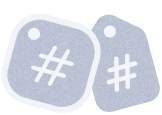
How To Log All Requests From The Python Request Library?
If you are new to logging in Python, please feel free to start with our Introduction to Python logging to get started smoothly. Otherwise, here is how to log all requests from the python request li...
How To Disable Logging From The Python Request Library?
You can change the log level of the logger taking care of these messages. Setting the level to WARNING will remove the request messages and keep warnings and errors: import logging logging.getLogge...
How To Use Logging In Multiple Modules?
It's recommended to have a logger defined in each module like this: import logging logger = logging.getLogger(name) Then in your main program, do the following: import logging.config logging.config...
How to Log to Stdout with Python?
If you are new to logging in Python, please feel free to start with our Introduction to Python logging to get started smoothly. Otherwise, here is how to log to Stdout with Python: Using Basic Conf...
How To Color Python Logging Output?
If you are new to logging in Python, please feel free to start with our Introduction to Python logging to get started smoothly. Otherwise, here is how to color python logging output: 🔭 Want to cent...
How To Write Logs To A File With Python?
If you are new to logging in Python, please feel free to start with our Introduction to Python logging to get started smoothly. Otherwise, here is how to write logs to a file in Python: Using Basic...
How To Log Uncaught Exceptions In Python?
For this, you can use the sys.excepthook that allows us to attach a handler for any unhandled exception: Creating a logger logger = logging.getLogger(name) logging.basicConfig(filename='example.log...
How to log data as JSON with Python
How to Log Data As JSON With Python? The simplest way is to use a custom module.
How To Disable Logging While Running Django Unit Tests?
By Disabling Tests At The Start Of The Application Suppose you want to do it the quick way. In that case, the following line of code will disable any log messages less severe or equal to CRITICAL: ...
How to Get Started with Logging in Flask
Learn how to start logging with Flask and go from basics to best practices in no time.
How to Get Started with Logging in Django
Django comes with an integrated logging module that provides basic as well as advanced logging features. Read on to learn how to start using it in your projects
A Complete Guide to Logging in Python with Loguru
Learn how to install, configure, and use the Loguru framework for logging in Python applications
How to Get Started with Logging in FastAPI
Learn how to add powerful logging to your FastAPI application using Python’s built-in tools. This step-by-step guide covers console logs, JSON formatting, log rotation, and centralized logging with Better Stack to help you monitor and debug your app in production.
Containerizing Flask Applications with Docker
Learn how to containerize a Flask app with Docker for consistent deployment and easy management using Docker Compose.
Vite.js: A Beginner's Guide
Learn how to set up and customize a Vite.js development environment with support for Hot Module Replacement, CSS, assets, and testing using Vitest. Ideal for building fast, modern web apps with minimal configuration
One-Line Python HTTP Server: Quick Start Guide
Learn how to create a simple one-line HTTP server in Python using the built-in `http.server` module. Share files, test sites, and build custom servers—no extra packages needed.
Working with YAML Files in Python
Learn how to read, write, and validate YAML files in Python using PyYAML and PyKwalify. This guide covers parsing, modifying, and structuring configuration data for real-world applications.
Working with CSV Files in Python
Learn how to handle CSV files in Python using the built-in csv module and pandas library. This guide covers everything from basic reading and writing of CSV files to advanced data manipulation and validation techniques, including handling different formats and ensuring data integrity.
Working With JSON Data in Python
Learn how to efficiently handle JSON data in Python with the built-in `json` module. This guide covers serializing Python objects to JSON, deserializing JSON back to Python dictionaries, formatting JSON for readability, and validating JSON data using JSON Schema.
Dependency Injection in Python
Learn how to implement dependency injection in Python, from basic constructor injection to using the `dependency-injector` library for scalable and testable applications.
Getting Started with PyMongo
Learn how to use PyMongo to interact with MongoDB in Python. This beginner-friendly guide covers setting up your project, connecting to a database, and performing essential CRUD operations with clean, modular code examples.
