Parcel vs Vite: Choosing the Right Frontend Build Tool
Two popular tools for building frontend applications are Parcel and Vite. Both help you bundle and serve JavaScript apps, but they work differently.
Parcel focuses on simplicity with zero configuration. You can start using it immediately without setting up config files, making it approachable for developers at any skill level.
Vite prioritizes speed through native ES modules. Created by Evan You (who made Vue.js), Vite serves source files directly during development and only bundles for production.
This article compares both tools to help you choose the right one for your projects.
What is Parcel?
Parcel is a bundler that works without requiring configuration. It simplifies frontend development by handling all the complex tooling decisions for you.
Released in 2017 as an alternative to configuration-heavy tools like Webpack, Parcel looks at your assets and automatically figures out what to include in your bundle. It detects dependencies and applies the right transformations without you needing to specify them.
Parcel makes development faster with hot module replacement, and it automatically discovers configuration files for preprocessors like Sass or TypeScript. It handles JavaScript, CSS, HTML, images, and many other file types right out of the box - no extra setup needed.
What is Vite?
Vite is a modern build tool designed for speed. Unlike traditional bundlers, Vite uses native ES modules in the browser to make development incredibly fast.
Though it started as a Vue.js tool, Vite now works with any JavaScript framework. It solves the problem of slow startup times in modern frontend development by splitting how it handles development and production. During development, it serves individual files directly for instant updates. For production, it uses Rollup to create optimized bundles.
Vite supports TypeScript, JSX, CSS preprocessors, and more with minimal setup. It specifically targets issues like slow server starts, sluggish updates, and complex build processes that can slow down work on large applications.
Parcel vs Vite: a quick comparison
Your choice between these tools affects how you'll develop, build, and deploy your projects. Each has different strengths that make them suitable for different situations.
Here's a comparison of their key features:
Feature | Parcel | Vite |
---|---|---|
Philosophy | Zero-configuration bundling | Native ESM-powered development |
Development server speed | Fast with caching | Extremely fast with no bundling in dev |
Configuration required | Minimal to none | Minimal, but more explicit |
Production build tool | Custom bundler | Rollup |
Hot Module Replacement | Automatic for supported file types | Precise and extremely fast |
Framework integration | Framework-agnostic | First-class support for many frameworks |
TypeScript support | Built-in | Built-in |
CSS preprocessor support | Automatic detection | Requires minimal configuration |
Asset handling | Automatic processing | Manual imports, but optimized |
Plugin ecosystem | Growing, but smaller | Extensive and growing rapidly |
Learning curve | Very gentle | Gentle, steeper for customization |
Ideal project size | Small to medium projects | Small to large-scale applications |
Community and ecosystem | Solid community | Fast-growing, strong framework integration |
Caching mechanism | File-based caching | Browser native caching + in-memory |
Configuration file | package.json or .parcelrc | vite.config.js |
Installation and setup
How you start with a build tool sets the tone for your development experience. Parcel and Vite have different approaches to getting started.
Parcel truly delivers on its zero-configuration promise. You just install it and point it to your entry file:
# Install Parcel
npm install --save-dev parcel
# Add start script to package.json
# "scripts": {
# "start": "parcel index.html",
# "build": "parcel build index.html"
# }
# Start the development server
npm start
With just these commands, Parcel automatically finds your project's dependencies and frameworks. It discovers and uses your existing configurations for Babel, PostCSS, or TypeScript if you have them, or it applies defaults if you don't:
<!-- index.html -->
<!DOCTYPE html>
<html>
<head>
<title>Parcel Example</title>
<link rel="stylesheet" href="./styles.scss">
</head>
<body>
<div id="app"></div>
<script src="./index.js"></script>
</body>
</html>
Parcel will process the SCSS file and bundle the JavaScript without you adding any configuration. This reduces mental overhead, especially if you're new to build tools.
