JavaScript data validation has come a long way from manual checks and brittle conditionals. Today, you have powerful libraries at your fingertips to ensure the integrity of your data before it ever reaches the business logic.
Yup and TypeBox are two popular tools in this space. While both aim to solve the same core problem—validating and shaping data—they take fundamentally different approaches. Yup offers a fluent, chainable API that's great for runtime validation. TypeBox, on the other hand, embraces TypeScript deeply, generating schemas that serve both validation and static type safety. too.
Let's compare these libraries to help you pick the right one for your next project.
What is Yup?
Yup started as a validation library for React forms. Its creators wanted to make validation feel natural and readable for JavaScript developers.
With Yup, you write validation rules by chaining methods together methods like .required()
, .email()
, and .min(5)
create rules that are easy to understand even without TypeScript. This makes your code clear to read and maintain.
Yup began in the React ecosystem, and you can use it anywhere. It works with Vue, Angular, or any JavaScript application. Though not built specifically for TypeScript, it offers good support through type definitions.
What is TypeBox?
TypeBox connects TypeScript types with JSON Schema validation. It bridges what TypeScript checks during development and what your program validates when it runs.
When you use TypeBox, you write schemas once and get both TypeScript types and runtime validation. The syntax looks similar to how you'd write TypeScript types, which makes it feel familiar to TypeScript developers.
Even if you don't use TypeScript, TypeBox gives you solid validation based on JSON Schema standards. Its error messages follow JSON Schema patterns, making them useful for both debugging and user feedback.
Yup vs. TypeBox: a quick comparison
Your choice between Yup and TypeBox will shape how you handle validation throughout your project. Here's a simple breakdown of their key differences:
Feature | Yup | TypeBox |
---|---|---|
Main approach | Chain-based API with simple methods | JSON Schema with TypeScript type generation |
TypeScript support | Added later through type definitions | Built from the ground up for TypeScript |
How you write schemas | Chain methods like .email().required() |
Structure that mirrors TypeScript syntax |
Error handling | Detailed errors with paths and messages | JSON Schema standard error objects |
Learning curve | Easy for beginners with clear method names | Steeper if you don't know JSON Schema |
Custom validators | Simple with the .test() method |
Uses JSON Schema keywords and extensions |
Performance | Good for most web applications | Fast due to JSON Schema compilation |
Bundle size | Medium with good tree-shaking | Smaller core size |
Framework support | Works great with React ecosystem | Works with any framework, special tRPC support |
Schema definition
The way you write validation rules ripples through your entire project, from type safety and developer experience to runtime performance.
Yup uses a method-chaining style that feels natural to JavaScript developers. You build rules step by step, adding constraints like this:
import * as yup from 'yup';
const userSchema = yup.object({
name: yup.string().required().min(2),
email: yup.string().email().required(),
age: yup.number().positive().integer().min(18)
});
// Validate the data
try {
const user = userSchema.validateSync(formData);
saveUser(user);
} catch (error) {
showErrors(error.errors);
}
Yup's approach is JavaScript-first. While it works with TypeScript, you typically define your types separately or use Yup's InferType
utility.
TypeBox takes a completely different approach. It uses a structure that mirrors TypeScript's own type system:
import { Type } from '@sinclair/typebox'
import { TypeCompiler } from '@sinclair/typebox/compiler'
const UserSchema = Type.Object({
name: Type.String({ minLength: 2 }),
email: Type.String({ format: 'email' }),
age: Type.Number({ minimum: 18, integer: true })
})
// Create a validator from the schema
const validator = TypeCompiler.Compile(UserSchema)
// Validate the data
if (validator.Check(formData)) {
saveUser(formData);
} else {
showErrors(validator.Errors(formData));
}
TypeBox generates both TypeScript types and validation rules from one definition. This keeps everything in sync and eliminates duplication.
The key difference is their focus. Yup aims for readability and an intuitive API. TypeBox prioritizes TypeScript integration and JSON Schema compatibility.
Validation patterns
Validation isn’t just a background task—it’s deeply tied to how your application handles errors, structures its logic, and maintains consistency. Whether you're working on form inputs, API responses, or internal data flows, the way validation runs can shape the architecture around it.