Poetry vs Pip: Choosing the Right Python Package Manager
Compare Python package managers: Pip is ideal for simple projects, while Poetry offers advanced features for managing dependencies, virtual environments, and packaging in modern apps. Choose the right tool for your needs.
Getting Started with Pipenv: A Practical Guide
Learn Pipenv for Python development: installation, dependency management, and troubleshooting. Simplify virtual environments and package handling for more reliable projects.
Getting Started with AnyIO in Python
AnyIO simplifies asynchronous programming in Python by providing a unified interface for different async backends like asyncio and trio. This guide covers key features such as task concurrency, error handling, and timeouts, helping you build efficient, non-blocking applications with ease.
Practical Guide to Asynchronous Programming in Python
Learn how to use Python's `asyncio` library to write efficient, concurrent code. This guide covers async functions, async generators, and semaphores, helping you handle multiple tasks concurrently for improved performance. Ideal for I/O-bound tasks and large datasets.
Introduction to Python Generators
Learn how Python generators work with `yield` and `send()`, enabling efficient, memory-friendly handling of large datasets and infinite sequences. Discover the power of stateful iteration in Python and improve your code's performance.
DuckDB vs SQLite: Choosing the Right Embedded Database
Compare DuckDB and SQLite to find the best embedded database for your needs. Learn their key differences in performance, storage, and use cases for analytics vs. transactional workloads.
A Complete Guide to Python Type Hints
Learn how to use type hints in Python with the typing module and mypy. Improve your code quality, catch bugs early, and make your code easier to read and maintain with modern static typing techniques.
Getting Started with Playwright Testing in Python
Learn how to use Playwright with Python for end-to-end web testing. This comprehensive guide covers everything from basic setup to advanced features with practical examples.
Getting Started with IPython
Learn how to use IPython to boost your Python development workflow with features like interactive debugging, magic commands, tab completion, session history, and extension support
Jinja Templating in Python: A Practical Guide
Jinja (specifically Jinja2, its current major version) was created by Armin Ronacher, the same developer behind the Flask web framework. It draws inspiration from Django's templating system but off...
Introduction to Django ORM
Learn how to use Django’s powerful ORM to create, read, update, and delete database records with Python. This beginner-friendly guide covers models, queries, relationships, aggregations, and performance tips.
An Introduction to Python Subprocess
Learn Python’s `subprocess` module to run commands, capture output, handle errors, and build safer, more flexible automation scripts.
Optimize Your Workflow in Python with pipx
Learn how to use `pipx` to install and manage Python CLI tools in isolated environments. Avoid dependency conflicts, keep tools updated, and streamline your development workflow with this powerful Python utility.
Mastering unittest.mock in Python
Learn how to use Python's unittest.mock library to create effective test doubles that simulate real objects, making your tests more isolated, predictable, and maintainable
DuckDB for Python: A beginner's guide
Learn how to use DuckDB, a fast in-process SQL database for Python, to build powerful data analysis workflows. This guide covers setup, querying CSV and Parquet files, joins, aggregation, and integration with pandas—no server required.
A Beginner's Guide to Unit Testing with Freezegun
Learn how to use Freezegun, a Python library for freezing and simulating time in tests. This step-by-step guide covers setup, time-freezing, advancing time, auto-tick, and testing across time zones using `pytest`. Perfect for writing reliable tests for time-sensitive code.
A Beginner's Guide to Unit Testing with Hypothesis
Discover how to use Hypothesis with pytest to write smarter Python tests, catch edge cases, and improve code quality with less effort. This guide covers setup, examples, and advanced testing tips.
Structural Pattern Matching in Python: A Comprehensive Guide
Learn how to use structural pattern matching in Python to simplify complex conditionals and make your code more readable. This guide covers matching with basic types, dictionaries, guard clauses, and more—introduced in Python 3.10 and enhanced in Python 3.13. Includes clear examples and comparisons with traditional approaches.
