Questions
Find answers to frequently asked development questions. For information about Better Stack products, explore our docs.
How to run cron jobs inside a docker container?
Running cron jobs inside a Docker container can be done by installing the cron daemon and scheduling the jobs in the container. Here are the steps to run cron jobs inside a Docker container: 🔠Want...
How can I add a volume to an existing Docker container?
You cannot directly add a volume to an existing Docker container, but you can create a new container with the same configuration as the existing container, but with an additional volume mounted. He...
How to get the IP address of the docker host from inside a docker container?
To get the IP address of the Docker host from inside a Docker container, you can use the docker.for.mac.localhost hostname. This hostname resolves to the IP address of the Docker host when used fro...
Will the Docker container automatically stop after "docker run -d"?
No, the Docker container will not automatically stop after running the docker run -d command. The -d flag tells Docker to run the container in "detached" mode, which means that it will run in the b...
How to rebuild docker container in docker-compose.yml?
To rebuild a Docker container specified in a docker-compose.yml file, you can use the docker-compose build command. Here are the steps to rebuild a container: Navigate to the directory containing t...
How to restart a single container with Docker Compose?
To restart a single container using Docker Compose, you can use the docker compose restart command, followed by the name of the service you want to restart. If you're using Compose standalone, use ...
Interactive shell using Docker Compose
To create an interactive shell using Docker Compose, you can specify the command to run in the container as an interactive shell. Here's an example docker-compose.yml file that launches an interact...
How do you attach and detach from Docker's process?
To attach to a running Docker container's process, you can use the docker attach command. This command attaches your terminal to the running process of the container, allowing you to view its outpu...
Copy directory to another directory using ADD command?
You can copy a directory to another directory using the ADD command in Docker. The basic syntax of the ADD command is: ADD source destination source: The path to the file or directory you want to c...
How to set image name in Dockerfile?
You can set the image name in a Dockerfile using the FROM instruction. The FROM instruction specifies the base image that your Docker image will be built upon. The basic syntax of the FROM instruct...
How to make Docker Compose wait for container X before starting Y?
You can make Docker Compose wait for a container X to be fully up and running before starting container Y by using the depends_on option in your docker-compose.yml file. The depends_on option allow...
How do I run a command on an already existing Docker container?
To run a command on an already existing Docker container, you can use the docker exec command. The docker exec command allows you to run a command in a running container. The basic syntax of the do...
How to use local docker images with Minikube?
To use local Docker images with Minikube, you can follow these steps: Start the Minikube cluster by running the following command: minikube start Build the Docker image: Build the Docker image usin...
How do I make a comment in a Dockerfile?
In a Dockerfile, you can add comments to provide information about the Dockerfile and the instructions in it. Comments in Dockerfiles start with the # symbol and continue to the end of the line. Fo...
How to fix Docker can't connect to daemon?
The error message "Cannot connect to the Docker daemon at unix:/var/run/docker.sock. Is the docker daemon running?" typically occurs when the Docker client is unable to connect to the Docker daemon...
What is the difference between RUN and CMD in a Dockerfile?
RUN and CMD are both instructions used in Dockerfiles, but they have different purposes. RUN instruction RUN is used to execute commands during the build process of a Docker image. These commands a...
What's the difference between Docker Compose vs. Dockerfile ?
Docker Compose and Dockerfile are two tools that are often used together in Docker-based application development, but they serve different purposes. Dockerfile Dockerfile is used to define the envi...
How to push a docker image to a private repository?
To push a Docker image to a private repository, you can follow these steps: Log in to the private repository using the following command: docker login <repository_url> Replace <repository_url> with...
How to upgrade the docker container after its image changed?
To upgrade a Docker container after its image has changed, you can follow these steps: Stop the container using the following command: docker stop <container_name_or_id> Replace <container_name_or_...
How to enter a Docker container already running with a new TTY?
To enter a Docker container already running with a new TTY, you can use the docker exec command. Here's an example: docker exec -it <container_id_or_name> sh Replace <container_id_or_name> with the...
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us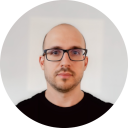
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github
Thank you to everyone who
Here is to all the fantastic people that are contributing and sharing their amazing projects: Thank you!