CSV (Comma-Separated Values) remains a widely used data format, even alongside newer options like JSON and XML. Its strength lies in its simplicity: a plain-text, table-like structure that works with virtually every spreadsheet application and data tool.
Papa Parse stands out in the Node.js ecosystem as a CSV parsing library that originated in the browser before being adapted for server-side use. This unique heritage gives it cross-environment compatibility that pure Node.js CSV libraries lack.
This guide walks through Papa Parse's core features in Node.js, from reading and transforming CSV files to converting data between formats using both in-memory and streaming approaches.
Prerequisites
You'll need Node.js 16 or later installed to use the ES module syntax and modern file system APIs.
Familiarity with JavaScript's asynchronous patterns and array manipulation methods will help you extract maximum value from Papa Parse.
Getting started with Papa Parse in Node.js
Papa Parse was initially made for use in web browsers, and it takes a different approach to handling CSV files. Unlike most Node.js libraries that focus on streaming data for servers, Papa Parse aims to be easy to use and work the same way on both the front end and back end. This makes it especially useful if you’re building apps that share code between the browser and the server.
As shown in the diagram below, Papa Parse processing follows a straightforward flow, converting raw CSV into JavaScript objects you can manipulate with standard methods:
This architecture makes Papa Parse particularly well-suited for microservices, serverless functions, and small to medium-sized data processing tasks where code clarity trumps raw performance.
Let's set up a project to explore Papa Parse:
mkdir csv-node && cd csv-node
Next, initialize your project:
npm init -y
Install Papa Parse from npm:
npm install papaparse
Set up your project to use ES modules instead of CommonJS. Papa Parse supports both, but ES modules offer cleaner and more modern syntax:
npm pkg set type="module"
Now, you're ready to start working with CSV files.
Reading CSV files with Papa Parse
Papa Parse's primary function parse()
provides a deceptively simple interface that handles many CSV complexities automatically. Unlike Node.js stream-based parsers that require event handling, Papa Parse's synchronous API returns a complete results object immediately.
Create a sample CSV file named employees.csv
with this data:
id,name,department,salary
1,John Smith,Engineering,75000
2,Sara Johnson,Marketing,82000
3,Michael Brown,Finance,95000
Now create an app.js
file with this code:
import { readFile } from 'fs/promises';
import Papa from 'papaparse';
async function parseCSV() {
// Read the file content
const fileContent = await readFile('employees.csv', 'utf8');
// Parse CSV string to objects
const result = Papa.parse(fileContent, {
header: true, // First row contains headers
skipEmptyLines: true, // Skip blank lines
dynamicTyping: true // Convert numbers and booleans
});
// Print the headers
console.log(`Headers: ${result.meta.fields}`);
// Print each row
result.data.forEach(row => {
console.log(`Employee: ${row.name} works in ${row.department} earning $${row.salary}`);
});
}
parseCSV().catch(console.error);
This approach differs from traditional Node.js CSV parsers. Instead of setting up streams and handling chunks as they arrive, Papa Parse processes the entire file at once. The result
object provides three key properties:
data
: Array of parsed rows (as objects when usingheader: true
)meta
: File metadata including field names, delimiters detected, etc.errors
: Any parsing errors encountered
Run the script to see Papa Parse in action:
node app.js
Headers: id,name,department,salary
Employee: John Smith works in Engineering earning $75000
Employee: Sara Johnson works in Marketing earning $82000
Employee: Michael Brown works in Finance earning $95000
Notice how Papa Parse automatically converted numeric values like 75000
to JavaScript numbers instead of strings. This happens because of the dynamicTyping: true
option. Unlike many other CSV parsers that return all values as strings, Papa Parse can detect and convert numbers automatically.
Working with CSV data as JavaScript objects
One of the great things about Papa Parse is how well it works with common JavaScript data handling methods. If you parse a CSV using the header: true
option, you'll get an array of plain JavaScript objects. That means you can easily use functions like map()
, filter()
, and reduce()
to work with the data.
Now let’s update app.js
to show how this works:
import { readFile } from 'fs/promises';
import Papa from 'papaparse';
async function parseCSV() {
// Read and parse in one function
const fileContent = await readFile('employees.csv', 'utf8');
const { data, meta } = Papa.parse(fileContent, {
header: true,
skipEmptyLines: true,
dynamicTyping: true
});
// Calculate total salary
const totalSalary = data.reduce((sum, employee) => sum + employee.salary, 0);
const averageSalary = totalSalary / data.length;
// Find high earners
const highEarners = data.filter(employee => employee.salary > 80000);
// Print summary data
console.log(`Total employees: ${data.length}`);
console.log(`Average salary: $${averageSalary.toFixed(2)}`);
console.log(`Number of high earners: ${highEarners.length}`);
// List high earners
console.log("\nHigh earners:");
highEarners.forEach(employee => {
console.log(`- ${employee.name} ($${employee.salary})`);
});
}
parseCSV().catch(console.error);
In this highlighted code, you're using Papa Parse to return structured data as plain JavaScript objects.
With dynamicTyping: true
enabled, values like salary
are automatically parsed as numbers. This lets you use array methods like reduce()
to calculate totals and averages, and filter()
to find high earners without manually converting strings to numbers.
The totalSalary
calculation works as expected because employee.salary
is already a number.
Run the updated code:
node app.js
Total employees: 3
Average salary: $84000.00
Number of high earners: 2
High earners:
- Sara Johnson ($82000)
- Michael Brown ($95000)
This pattern of parsing once and then using standard JavaScript array methods is the recommended approach for using Papa Parse. It sets the library apart from stream-based parsers, which are built around processing one row at a time as the file is read.