Getting Started with Peewee ORM for Python
Learn how to use Peewee, a lightweight and powerful Python ORM, to manage SQLite databases with minimal code. This step-by-step tutorial covers setting up Peewee, defining models, performing CRUD operations, and efficiently handling database queries.
Getting Started with SQLModel for Python
Learn how to use SQLModel, a powerful Python library that combines SQLAlchemy’s ORM with Pydantic’s data validation for seamless database management. This step-by-step tutorial covers setting up SQLModel, creating models, performing CRUD operations, and managing an SQLite database
Getting Started with HTTPX: Python's Modern HTTP Client
Discover how HTTPX modernizes Python HTTP requests with async support and HTTP/2 capabilities. This step-by-step guide covers everything from basic GET requests to advanced authentication patterns, timeout handling, and concurrent API calls—complete with practical code examples that will elevate your web applications' performance and reliability.
Getting Started with TortoiseORM for Python
Learn how to build asynchronous Python applications with TortoiseORM and SQLite in this comprehensive tutorial. Master the fundamentals of async database operations, from setting up your project and defining models to performing CRUD operations efficiently.
TortoiseORM vs SQLAlchemy: An In-Depth Framework Comparison
Discover the key differences between SQLAlchemy and TortoiseORM, two popular Python ORMs. Learn about their performance, async support, query building, migrations, and ideal use cases to help you choose the right ORM for your application.
Getting Started with SQLAlchemy ORM for Python
Learn SQLAlchemy with this step-by-step tutorial! Discover how to set up a SQLAlchemy project with SQLite, define database models, and perform CRUD operations (Create, Read, Update, Delete) using Python. This guide covers everything from database connections to data manipulation, making it perfect for beginners and those looking to enhance their SQLAlchemy skills.
Introduction to PDM: A Python Project and Dependency Manager
Learn how to streamline your Python project management with PDM, a modern dependency manager that follows Python's latest standards. This comprehensive guide covers installation, dependency management, virtual environments, and Python version control, showing you how PDM provides a more efficient alternative to traditional tools like pip and virtualenv
Building Web APIs with Litestar: A Beginner's Guide
SEO Description Learn how to build a high-performance blog API with Litestar and SQLAlchemy in Python. This step-by-step tutorial covers creating a complete RESTful API with CRUD operations, async database interactions, dependency injection, and automatic API documentation. Perfect for Python developers looking to create modern, type-safe web services with minimal boilerplate code.
How to Manage Multiple Python Versions With pyenv
Learn how to use `pyenv` to manage multiple Python versions effortlessly. This comprehensive guide covers installation, version switching, project-specific environments, virtual environments, and development tool management. Optimize your Python workflow with `pyenv` and eliminate version conflicts.
Getting Started with Poetry
Discover how Poetry transforms Python development with streamlined dependency management and virtual environments in one cohesive tool. Learn to create projects, manage dependencies with lockfiles, and organize package groups while ensuring reproducible builds across machines.
A Comprehensive Guide to Profiling in Python
Discover how to quickly identify and resolve performance issues in your Python applications using Python's built-in profiling tools. In this step-by-step guide, you'll explore manual timing, profiling with `cProfile`, creating custom decorators, visualizing profiling data with SnakeViz, and applying practical optimization techniques.
Job Scheduling in Python with APScheduler
Learn how to schedule tasks in Python using APScheduler. This practical guide covers installation, date, interval, and cron triggers, persistent job storage, and best practices to effectively automate background tasks in your Python applications
Managing Python Projects With pyproject.toml
Learn how to manage Python projects efficiently with pyproject.toml. This guide covers configurations, tool integrations, dependency management, and versioning strategies. Discover how this modern approach enhances security, simplifies workflows, and improves maintainability compared to legacy setup.py methods.
Django vs FastAPI: Choosing the Right Python Web Framework
Which Python web framework is right for your project—Django or FastAPI? Django offers a full-stack solution with built-in tools for rapid development, while FastAPI is optimized for high-performance APIs with async support and automatic documentation. This guide compares their architecture, setup, API features, and performance to help you make the best choice.
