How to disable Request logs in Python?
To disable request logs in Python, you need to configure your web server or web application framework to suppress logging of HTTP request information. The specific method to disable request logs can vary depending on the web server or framework you are using. Below are examples for some popular web frameworks:
Flask
If you are using Flask, you can configure the Flask application to disable request logging using the logging
module. By default, Flask logs requests with the WARNING
level. To disable request logging, you can set the log level to a higher value, such as ERROR
or CRITICAL
, which will prevent request logs from being shown in the console or log files.
Here's an example of how to disable request logs in Flask:
import logging
from flask import Flask
app = Flask(__name__)
# Disable request logging
app.logger.setLevel(logging.ERROR)
Django
In Django, you can control request logging through the application's logging configuration. Django uses the Python logging
module under the hood, and you can configure the loggers in your settings file.
To disable request logging in Django, you can adjust the log level of the relevant logger (usually the django.request
logger) to a higher level than DEBUG
. For example, setting the level to ERROR
will prevent request logs from being displayed.
In your Django settings file (settings.py
), add the following:
LOGGING = {
'version': 1,
'disable_existing_loggers': False,
'handlers': {
'console': {
'level': 'ERROR', # Set the log level to ERROR to disable request logs
'class': 'logging.StreamHandler',
},
},
'loggers': {
'django.request': {
'handlers': ['console'],
'level': 'ERROR',
'propagate': False,
},
},
}
Tornado
If you are using Tornado as your web server, you can configure the Tornado application to disable request logging by adjusting the log_level
parameter in the application settings.
Here's an example of how to disable request logs in Tornado:
import tornado.ioloop
import tornado.web
class MainHandler(tornado.web.RequestHandler):
def get(self):
self.write("Hello, world")
def make_app():
return tornado.web.Application([
(r"/", MainHandler),
], log_level=logging.ERROR) # Set the log_level to ERROR to disable request logs
if __name__ == "__main__":
app = make_app()
app.listen(8888)
tornado.ioloop.IOLoop.current().start()
These examples demonstrate how to disable request logs in popular Python web frameworks. Remember that logging is essential for debugging and monitoring applications, so use this sparingly and ensure you have other mechanisms in place to capture and handle important log information.
To learn more about logging in Python, visit Better Stack Community.
-
How To Color Python Logging Output?
If you are new to logging in Python, please feel free to start with our Introduction to Python logging to get started smoothly. Otherwise, here is how to color python logging output: đź” Want to cent...
Questions -
How to disable logging when running tests in Python
To disable logging when running tests in Python, you can temporarily adjust the log level or remove the log handlers used during the test execution. This will prevent logs from being displayed or s...
Questions -
How To Disable Logging From The Python Request Library?
You can change the log level of the logger taking care of these messages. Setting the level to WARNING will remove the request messages and keep warnings and errors: import logging logging.getLogge...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us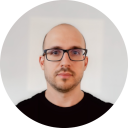
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github