When building TypeScript applications that connect to databases, you'll likely reach for an ORM (Object-Relational Mapper) to simplify your data access code. TypeORM and MikroORM stand out as two powerful options, each with distinct approaches to database interaction.
TypeORM dominates the TypeScript landscape today with its flexible approach to data access. It allows you to choose between different coding styles, works with numerous databases, and provides an extensive ecosystem of plugins and integrations.
MikroORM takes a more opinionated path, focusing on data integrity and clean architecture. Its automatic change tracking and explicit transaction handling help maintain consistency in complex applications.
This comparison will help you understand their key differences and decide which one fits your next project best.
What is TypeORM?
TypeORM became the most popular TypeScript ORM after its release in 2016. It took good ideas from other ORMs like Hibernate and Entity Framework and brought them to TypeScript.
What makes TypeORM special is how it lets you choose how to work with your data. You can either add save/update methods directly to your data classes (Active Record style) or keep that logic separate (Data Mapper style). This flexibility makes TypeORM feel familiar whether you came from Ruby on Rails or Java. TypeORM connects to various databases and integrates seamlessly with Express, NestJS, and other popular frameworks.
What is MikroORM?
MikroORM came along in 2018 with some fresh ideas about how TypeScript ORMs should work. It focuses on keeping your code organized following software design principles that many larger companies use.
The big difference with MikroORM is how it handles saving data. Instead of updating your database immediately when you make a change, it keeps track of all your changes and sends them to the database all at once when you're ready. This approach helps prevent data errors and often makes your app faster. MikroORM also leverages TypeScript's type system to catch more errors before your code even executes.
TypeORM vs. MikroORM: a quick comparison
Your choice between these ORMs affects how you'll write code and structure your application. Each was built with different goals in mind, making them better fits for different projects.
Here's how they compare on key features:
Feature | TypeORM | MikroORM |
---|---|---|
Core pattern | Active Record and Data Mapper | Unit of Work and Identity Map |
Entity definition | Class-based with decorators | Class-based with decorators |
Change tracking | Manual with save calls | Automatic via Identity Map |
Transaction management | Both explicit and implicit | Explicit via Unit of Work |
Query building | QueryBuilder and find methods | QueryBuilder and EntityRepository |
Performance optimization | Eager and lazy relations | Explicit loading with collections |
TypeScript integration | Good type safety | Excellent type safety with generics |
Relationship handling | Cascades and lazy loading | Collection semantics and proxies |
Migration support | Built-in CLI | Integration with Umzug |
Raw query support | Extensive | Good with QueryBuilder |
Documentation quality | Comprehensive but fragmented | Well-structured and consistent |
Learning curve | Gentle for basic use | Steeper due to DDD concepts |
Community size | Large, mature ecosystem | Smaller but growing quickly |
Framework integration | Many integrations available | Official NestJS, Express packages |
Database support | Extensive (10+ databases) | Good (MySQL, PostgreSQL, SQLite, MongoDB) |
Model definition
The foundation of any ORM lies in how you define your database models. Both TypeORM and MikroORM use TypeScript decorators, but their philosophies differ significantly.
TypeORM lets you choose between two popular ways to work with your data. You can use the Active Record pattern where your models save themselves:
// Active Record style
@Entity()
export class User extends BaseEntity {
@PrimaryGeneratedColumn()
id: number;
@Column({ length: 50, unique: true })
username: string;
// Method that saves itself
async setUsername(name: string) {
this.username = name;
return this.save();
}
}
// Using it
const user = new User();
user.username = "john";
await user.save();
Or you can use the Data Mapper pattern with separate repositories:
@Entity()
export class User {
@PrimaryGeneratedColumn()
id: number;
@Column({ length: 50, unique: true })
username: string;
}
// Using a repository
const userRepository = dataSource.getRepository(User);
const user = new User();
user.username = "john";
await userRepository.save(user);
TypeORM's approach feels familiar if you've used other ORMs, providing flexibility without forcing a particular coding style.