Building Web APIs with Django Rest Framework: A Beginner's Guide
Build a production-ready REST API with Django Rest Framework in this comprehensive tutorial. Learn to create models, serializers, and viewsets while implementing CRUD operations for a task management system.
Flask vs FastAPI: An In-Depth Framework Comparison
This guide compares Flask and FastAPI, two leading Python web frameworks. Flask offers simplicity and flexibility, while FastAPI provides high performance, async support, and automatic documentation. Explore their architecture, performance, and best use cases to find the right fit for your project.
A Guide to Debugging Python Code with ipdb
Learn how to debug Python applications efficiently with `ipdb`, an enhanced debugger that improves `pdb` with advanced features like better tracebacks, breakpoints, and interactive debugging
A Complete Guide to Timeouts in Python
Learn how to implement effective timeout strategies in Python to prevent application hangs, improve responsiveness, and protect system resources. This guide covers HTTP request timeouts, database timeouts, async timeouts, and best practices for choosing optimal timeout values.
Creating composable CLIs with click in Python
Learn to build powerful Python CLI applications with Click. This guide covers commands, options, arguments, validation, and advanced features like command groups and context management
Implementing OpenTelemetry Metrics in Python Apps
Learn how to implement OpenTelemetry metrics in a Python Flask application to monitor performance, track custom metrics, and gain valuable insights into your app's health.
Linting with Ruff: A Fast Python Linter
Ruff is a fast Python linter and code formatter written in Rust that has rapidly gained popularity in the Python ecosystem. It includes all the standard features expected in any linting framework,...
Building Web APIs with FastAPI: A Beginner's Guide
Learn how to build a production-ready RESTful API using FastAPI, SQLModel, and Pydantic. This step-by-step guide covers database integration with SQLite, CRUD operations, and automatic validation, helping you create scalable and high-performance web services
Django Error Handling Patterns
Learn essential Django error handling patterns to create applications that handle failures gracefully. This guide covers custom exceptions, middleware, and logging strategies.
Building Web APIs with Flask: A Beginner's Guide
Do you want to build a RESTful blog API with Flask? This step-by-step guide walks you through creating, reading, updating, and deleting blog posts using Flask-SQLAlchemy and Flask-Smorest. Learn how to set up a database, define models, validate requests, handle errors, and follow REST best practices—all while generating automatic API documentation.
Django Docker Best Practices: 7 Dos and Don'ts
Deploying Django with Docker? Avoid common pitfalls and follow these 7 best practices to ensure security, scalability, and smooth performance.
A Comprehensive Guide to Logging in Python
Python provides a built-in logging module in its standard library that provides comprehensive logging capabilities for Python programs
Load Testing with Locust: A High-Performance, Scalable Tool for Python
Learn how to perform high-concurrency load testing with Locust, an open-source Python framework. This step-by-step guide covers setting up Locust, creating test scenarios, analyzing performance metrics, and automating load tests to optimize API performance
Flask Error Handling Patterns
Learn how to handle errors in Flask effectively with custom error handlers, structured logging, and centralized monitoring. This guide covers best practices for managing exceptions, debugging issues, and improving application reliability with Flask
FastAPI Error Handling Patterns
Proper error handling is crucial for building secure, and maintainable APIs with FastAPI. Without thoughtful error management, web applications risk instability, unintended exposure of sensitive in...
Introduction to Mypy
Learn how to use Mypy, Python’s powerful static type checker, to improve code quality, catch type errors early, and enforce type safety. This comprehensive guide covers installation, configuration, advanced type hints, type narrowing, generics, and type coverage reports. Start writing cleaner, more reliable Python code today
Introduction to Pyright
Learn how to set up and use Pyright, a powerful and fast static type checker for Python. This guide covers installation, configuration, type checking modes, advanced type hints, and enforcing type safety with `Final` and `Literal`.
