Best Practices for Securing Node.js Applications in Production
Node.js is a widely adopted JavaScript runtime environment crucial for server-side development. It is supported by npm's extensive ecosystem of third-party packages, which sees millions of daily downloads.
However, this popularity also attracts cybercriminals. Attackers often exploit the trust developers place in these packages by injecting malicious code into dependencies, a tactic known as a supply chain attack. These attacks can propagate broadly, impacting multiple projects.
In addition to package vulnerabilities, attackers directly target applications, exploiting security flaws to gain unauthorized access. Common attacks include Cross-Site Scripting (XSS), injection attacks, and Man-in-the-Middle (MITM) attacks, which can manipulate databases, steal data, and compromise web applications. To mitigate these risks, adhering to best practices is essential to safeguard your applications.
This article will explore various best practices for securing Node.js applications in production.
Why you should secure Node.js applications?
As you build your applications, you'll rely heavily on npm packages. However, npm's popularity also makes it a target for attacks, which can impact your projects. For instance, in 2022, attackers exploited stolen OAuth tokens to exfiltrate private npm repositories, gaining access to sensitive data. Additionally, npm has faced other security issues, including account takeovers and malicious code injection into popular packages like ua-parser-js
and rc
.
Even without npm vulnerabilities, your application is still exposed to threats. Database interactions can lead to SQL injection, user inputs can cause cross-site scripting (XSS), and external API calls may result in data breaches. Inadequate error handling, flawed authentication or authorization, insecure configurations, and improper session management can also compromise your application's security.
Addressing these vulnerabilities requires a comprehensive approach, especially as such attacks are increasingly common. An IBM Security report from 2022 noted a 33% rise in incidents due to vulnerability exploitation between 2020 and 2021.
The following sections will explore best practices for securing Node.js applications in production.
1. Implement security headers
Implementing security headers is crucial to ensure the security of your application. Without these headers, your site is vulnerable to various attacks, such as:
- Cross-Site Scripting (XSS): Attackers inject malicious code to steal user data or hijack sessions.
- Clickjacking: Tricks users into clicking on hidden elements to perform unintended actions.
- Content Injection: Allows unauthorized modifications of website content.
To mitigate these risks, you should add security headers to your application. A popular option is to use the Helmet library with frameworks like Fastify or Express. Helmet will automatically add the necessary security headers.
For Express, you can use Helmet like this:
import helmet from "helmet";
app.use(helmet());
For Fastify, use the fastify-helmet plugin:
import helmet from "@fastify/helmet";
fastify.register(
helmet,
{ contentSecurityPolicy: false } // Example: disable the `contentSecurityPolicy` middleware
);
By default, this will add necessary security headers to your application. For instance, the Strict-Transport-Security
header instructs browsers to prefer HTTPS, ensuring encrypted communications. The Cross-Origin-Resource-Policy
header blocks other sites from accessing your resources, protecting against cross-origin attacks. For a detailed list of the security headers the package adds, refer to the Helmet documentation.
2. Regularly update Node.js and dependencies
Maintaining a secure environment requires regularly updating Node.js and your dependencies. Npm has faced vulnerabilities, and maintainers actively patch these to ensure security. Keeping everything up-to-date helps protect your application from known issues, ensuring you benefit from the latest patches and improvements.
One efficient way to update Node.js is to use nvm. Here’s how to install and switch to a new version:
nvm install <version>
nvm use <version>
Automating this process in your CI/CD pipeline ensures consistent environments across development and production.
To ensure the packages you use are secure, leverage tools like npm-audit
or Snyk.
The npm-audit
command analyzes your dependency tree against the GitHub Advisory Database to identify known vulnerabilities and suggests remedies:
npm audit
If vulnerabilities are detected, you will be informed of the necessary actions:
23 vulnerabilities (5 moderate, 18 high)
To address all issues, run:
npm audit fix
Running npm audit fix
will install compatible updates for vulnerable dependencies, ensuring they are secure and up-to-date.
For a more comprehensive analysis, consider third-party tools like Snyk
, which provides deeper insights into potential vulnerabilities:
npx snyk test
npx snyk wizard
Regularly updating your packages to their latest versions is also crucial. The npm-check-updates
tool can help you identify and update packages with new versions:
npx npm-check-updates
To update the package.json
file with the latest versions, add the -u
option:
npx npm-check-updates -u
When updating, constantly review changelogs for major updates to understand potential impacts. It's wise to test updates in a staging environment before applying them to production. Having a rollback strategy is also crucial in case updates cause unexpected issues.
4. Protect sensitive information
When your application has authentication functionality, sensitive information like emails and passwords can be exposed to attackers, especially if stored in plain text in the database. Additionally, attackers can exploit the time it takes for your application to respond to requests, such as when comparing passwords. By measuring response times, they can guess the length and value of passwords through repeated requests.
Moreover, it can be a significant security risk if your application code contains hardcoded secrets like database names, passwords, or secret keys from different services (such as AWS). If your source code is compromised, the secrets will be exposed. Hardcoding secrets make it difficult to rotate or update them without modifying and redeploying the code, increasing the risk of unauthorized access.
To avoid these security lapses: Implement strong authentication mechanisms in your Node.js application using libraries like Passport.js or OAuth. Consider multi-factor authentication (MFA) to reduce the risk of unauthorized access. Enforce strict requirements when creating passwords to ensure that users create strong passwords.These requirements should include a minimum length of at least 12 characters and a combination of uppercase and lowercase letters, numbers, and special characters.
In addition, never store passwords or other sensitive data in plain text. Instead, use strong, one-way hashing algorithms from packages like bcrypt
or Argon2. These algorithms are designed to be computationally intensive, making brute-force attacks impractical.