MikroORM takes a more deliberate approach focused on clean code organization:
@Entity()
export class User {
@PrimaryKey()
id: number;
@Property({ length: 50 })
@Unique()
username: string;
// Just change the data - no saving yet
setUsername(name: string) {
this.username = name;
// MikroORM tracks this change automatically
}
}
// Working with an EntityManager
const em = orm.em.fork(); // Creates a separate workspace
const user = new User();
user.username = "john";
em.persist(user); // Mark for saving later
// Save all changes at once
await em.flush();
MikroORM introduces concepts like EntityManager and fork() that create isolated workspaces for your changes. This requires more learning but delivers automatic change tracking and better transaction handling.
Query building
Database queries reveal the most striking differences between these ORMs. Your choice affects both code readability and performance.
TypeORM gives you several ways to query your data. For complex queries, you can use the QueryBuilder with a chain of methods:
// Building a complex query step by step
const usersWithPosts = await dataSource
.getRepository(User)
.createQueryBuilder("user")
.leftJoinAndSelect("user.posts", "post")
.where("user.isActive = :active", { active: true })
.getMany();
// Simple queries with find methods
const activeUsers = await userRepository.find({
where: { isActive: true },
take: 10
});
TypeORM makes queries feel natural, from simple lookups to complex reports. You can start with basic find methods and move to QueryBuilder when you need more power.
MikroORM takes a more organized approach to queries by putting them in custom repository classes:
// Define a custom repository with your query methods
@Repository(User)
export class UserRepository extends EntityRepository<User> {
// Custom query method
findActiveWithRecentPosts(date: Date): Promise<User[]> {
return this.createQueryBuilder("user")
.leftJoinAndSelect("user.posts", "post")
.where({ isActive: true })
.getResult();
}
}
// Using the repository
const userRepository = em.getRepository(User);
const usersWithRecentPosts = await userRepository.findActiveWithRecentPosts(
new Date("2023-01-01")
);
MikroORM encourages you to organize query logic in repository classes. This requires more initial setup but keeps your code cleaner as your project grows.
Transaction management
Reliable transaction handling forms the cornerstone of data integrity in any application. Each ORM offers different approaches to this crucial functionality.
TypeORM gives you multiple ways to handle transactions:
// Detailed transaction control
const queryRunner = dataSource.createQueryRunner();
await queryRunner.connect();
await queryRunner.startTransaction();
try {
const user = new User();
user.username = "john";
await queryRunner.manager.save(user);
// More operations...
await queryRunner.commitTransaction();
} catch (err) {
await queryRunner.rollbackTransaction();
throw err;
}
// Or use a decorator for cleaner code
@Transaction()
async createUserWithProfile(
@TransactionManager() manager: EntityManager,
userData: UserDto
) {
const user = new User();
user.username = userData.username;
await manager.save(user);
// More operations...
return user;
}
TypeORM's flexibility lets you choose between detailed control or convenience, though you need to understand how each approach works.
MikroORM builds its whole design around explicit transactions with its Unit of Work pattern:
// Unit of Work pattern
const em = orm.em.fork();
try {
await em.begin();
const user = new User();
user.username = "john";
em.persist(user);
// Send all changes to database at once
await em.commit();
} catch (err) {
await em.rollback();
throw err;
}
// Or use this helper for simpler code
await em.transactional(async (em) => {
const user = new User();
user.username = "john";
em.persist(user);
// Commits automatically at the end
});
MikroORM encourages thinking in complete transactions rather than individual operations. The fork() method creates a separate workspace so changes in one transaction don't affect others.
Relationship handling
Managing connections between data entities profoundly influences application architecture and performance. The ORMs tackle this challenge with distinct strategies.
TypeORM lets you define relationships with decorators and control how they load:
@Entity()
export class User {
// Other fields...
@OneToMany(() => Post, post => post.author, {
cascade: true, // Saves related posts when user is saved
eager: false // Don't load automatically
})
posts: Post[];
}
@Entity()
export class Post {
// Other fields...
@ManyToOne(() => User, user => user.posts)
author: User;
}
// Different ways to load relationships
const user = await userRepository.findOneBy({ id: 1 });
const posts = await user.posts; // Makes a separate query
// Eager loading - gets everything at once
const userWithPosts = await userRepository.findOne({
where: { id: 1 },
relations: ["posts"]
});
TypeORM provides familiar relationship patterns with options like eager loading, lazy loading, and cascading saves/deletes. This flexibility enables both quick development and performance tuning.
