Understanding slicing in Python?
Slicing is a way of extracting a specific part of an array.
The syntax is following:
my_list[start:end] # items start through end-1
my_list[start:] # items start through the rest of the array
my_list[:end] # items from the beginning through end-1
my_list[:] # a copy of the whole array
You can also use a step argument with all of the above:
my_list[start:end:step]
Start represents the first value that is present in the new array and stop represents the first value that is not present. Step sets how many values we move over when taking the next element. The default is 1
, meaning that we don’t skip any value from start
to end - 1
.
You can also use negative numbers. In that case, elements will be counted from end to start.
Here are some examples:
my_list = [1,2,3,4,5,6,7,8,9]
# second element from the end
print( my_list[-2] ) # 8
# two last elements
# note that if : is used the return type is array
print( my_list[-2:] ) # [8, 9]
# from the second element to the rest
print( my_list[2::1] ) # [3, 4, 5, 6, 7, 8, 9]
# from the start to the second element reversed (first three values)
print( my_list[2::-1] ) # [3, 2, 1]
# all except the last element reveresed
print( my_list[-2::-1] ) # [8, 7, 6, 5, 4, 3, 2, 1]
-
How can I safely create a nested directory in Python?
The most common way to safely create a nested directory in Python is using the pathlib or os modules. Using pathlib You can create a nested directory in python 3.5 or later using the Path and mkdir...
Questions -
How to access the index in for loops in Python?
In python, if you are enumerating over a list using the for loop, you can access the index of the current value by using enumerate function. my_list = [1,2,3,4,5,6,7,8,9,10] for index, value in enu...
Questions -
How to flatten a list in Python?
You can flatten a list in python using the following one-liner: flat_list = [item for sublist in l for item in sublist] In the example above, l is the list of lists that is to be flattened. The pre...
Questions -
How to use the ternary conditional operator in Python?
The ternary conditional operator is a shortcut when writing simple conditional statements. If the condition is short and both true and false branches are short too, there is no need to use a multi-...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us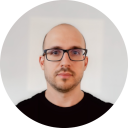
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github