Yup gives you several ways to validate data. You can validate synchronously or with promises, throw errors or return results:
// Sync validation with error throwing
try {
const data = schema.validateSync(input);
// Use valid data
} catch (error) {
// Handle validation errors
}
// Promise-based validation
schema.validate(input)
.then(data => {
// Use valid data
})
.catch(error => {
// Handle validation errors
});
// Just check if valid
if (schema.isValidSync(input)) {
// Data is valid
}
Yup also offers options like abortEarly
and stripUnknown
to control validation behavior. This flexibility lets you handle validation errors in the way that best fits your app.
TypeBox follows JSON Schema patterns. You first compile your schema for performance, then run validation without throwing errors:
// Compile the schema once
const validator = TypeCompiler.Compile(schema)
// Check if data is valid
if (validator.Check(input)) {
// Use valid data
} else {
// Get detailed errors
const errors = validator.Errors(input)
handleErrors(errors)
}
TypeBox's approach separates schema definition from validation logic. This clean separation can make your code more maintainable, especially in larger projects.
The main difference is that Yup combines validation and error handling in one step, while TypeBox separates these concerns. Both work well, but they fit different coding styles.
Handling complex validation scenarios
Real apps need more than basic field validation. You often need conditionals, dependencies between fields, or custom business rules. Both libraries can handle these complex needs.
Yup shines with its built-in methods for complex cases. Here's how you might validate a shipping form with conditional rules:
const shippingSchema = yup.object({
method: yup.string().oneOf(['standard', 'express', 'pickup']),
// Address only required for delivery options
address: yup.string().when('method', {
is: val => val !== 'pickup',
then: schema => schema.required('Address needed for delivery')
}),
// Store only required for pickup
storeId: yup.string().when('method', {
is: 'pickup',
then: schema => schema.required('Store required for pickup')
})
});
Yup's .when()
method makes conditional validation simple. For custom rules, you can use the .test()
method to write any validation logic you need.
TypeBox handles complex validation through JSON Schema patterns. It might require more setup but offers powerful type integration:
import { Type } from '@sinclair/typebox'
// Define separate schemas for different methods
const DeliverySchema = Type.Object({
method: Type.Union([
Type.Literal('standard'),
Type.Literal('express')
]),
address: Type.String(),
storeId: Type.Optional(Type.String())
})
const PickupSchema = Type.Object({
method: Type.Literal('pickup'),
storeId: Type.String(),
address: Type.Optional(Type.String())
})
// Combine them with a discriminated union
const ShippingSchema = Type.Union([DeliverySchema, PickupSchema])
TypeBox excels with TypeScript's type system features like discriminated unions and intersection types. For business logic that doesn't fit in schemas, you can add custom validation after the schema check.
For async validation (like checking if a username is taken), Yup has built-in support:
const schema = yup.object({
username: yup.string().test(
'username-available',
'Username already taken',
async value => {
const available = await checkUsername(value);
return available;
}
)
});
TypeBox requires a bit more setup for async validation, typically using ajv's async features alongside TypeBox schemas.
The main difference: Yup provides an all-in-one API for complex validation scenarios, while TypeBox leverages TypeScript's type system and JSON Schema patterns for structural validation, with custom logic added separately.
Type safety and integration
For TypeScript projects, how a validation library works with the type system is important. Yup and TypeBox take completely different approaches to TypeScript integration.
Yup was built for JavaScript first, with TypeScript support added later. You typically define your schemas and types separately:
// Define schema for validation
const userSchema = yup.object({
id: yup.number().integer().positive(),
name: yup.string(),
email: yup.string().email()
});
// Define type for TypeScript
interface User {
id: number;
name: string;
email: string;
}
// You need to connect them manually
function processUser(data: unknown): User {
return userSchema.validateSync(data) as User;
}
Yup does offer InferType
to generate types from schemas, but there can be subtle mismatches between what Yup validates and what TypeScript expects.
TypeBox was built for TypeScript from day one. It generates both validation rules and TypeScript types from the same definition:
// Define schema once
const UserSchema = Type.Object({
id: Type.Number({ integer: true, minimum: 1 }),
name: Type.String(),
email: Type.String({ format: 'email' })
})
// Get type automatically
type User = typeof UserSchema.static
// No need for type assertions
function processUser(data: unknown): User {
const validator = TypeCompiler.Compile(UserSchema)
if (validator.Check(data)) {
return data // TypeScript knows this is valid
}
throw new Error('Invalid data')
}
With TypeBox, validation and typing stay perfectly in sync. When validation passes, TypeScript automatically knows the data matches the expected type.
