How to check if a string contains a substring in Python?
There are multiple ways to check if a string contains a specific substring.
Using in keyword
You can use in keyword to check if a string contains a specific substring. The expression will return boolean value.
if 'Stack' in 'BetterStack':
print('Found it!')
else:
print('Did not found it!')
The general syntax is the following:
if substring in string:
# substring is in string
else
# substring is not in string
Using the String.index
You can also use String.index
method to find specific the index in the string where the substring starts.
string = 'BetterStack'
substring = 'Stack'
try:
string.index(substring)
except ValueError:
print('Not found!')
else:
print('Found it!')
Using the String.find
Also, you can use the find
function from the String class. This function will return -1
if the substring is not present in the string.
string = 'BetterStack'
substring = 'Stack'
if string.find(substring) != -1:
print('Found it!')
else:
print('Not found!')
-
Difference between static and class methods in Python?
Class method To create a class method, use the @classmethod decorator. Class methods receive the class as an implicit first argument, just like an instance method receives the instance. The class m...
Questions -
How to flatten a list in Python?
You can flatten a list in python using the following one-liner: flat_list = [item for sublist in l for item in sublist] In the example above, l is the list of lists that is to be flattened. The pre...
Questions -
Understanding slicing in Python?
Slicing is a way of extracting a specific part of an array. The syntax is following: mylist[start:end] # items start through end-1 mylist[start:] # items start through the rest of the array ...
Questions -
What Does the “yield” Keyword Do in Python?
To better understand what yield does, you need first to understand what generator and iterable are. What is iterable When you use a list or list-like structure, you can read the values from the lis...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us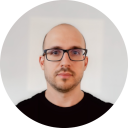
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github