A Complete Guide to Gunicorn
Learn how to deploy and optimize Gunicorn for production with systemd and Nginx. This guide covers performance tuning, logging, security, and scaling strategies to make your Python web application fast, stable, and production-ready
Python Monitoring with Prometheus (Beginner's Guide)
This article provides a detailed guide on integrating Prometheus metrics into your Python application. It explores key concepts, including instrumenting your application with various metric types, ...
A Deep Dive into UV: The Fast Python Package Manager
uv is a next-generation package manager for Python that delivers exceptional speed and modern dependency management. It is designed to be a drop-in replacement for pip, pip-tools, and virtualenv, p...
A Complete Guide to Pydantic
Discover how to use Pydantic for data validation and serialization in Python. This guide covers defining models, enforcing constraints, creating custom validators, handling serialization, and generating JSON Schemas
Containerizing Django Applications with Docker
This article provides step-by-step instructions for deploying your Django applications using Docker and Docker Compose
Logging in Python: A Comparison of the Top 6 Libraries
There are many different logging libraries available for Python, each with its own strengths and weaknesses. Learn about the top 6 options in this article.
15 Common Errors in Python and How to Fix Them
Dealing with errors is a significant challenge for developers. This article looks at some of the most common Python errors and discusses how to fix them
Python Requests Throwing Sslerror
When Python's requests library throws an SSLError, it typically indicates that the SSL/TLS handshake failed when trying to establish a secure connection to a remote server. This can happen for seve...
A Gentle Introduction to Python's unittest Module
This article provides a comprehensive guide to writing, organizing, and executing unit tests in Python using the unittest module
A Beginner's Guide to Unit Testing with Pytest
Learn how to write clean, concise, and effective Python tests using Pytest's intuitive syntax, fixtures, parametrization, and rich plugin ecosystem
A Complete Guide to Pytest Fixtures
Learn how to use Pytest fixtures for writing maintainable and isolated tests.
How to Solve the ModuleNotFoundError With Pytest?
To fix the ModuleNotFoundError in pytest, you can start by making your tests directory a Python package.This can be achieved by including an empty __init__.py file to the directory: └── tests/ ...
How to Debug Pytest With pdb Breakpoints?
To debug a pytest test using pdb, you can manually insert a breakpoint by adding import pdb; pdb.set_trace() in your test: import pytest def divide(x, y): return x / y def testzerodivision(): ...
How to Disable a Test Using Pytest?
If you need to disable a specific test when running your test suite with pytest, use the pytest skip decorator. Suppose you have the following tests in your test suite: import pytest def test_addit...
How to Profile and Identify Slow Tests?
You can easily profile the duration of tests using pytest to identify slow tests using the --durations=N option. To display the execution time of every test function, set --durations to 0: pytest -...
How to Skip Directories With Pytest?
You can instruct Pytest to exclude specific directories from testing with the --ignore option. To exclude a single directory, execute: pytest --ignore=somedirectory To exclude multiple directories ...
How to Use Pytest With Virtualenv?
To effectively use Pytest within a Python virtual environment, follow these instructions: First, create a virtual environment using Python. Assuming you are using the current latest version, (Pytho...
How to Assert if an Exception Is Raised With Pytest?
Pytest can be used to test whether a function raises an exception. For instance, consider a division function that raises a ZeroDivisionError if there is an attempt to divide by zero: def divide(x,...
How to Test a Single File Under Pytest
To run a single test file with pytest, use the command pytest followed by the file path: pytest tests/test_file.py To execute a specific test within that file, append :: and the test name to the fi...
Get Started with Job Scheduling in Python
Learn how to create and monitor Python scheduled tasks in a production environment
A Comprehensive Guide to Python Logging with Structlog
Learn how to install, configure, and use the Struclog framework for logging in Python applications
10 Best Practices for Logging in Python
This article describes 10 best practices to follow when logging in Python applications to produce high quality logs that will help you keep your application running smoothly