all or slow Redis queries?
Redis does not natively support query logging in the same way that some relational databases like MySQL do. However, you can still gather information about slow Redis commands or monitor Redis acti...
How to log all or slow MySQL queries?
To log all or slow MySQL queries, you'll need to modify the MySQL configuration file and enable query logging. The exact steps may vary depending on your MySQL version and the operating system you'...
How to log all or slow PostgreSQL queries?
To log all or slow queries in PostgreSQL, you can configure the PostgreSQL server's logging options. PostgreSQL provides various settings that allow you to control the level of detail for query log...
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us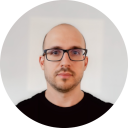
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github
Thank you to everyone who
Here is to all the fantastic people that are contributing and sharing their amazing projects: Thank you!