How to use the ternary conditional operator in Python?
The ternary conditional operator is a shortcut when writing simple conditional statements. If the condition is short and both true and false branches are short too, there is no need to use a multi-line if statement.
If you come from a different language, you will notice that the python version of the ternary conditional operator is slightly different than in other languages. It looks like this
on_true() if condition else on_false()
In the example above, if the condition
is evaluated to be true, the on_true
function will be executed, otherwise, on_false
will be executed. Here is a practical example:
number = 5
print('Number is ' + ('even' if number % 2 == 0 else 'odd'))
Output:
Number is odd
As you can see, the ternary operator can be used on its own, or it can even be used as a part of the argument in the function call as seen in the example above. But there is more.
Selecting from a tuple or dictionary
We can shorten this even more. You can wrap the condition into []
brackets and drop the if
and else
keyword together. Then you can use a tuple for selecting the value based on the result of the condition in the brackets. The same can be done using a dictionary with the True
and False
keys.
Note the order in the tuple example.
number = 5
# using tuple
# also note the different order here
print('Number is ' + (('odd', 'even')[number % 2 == 0 ]))
# using dictionary
print('Number is ' + ({True: 'even', False: 'odd'}[number % 2 == 0 ]))
-
What Does the “yield” Keyword Do in Python?
To better understand what yield does, you need first to understand what generator and iterable are. What is iterable When you use a list or list-like structure, you can read the values from the lis...
Questions -
What Does if name == “main”: Do in Python?
The if __name__ == "main" is a guarding block that is used to contain the code that should only run went the file in which this block is defined is run as a script. What it means is that if you run...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us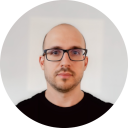
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github