This key difference affects how you work:
- In JavaScript projects, both work well and type integration doesn't matter.
- In mixed JavaScript/TypeScript codebases, Yup lets you add types gradually.
- In TypeScript-focused projects, TypeBox eliminates duplication and keeps types and validation in sync.
Your choice depends on how important TypeScript is to your project. Yup works well for teams who treat validation and typing as separate concerns. TypeBox is ideal for teams who want perfect alignment between runtime validation and compile-time type checking.
Ecosystem and maturity
A validation library exists within a broader ecosystem of tools and community support. The surrounding environment affects how productive you'll be.
Yup has been around since 2014 and has strong connections to the React world. It's widely used in production applications and has extensive documentation and examples. Most React form libraries like Formik and React Hook Form have official Yup adapters.
Using Yup with React forms is straightforward:
import { useFormik } from 'formik';
import * as yup from 'yup';
const schema = yup.object({
name: yup.string().required('Name is required'),
email: yup.string().email('Invalid email').required('Email is required')
});
function SignupForm() {
const formik = useFormik({
initialValues: { name: '', email: '' },
validationSchema: schema,
onSubmit: values => submitForm(values)
});
return (
<form onSubmit={formik.handleSubmit}>
{/* Form fields */}
</form>
);
}
TypeBox is newer (created in 2020) but has quickly gained traction in the TypeScript ecosystem. It works well with modern frameworks like tRPC and Fastify. Its deep integration with JSON Schema also connects it to tools like OpenAPI and ajv.
TypeBox works especially well in full-stack TypeScript applications:
import { Type } from '@sinclair/typebox'
import { initTRPC } from '@trpc/server'
// Define schema for API input
const UserInput = Type.Object({
name: Type.String(),
email: Type.String({ format: 'email' })
})
// Create tRPC router with TypeBox validation
const t = initTRPC.create()
const appRouter = t.router({
createUser: t.procedure
.input(UserInput)
.mutation(async ({ input }) => {
// Input is fully typed and validated
return saveUser(input);
})
});
The ecosystems reflect different strengths. Yup is ideal for React applications, especially form-heavy interfaces. It has years of refinement and widespread adoption. TypeBox excels in TypeScript projects, particularly full-stack applications where sharing types between client and server is important.
Performance considerations
Validation happens often in web apps - on every form submission, API request, and data update. While performance is rarely the bottleneck, understanding how each library handles it can help with high-traffic applications.
Yup focuses on developer experience first, with reasonable performance for most cases. It offers options to improve speed when needed:
// Performance optimizations
const schema = yup.object({
// Schema definition
}).strict() // Fail on extra properties
.noUnknown() // No unknown properties
// Stop at first error for faster validation
const result = schema.validateSync(data, { abortEarly: true });
Yup's bundle size is moderate (about 20kb minified and gzipped) with good tree-shaking support to reduce size further.
TypeBox takes advantage of JSON Schema compilation for performance. When you compile a schema, TypeBox generates optimized validation code:
// Compile once for best performance
const validator = TypeCompiler.Compile(schema)
// Fast validation without try/catch overhead
if (validator.Check(data)) {
// Valid data
}
TypeBox's core is smaller than Yup, especially if you only use the features you need. It works well with code splitting and tree-shaking.
For most applications, both libraries are fast enough. Yup might be slightly slower with complex, deeply nested objects, while TypeBox can handle these more efficiently due to its compilation step. But unless you're validating thousands of objects per second, the difference is minimal.
The real performance consideration is developer productivity - which library helps your team work faster and create fewer bugs. This often matters more than raw validation speed.
Final thoughts
Both Yup and TypeBox are solid choices for data validation—but they’re built for different workflows.
Go with Yup if you want a simple, chainable API, great error messages, and tight integration with React forms. It’s battle-tested and widely adopted.
Choose TypeBox if your project is TypeScript-heavy and you want seamless type safety without duplication. It pairs perfectly with full-stack TypeScript and JSON Schema tooling.
For most modern TypeScript projects, TypeBox offers a cleaner, more type-safe experience. But if you're already deep into Yup or React, sticking with what works is totally fine.
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us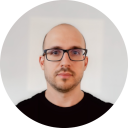
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github