Streaming large CSV files
While Papa Parse processes typically the entire file in one go, it also supports a streaming mode built for Node.js. This allows you to handle large files more efficiently while maintaining a simple API. It’s a useful middle ground between Papa Parse’s ease of use and the streaming model that Node.js is known for.
Create a new file named stream.js
and add the following code:
import fs from "node:fs";
import Papa from "papaparse";
// Create a readable stream from the CSV file
const fileStream = fs.createReadStream("employees.csv");
// Set up Papa Parse stream processor
const parseStream = Papa.parse(Papa.NODE_STREAM_INPUT, {
header: true,
dynamicTyping: true,
});
// Process data as it comes in
parseStream.on("data", (row) => {
// Each row is already an object with properties from headers
console.log(`Employee: ${row.name} works in ${row.department} earning $${row.salary}`);
// You can perform any processing on each row here
if (row.salary > 80000) {
console.log(`High earner found: ${row.name} ($${row.salary})`);
}
});
// Track processing events
parseStream.on("finish", () => {
console.log("Parsing finished");
});
// Connect the file stream to the Papa Parse stream
fileStream.pipe(parseStream);
// Add file stream event handlers
fileStream.on("open", () => console.log("File opened"));
fileStream.on("end", () => console.log("File reading completed"));
This approach uses standard Node.js stream event listeners to handle the parsed data, unlike the browser-based usage of Papa Parse. Here's what happens step by step:
- A file stream is created with
fs.createReadStream()
- A Papa Parse stream processor is set up using
Papa.parse(Papa.NODE_STREAM_INPUT, {...})
- A
data
event listener is added to process each parsed row - A
finish
event listener is used to detect when parsing is complete - The file stream is piped into the Papa Parse processor using
fileStream.pipe(parseStream)
Run the script to see how streaming works in practice:
.
command
node stream.js
File opened
Got chunk: { id: 1, name: 'John Smith', department: 'Engineering', salary: 75000 }
Got chunk: { id: 2, name: 'Sara Johnson', department: 'Marketing', salary: 82000 }
Got chunk: { id: 3, name: 'Michael Brown', department: 'Finance', salary: 95000 }
File data event
File ended
Parse stream finished
This streaming approach maintains low memory usage, regardless of the file size. It gives you immediate access to chunks of data as they’re parsed, so you can start working with them right away. Your application stays responsive, and you don’t have to wait for the entire file to load before beginning processing.
With small files like the one in this example, the benefits aren't obvious. But when you're working with files that are several megabytes or even gigabytes in size, streaming is essential to avoid memory issues and keep performance steady.
Converting CSV to JSON
In addition to parsing CSV into JavaScript objects in memory, Papa Parse also supports streaming conversion—allowing you to transform CSV data into JSON as it is read. This is especially useful when dealing with large files that would be inefficient to load all at once.
Create a new file named export.js
and add the following code:
import fs from 'node:fs';
import Papa from 'papaparse';
// Create read and write streams
const input = fs.createReadStream('employees.csv');
const output = fs.createWriteStream('employees.json');
// Track whether we're writing the first row
let isFirstRow = true;
// Write the opening bracket for the JSON array
output.write('[\n');
// Set up Papa Parse stream processor
const parseStream = Papa.parse(Papa.NODE_STREAM_INPUT, {
header: true,
dynamicTyping: true,
skipEmptyLines: true,
});
// Handle each parsed row
parseStream.on('data', (row) => {
const json = JSON.stringify(row, null, 2);
const prefix = isFirstRow ? '' : ',\n';
output.write(prefix + json);
isFirstRow = false;
});
// Handle stream completion
parseStream.on('finish', () => {
// Write the closing bracket of the JSON array
output.write('\n]\n');
output.end();
console.log('CSV successfully converted to JSON using streaming.');
});
// Pipe input CSV stream into Papa Parse
input.pipe(parseStream);
// Optional: track file stream lifecycle
input.on('open', () => console.log('File opened for reading'));
input.on('end', () => console.log('CSV reading completed'));
This script converts a CSV file into a JSON array without loading everything into memory:
- The file is read line by line using a stream.
- Each row is parsed and converted into a JSON object.
- The JSON objects are written incrementally into a new file, wrapped in brackets to form a valid array.
Run the script
node export.js
File opened for reading
CSV reading completed
CSV successfully converted to JSON using streaming.
After running the script, you'll find a file named employees.json
with content like:
[
{
"id": 1,
"name": "John Smith",
"department": "Engineering",
"salary": 75000
},
{
"id": 2,
"name": "Sara Johnson",
"department": "Marketing",
"salary": 82000
},
{
"id": 3,
"name": "Michael Brown",
"department": "Finance",
"salary": 95000
}
]
This approach allows you to convert large CSV files to JSON without consuming excess memory. It’s well-suited for background jobs, ETL pipelines, or any scenario where performance and scalability matter.
Final thoughts
Papa Parse provides a flexible and consistent approach to working with CSV data in Node.js, whether you're handling small files in memory or streaming large datasets efficiently.
Its support for both parsing and exporting, combined with built-in type conversion and cross-environment compatibility, makes it a practical tool for modern data processing tasks.
To explore more advanced features such as custom delimiters, encoding options, and web worker support, refer to the official documentation: https://www.papaparse.com/docs.
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us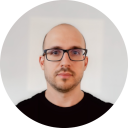
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github