Here’s how to use bcrypt
in Node.js to hash passwords:
import bcrypt from "bcrypt";
const saltRounds = 10;
const hashedPassword = await bcrypt.hash(password, saltRounds);
Be aware of timing attacks, in which attackers exploit response time differences to guess password lengths or values. Use constant-time comparison functions when checking passwords or other sensitive values.
The built-in crypto
library provides the timingSafeEqual
method for comparison:
import crypto from "crypto";
const safeCompare = (a, b) =>
crypto.timingSafeEqual(Buffer.from(a), Buffer.from(b));
Now that you know how to protect sensitive data using strong authentication and encryption, let's examine error handling and logging as the next security practice.
5. Error handling and logging
In production, your application, databases, or servers can generate errors. If not correctly handled, users might see error messages revealing critical details about your system, such as stack traces, mismatched inputs, or network timeouts. Poor error handling can expose your application to attackers who may learn about the versions of dependencies or frameworks your application uses, which could have known vulnerabilities.
Additionally, attackers may gain insights into your APIs or other system components, allowing them to bypass security measures if exceptions are not properly restricted. For instance, an attacker might bypass the login process if your website only checks for correct credentials without handling incorrect inputs or unexpected errors.
Moreover, inadequate logging can make it difficult to detect and diagnose issues. Logs are essential for noticing suspicious activity or tracing the steps of an attack. Without logs, you may not be aware that an attack has occurred or struggle to understand how it happened.
To address these concerns, thoroughly test your application by sending unexpected data or pushing it to edge cases. Implement the try/catch
construct for error handling:
try {
// Code that may throw an error
} catch (error) {
// Handle the error
}
For asynchronous operations, ensure you handle promises properly. For asynchronous methods with EventEmitters, route errors to the error
event:
emitter.on("error", (err) => {
console.error("An error occurred:", err);
});
For better insights, add logging using a tool like Pino, which can capture significant events. Examples include:
- Input validation failures.
- Authentication attempts, particularly unsuccessful ones.
- Access control failures.
- Any apparent tampering events, including unexpected changes to state data.
- Attempts to connect with invalid or expired session tokens.
Ensure that error messages are generic and do not include sensitive data, such as passwords or personal information. A centralized logging system like Better Stack can manage logs, preventing attackers from removing traces of their actions.
6. Protect your application from DoS attacks
Protecting your Node.js application from Denial of Service (DoS) attacks is essential to ensure its security. It requires a comprehensive approach that addresses various attack vectors and implements multiple layers of defense. DoS attacks come in diverse forms, including network-layer assaults like SYN floods, application-layer attacks like HTTP floods, and resource exhaustion techniques targeting CPU, memory, or disk space.
One of the key strategies in safeguarding your application is implementing rate limiting. This can be done using packages like rate-limiter-flexible for complex scenarios or express-rate-limit for simpler use cases in Node.js applications. Additionally, consider implementing rate limiting at the infrastructure level using services like Nginx, cloud load balancers, or API gateways for more robust protection.
Restricting the size of incoming request payloads is another vital strategy to prevent attackers from overwhelming your server with large data volumes. This can be configured at the firewall level or using built-in middleware in web frameworks:
app.use(express.json({limit: '2mb'}));
app.use(express.urlencoded({limit: '2mb', extended: false}));
To protect against memory-based attacks, use Node.js's --secure-heap=n
option to allocate a separate heap for storing sensitive data. Beyond these measures, implementing strict input validation, intelligent caching mechanisms, and leveraging Content Delivery Networks (CDNs) can also significantly enhance your application's resilience to DoS attacks.
Additional Security Practices
So far, you've seen some of the major security best practices. Several additional practices can further enhance your project's security:
Use Security-Related Linter Plugins: Incorporating linter plugins like eslint-plugin-security can significantly enhance your code’s security. This plugin helps catch vulnerabilities early in the development process by detecting issues such as unsafe Regular Expressions, improper input validation, and the risky use of
eval()
. For even greater effectiveness, consider integrating these linting rules with git hooks to enforce security checks before code is committed, ensuring your codebase remains secure throughout the development lifecycle.Import Built-in Modules Using the 'node:' Protocol: When importing built-in Node.js modules, always use the
node:
protocol, like this:import { methodName } from "node:module";
This practice protects against typosquatting attacks, where malicious actors may try to trick you into using a compromised package by mimicking the name of a legitimate one. By explicitly specifying the
node:
protocol, you ensure that only official Node.js modules are imported, reducing the risk of inadvertently including harmful third-party packages.Use Non-Root Users in Docker Containers: In Docker environments, running containers with root privileges by default is common, posing a security risk. To mitigate this, create a non-root user and run your application under this user. For example:
# Dockerfile example ... EXPOSE 3000 USER node ...
Additionally, apply the same principle to your servers by ensuring that only non-root users have active roles. This limits the impact of potential security breaches, as the non-root user would have restricted permissions.
Prevent SQL Injection with Parameterized Queries: SQL injection is a common attack vector that can be mitigated by using parameterized queries, which safely escape user inputs. To further reduce the risk of SQL injection, consider using Object-Relational Mappers (ORMs) like Sequelize, Knex, TypeORM, or Objection.js. These ORMs provide built-in mechanisms to safely interact with databases, abstracting away raw SQL queries and automatically applying the necessary protections against SQL injection. You can also use sqlmap to detect memory vulnerabilities.
Final thoughts
This guide outlines some of the best security practices for ensuring your Node.js applications can run safely and without vulnerabilities in production.
To continue learning how to secure applications, see the Node.js security practices in the Node.js documentation. Also, check out the OWASP Top 10 for comprehensive insights into common security threats.
Thanks for reading, and happy coding!
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us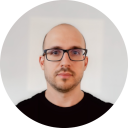
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github