Convert bytes to a string in Python and vice versa?
To convert a string to bytes in Python, you can use the bytes
function. This function takes two arguments: the string to encode and the encoding to use. The default encoding is utf-8
. Here is an example:
s = 'hello'
b = bytes(s, 'utf-8')
print(b)
b'hello'
To convert bytes to a string in Python, you can use the decode
method of the bytes object. This method takes an encoding argument, which specifies the encoding of the bytes. Here is an example:
b = b'hello'
s = b.decode('utf-8')
print(s)
hello
-
Understanding slicing in Python?
Slicing is a way of extracting a specific part of an array. The syntax is following: mylist[start:end] # items start through end-1 mylist[start:] # items start through the rest of the array ...
Questions -
How to check if a string contains a substring in Python?
There are multiple ways to check if a string contains a specific substring. Using in keyword You can use in keyword to check if a string contains a specific substring. The expression will return bo...
Questions -
What is the difference between str and repr in Python?
In Python, str is used to represent a string in a more readable format, while repr is used to represent a string in an unambiguous and official format. The main difference between the two is that s...
Questions -
How to use the ternary conditional operator in Python?
The ternary conditional operator is a shortcut when writing simple conditional statements. If the condition is short and both true and false branches are short too, there is no need to use a multi-...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us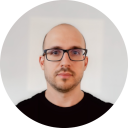
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github