What is the difference between str and repr in Python?
In Python, str
is used to represent a string in a more readable format, while repr
is used to represent a string in an unambiguous and official format. The main difference between the two is that str
is meant to be more user-friendly, while repr
is meant to be more machine-friendly.
For example, consider the following code:
s = 'Hello, world!'
print(str(s))
print(repr(s))
This will output the following:
Hello, world!
'Hello, world!'
As you can see, the output of str(s)
is more readable, while the output of repr(s)
includes quotes around the string to make it clear that it is a string.
In general, you should use str
when you want to display a string to the user, and repr
when you want to represent a string in a way that can be used to recreate the original object, such as in a debugger or when logging the state of an object.
-
What are metaclasses in Python?
In Python, a metaclass is the class of a class. It defines how a class behaves, including how it is created and how it manages its instances. A metaclass is defined by inheriting from the built-in ...
Questions -
How to use the ternary conditional operator in Python?
The ternary conditional operator is a shortcut when writing simple conditional statements. If the condition is short and both true and false branches are short too, there is no need to use a multi-...
Questions -
What Does the “yield” Keyword Do in Python?
To better understand what yield does, you need first to understand what generator and iterable are. What is iterable When you use a list or list-like structure, you can read the values from the lis...
Questions -
What Does if name == “main”: Do in Python?
The if __name__ == "main" is a guarding block that is used to contain the code that should only run went the file in which this block is defined is run as a script. What it means is that if you run...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us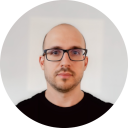
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github