When testing JavaScript, two tools often come up: Jest, the long-time favorite, and Vitest, the fast-growing alternative. Both help you write reliable tests, but they take different approaches.
Vitest is built on top of Vite and focuses on speed. It starts quickly, runs fast, and fits well with modern front-end development.
Jest, created by Facebook, has been a popular choice for years. It includes everything you need for testing, like mocking, coverage reports, and snapshot testing, with little setup required.
This guide compares them so you can choose the one that works best for your project.
What is Jest?
Jest is the most used JavaScript testing tool, giving you everything in one package.
Facebook made Jest in 2014, combining a test runner with assertion tools, mocking features, and code coverage in a straightforward setup.
Unlike other testing tools that need many plugins, Jest works immediately. It tests across different environments, runs tests in parallel, and includes a helpful watch mode that updates as you code.
What is Vitest?
Vitest is built for modern JavaScript, using Vite's super-fast bundling system. It feels familiar to Jest users but fully supports ESM, TypeScript, and instant updates, making it perfect for Vue, React, and Svelte projects.
Vitest focuses on developer experience with minimal setup while delivering impressive speed. Since it's part of the Vite ecosystem, testing feels consistent with how you develop, improving both speed and workflow.
Vitest vs. Jest: quick comparison
Feature | Vitest | Jest |
---|---|---|
Main focus | Speed with Jest compatibility | Reliability with complete features |
Setup difficulty | Minimal, uses your Vite config | Simple, with good defaults |
Speed | Very fast with instant updates | Good speed with watch mode |
Module support | Native modern JavaScript with legacy support | Legacy-first with limited modern support |
Framework fit | Perfect for Vite projects (Vue, React, Svelte) | Works with any JavaScript project |
Snapshot testing | Supported, works like Jest | Built-in with excellent tools |
Mocking | Compatible with Jest, plus Vite features | Complete built-in mocking |
TypeScript | Native, no extra setup | Needs additional configuration |
Watch mode | Ultra-fast updates from Vite | Interactive and stable updates |
Ecosystem | Growing quickly, fewer plugins | Large, mature ecosystem |
Test UI | Modern browser-based interface | Terminal-based display |
Writing tests
How you write tests affects your productivity and test quality.
Jest provides ready-to-use functions without imports. Its mature ecosystem includes extensive guides and patterns:
// Jest example
test('displays username', () => {
render(<UserProfile username="testuser" />);
expect(screen.getByText('testuser')).toBeInTheDocument();
});
Jest's functions are available automatically. The testing flow is straightforward, with built-in mocking. This makes writing tests simple but does clutter the global scope.
Vitest lets you use the same style or import functions directly:
// Vitest example
import { test, expect, vi } from 'vitest';
test('handles empty data', () => {
const consoleSpy = vi.spyOn(console, 'error');
render(<UserProfile />);
expect(consoleSpy).not.toHaveBeenCalled();
});
Vitest gives you options between global functions and explicit imports. It uses vi
instead of jest
for mocking but works similarly while running faster.
Mocking
Good tests often need to fake parts of your code, and both frameworks handle this differently.
Jest includes powerful mocking tools for faking modules, spying on functions, and controlling timers:
// Jest mocking
jest.mock('node-fetch');
global.fetch = jest.fn(() => Promise.resolve({
json: () => Promise.resolve({ id: 1 })
}));
Jest's mocking is powerful but sometimes tricky to understand with its hoisting system.
Vitest offers similar mocking features with better performance and modern JavaScript support:
// Vitest mocking
vi.mock('node-fetch');
global.fetch = vi.fn(() => Promise.resolve({
json: () => Promise.resolve({ id: 1 })
}));
Vitest's mocking looks familiar to Jest users but works better with modern JavaScript modules and runs faster.
Speed
Test speed matters for your workflow and continuous integration.
Jest prioritizes reliability over raw speed. It creates separate environments for tests and uses Node.js processes for isolation:
# Jest output
PASS src/components/UserProfile.test.js
Time: 3.45 s
Jest performs well, especially with parallelization and watch mode, but setting up modules and environments can slow down larger projects.
Vitest takes a completely different approach using Vite's dev server and hot reloading:
# Vitest output
✓ src/components/UserProfile.test.js (3 tests) 42ms
Duration: 135ms
Vitest with Vite runs dramatically faster, especially in watch mode. Tests use hot reloading, meaning only changed code and affected tests run again, not entire test files.
Independent tests show Vitest running 10-20x faster than Jest for the same tests in watch mode, especially with TypeScript and modern JavaScript.
Snapshot testing
Snapshot testing captures component output to detect unwanted changes. Both frameworks handle snapshots differently.
Jest created snapshot testing as a core feature, making it easy to capture and check component output:
// Jest snapshot
test('Button renders correctly', () => {
const tree = renderer.create(<Button label="Submit" />).toJSON();
expect(tree).toMatchSnapshot();
});
Jest creates snapshot files next to test files, making them easy to track. Snapshots store as readable text for easy review.
Vitest works similarly to Jest but adds improvements for modern components:
// Vitest snapshot
test('Button label', () => {
const { getByRole } = render(<Button label="Submit" />);
expect(getByRole('button').textContent).toMatchInlineSnapshot(`"Submit"`);
});
Vitest's snapshot system works like Jest's but compares snapshots faster thanks to Vite integration. It also handles component libraries with dynamic classes better.
Framework integration
Testing tools need to work well with modern JavaScript libraries. Jest and Vitest handle integration differently.
Jest needs configuration for each framework, especially modern ones. This approach is mature but takes setup:
// Jest config
module.exports = {
testEnvironment: 'jsdom',
transform: {
'^.+\\.(js|jsx)$': 'babel-jest',
},
};
Jest requires manual setup for each project, often with specific presets for different frameworks.
Vitest inherits settings from your Vite setup, making framework integration mostly automatic:
// Vitest config
export default defineConfig({
plugins: [vue()],
test: {
environment: 'jsdom',
globals: true,
},
});
Vitest's integration is smoother because it uses the same plugins and transformers your app uses during development. This reduces setup work and ensures tests run in an environment like production.
Debugging tests
Good debugging helps maintain test quality and fix failures quickly.
Jest offers several debugging options, from watch mode to Node.js debuggers:
// Jest debugging
test('debug test', () => {
console.log({ result });
expect(result).toEqual(expected);
});
Jest includes detailed error messages for failed tests, though Node.js debugging takes extra setup.
Vitest improves debugging with a browser interface and better error reporting:
// Vitest debugging
test.only('focus on this test', () => {
console.log({ result });
expect(result).toEqual(expected);
});
Vitest's browser UI gives you a significant advantage for debugging, with live test results, error navigation, and console output in an interface that feels natural to web developers.
Final thoughts
Vitest is a great choice if you're using modern tools like Vite. It's fast and works well with new JavaScript features. Jest has been around longer and is known for being reliable with lots of plugins and community support.
Use Vitest if you want speed and are building with tools like Vue, React (with Vite), or Svelte. Use Jest if you want something more established that works with almost any JavaScript project.
Both are solid tools. Choose the one that best fits your project, team, and what matters most to you—speed, support, or compatibility.
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us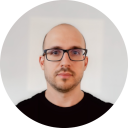
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github