MikroORM uses a different approach with Collections, giving you more explicit control:
@Entity()
export class User {
// Other fields...
@OneToMany(() => Post, post => post.author)
posts = new Collection<Post>(this);
}
@Entity()
export class Post {
// Other fields...
@ManyToOne()
author: User;
}
// Working with collections
const user = await em.findOne(User, { id: 1 });
// Nothing loaded yet
console.log(user.posts.isInitialized()); // false
// Load posts when you need them
await user.posts.init();
console.log(user.posts.length); // Now loaded
// Adding related records
const post = new Post();
post.title = "MikroORM guide";
user.posts.add(post);
MikroORM's Collection approach provides clear control over when data loads. This prevents the common N+1 query problem (where you accidentally make too many database queries), but requires more deliberate coding.
Migration support
Synchronizing database schema changes with your evolving codebase presents a critical challenge for any application. Both ORMs offer specialized tools to address this need.
TypeORM comes with a built-in command-line tool for creating and running migrations:
// TypeORM migration file
export class CreateUserTable1617293465123 implements MigrationInterface {
async up(queryRunner: QueryRunner): Promise<void> {
await queryRunner.query(`
CREATE TABLE "user" (
"id" SERIAL PRIMARY KEY,
"username" varchar(50) NOT NULL UNIQUE
)
`);
}
async down(queryRunner: QueryRunner): Promise<void> {
await queryRunner.query(`DROP TABLE "user"`);
}
}
Using the TypeORM CLI is straightforward:
# Create a migration based on your entity changes
typeorm migration:generate -n CreateUserTable
# Apply migrations to your database
typeorm migration:run
TypeORM simplifies keeping your database in sync with your code. The automatic migration generation proves especially valuable during rapid development cycles.
MikroORM uses the Umzug library for its migration system:
// MikroORM migration file
export class CreateUserTable extends Migration {
async up(): Promise<void> {
this.addSql(`
CREATE TABLE "user" (
"id" SERIAL PRIMARY KEY,
"username" varchar(50) NOT NULL UNIQUE
)
`);
}
async down(): Promise<void> {
this.addSql(`DROP TABLE "user"`);
}
}
MikroORM's command-line tools work similarly:
# Create a migration from schema changes
npx mikro-orm migration:create --name CreateUserTable
# Apply migrations
npx mikro-orm migration:up
MikroORM's migration system integrates seamlessly with its overall design. The migrations work consistently across different database systems thanks to MikroORM's database abstraction layer.
TypeScript integration
Type safety represents a major advantage of using TypeScript-first ORMs. While both libraries offer strong typing features, their implementations differ significantly.
TypeORM provides solid TypeScript integration that helps catch many common errors:
// Repository methods are properly typed
const userRepository = dataSource.getRepository(User);
const user = await userRepository.findOneBy({ id: 1 });
// user is typed as User | null
// Relationship types are enforced
const post = new Post();
post.title = "Hello TypeORM";
post.author = 123; // Error: Type 'number' is not assignable to type 'User'
TypeORM ensures you use the correct entity types and properties throughout your code. Occasionally, though, it loses type safety with more complex queries.
MikroORM elevates TypeScript integration to another level with extensive generics and strict typing:
// Fully typed repositories
const userRepository = em.getRepository(User);
const user = await userRepository.findOne({ id: 1 });
// Precisely typed as User | null
// Custom repositories with typed methods
@Repository(User)
export class UserRepository extends EntityRepository<User> {
findByEmail(email: string): Promise<User | null> {
return this.findOne({ email });
}
}
MikroORM fully embraces TypeScript's advanced features to deliver excellent type safety throughout your application. This strict typing catches errors during development rather than in production, proving especially valuable for larger projects.
Final thoughts
TypeORM is a good choice if you want something flexible and easy to learn. It supports many databases and works well for quick development.
MikroORM is better if you need more structure, strong TypeScript support, and reliable data handling in complex apps.
Both are solid options. Select the option that best suits your project and team.
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us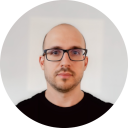
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github