Configuring Log4j Loggers Programmatically
In Log4j, you can configure loggers programmatically using Java code. This allows you to define the logging configuration dynamically at runtime. To configure Log4j loggers programmatically, follow these steps:
1. Add Log4j Dependency
First, make sure you have the Log4j library added to your project's classpath. You can add the Log4j dependency in your build tool (e.g., Maven, Gradle) or manually include the Log4j JAR files.
2. Create a Java Class to Configure Log4j
Create a Java class where you will configure the Log4j loggers programmatically. This class should typically be executed at the start of your application to ensure the loggers are configured before any log statements are executed.
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import org.apache.logging.log4j.core.config.Configurator;
import org.apache.logging.log4j.core.config.LoggerConfig;
import org.apache.logging.log4j.Level;
public class Log4jProgrammaticConfig {
private static final Logger LOGGER = LogManager.getLogger(Log4jProgrammaticConfig.class);
public static void main(String[] args) {
configureLoggers();
// Now, you can use the configured loggers for logging.
LOGGER.debug("This is a debug message.");
LOGGER.info("This is an info message.");
LOGGER.warn("This is a warning message.");
LOGGER.error("This is an error message.");
}
private static void configureLoggers() {
// Get the root logger configuration
LoggerConfig rootLoggerConfig = Configurator.getRootLogger().get();
// Set the root logger's log level (DEBUG, INFO, WARN, ERROR, FATAL)
rootLoggerConfig.setLevel(Level.DEBUG);
// Create and configure additional loggers
LoggerConfig customLoggerConfig = new LoggerConfig("com.example.myapp.CustomLogger", Level.INFO, false);
Configurator.setLevel(customLoggerConfig.getName(), Level.INFO);
// Add the additional loggers to the logger context
Configurator.setAllLevels(rootLoggerConfig.getName(), rootLoggerConfig.getLevel());
Configurator.getLoggerContext().updateLoggers();
}
}
In this example, we're using the Log4j 2 API to configure loggers programmatically. The configureLoggers()
method sets the log level for the root logger and creates and configures an additional custom logger.
3. Customize the Log4j Configuration
In addition to the basic configuration shown in the example above, you can customize the Log4j configuration programmatically further. For instance, you can set appenders, layouts, and other logging-related settings.
Keep in mind that you can also use XML or properties-based configuration files to configure Log4j, which allows for more complex and dynamic configurations. However, the programmatic configuration can be useful when you need to dynamically change log levels or apply specific settings based on runtime conditions.
To learn more about logging, visit Better Stack Community.
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us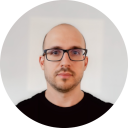
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github