Vite also aims for simplicity but takes a more explicit approach:
# Create a new project with Vite
npm create vite@latest my-project
cd my-project
# Follow the prompts to select a template
# (e.g., vanilla, vue, react, preact, lit, svelte)
# Install dependencies
npm install
# Start development server
npm run dev
Vite offers templates for popular frameworks, giving you a well-structured starting point:
import { defineConfig } from 'vite'
import react from '@vitejs/plugin-react'
export default defineConfig({
plugins: [react()],
server: {
open: true,
},
})
While Vite needs a config file for customization, the starter templates give you sensible defaults. The configuration is minimal but clear, so you can see how your project is processed.
Project configuration
How you set up your build process affects how easy it is to maintain and change. Parcel and Vite take very different approaches to configuration.
Parcel tries to eliminate configuration entirely. When you do need to configure it, you have a few options:
{
"source": "src/index.html",
"browserslist": "> 0.5%, last 2 versions, not dead",
"targets": {
"default": {
"distDir": "dist",
"sourceMap": true
}
}
}
For more complex needs, you can use a .parcelrc
file:
{
"extends": "@parcel/config-default",
"transformers": {
"*.{ts,tsx}": ["@parcel/transformer-typescript-tsc"]
},
"optimizers": {
"*.js": ["@parcel/optimizer-terser"]
}
}
Parcel's configuration focuses on overriding defaults rather than specifying everything. This reduces boilerplate but sometimes makes it harder to understand what's happening behind the scenes.
Vite uses a JavaScript configuration file that gives you direct control over the build process:
import { defineConfig } from 'vite'
import vue from '@vitejs/plugin-vue'
import legacy from '@vitejs/plugin-legacy'
export default defineConfig({
plugins: [
vue(),
legacy({
targets: ['> 1%, last 2 versions, not dead']
})
],
css: {
preprocessorOptions: {
scss: {
additionalData: `@import "./src/styles/variables.scss";`
}
}
},
build: {
rollupOptions: {
output: {
manualChunks: {
vendor: ['vue', 'vue-router']
}
}
}
}
})
Vite's approach is more explicit and programmatic. You can use conditional logic, environment-specific settings, and directly access Rollup's configuration for production builds.
This explicit approach makes understanding and adjusting the build process easier, though you need to know more about the available options. Vite balances sensible defaults with control when you need it.
Development server and hot reloading
A fast development server with hot module replacement (HMR) is essential for productive work. Both tools excel here but use different methods.
Parcel includes a development server with automatic HMR:
# Start Parcel dev server
npx parcel src/index.html
Once running, Parcel watches for file changes and rebuilds the affected modules. For most file types, it can replace the changed modules without reloading the page:
import { createApp } from './app'
const app = createApp()
document.getElementById('app').appendChild(app)
// HMR interface
if (module.hot) {
module.hot.accept('./app', function() {
console.log('App updated')
document.getElementById('app').removeChild(app)
const newApp = createApp()
document.getElementById('app').appendChild(newApp)
})
}
While Parcel handles most HMR setup automatically, you can customize the behavior for specific modules as shown above. The development server also includes automatic HTTPS, caching for faster rebuilds, and custom middleware support.
Vite takes a completely different approach. Instead of bundling during development, it serves files directly to the browser using native ES modules:
# Start Vite dev server
npx vite
This approach skips the bundling step during development, making server startup and updates extremely fast:
import { createApp } from 'vue'
import App from './App.vue'
import './style.css'
createApp(App).mount('#app')
// HMR is handled automatically for Vue files
// For custom handling:
if (import.meta.hot) {
import.meta.hot.accept('./some-module.js', (newModule) => {
// Custom HMR handling
})
}
Vite's HMR is incredibly precise and fast, often updating in less than 50ms. It works exceptionally well with Vue, React, Svelte, and other frameworks without additional configuration.
Vite's development server also pre-bundles dependencies (for node_modules), splits CSS code, and optimizes assets. The ESM-based approach requires a modern browser during development, but the production build supports older browsers through the legacy plugin.
Building for production
The production build process becomes critical for performance and browser compatibility when preparing your app for deployment.
Parcel's production builds start with the build command:
# Create production build
npx parcel build src/index.html
Parcel applies several optimizations automatically:
// Example of code Parcel will automatically optimize
import { someFunction } from './utils'
import unusedModule from './unused'
// Tree-shaking will remove unusedModule
export function main() {
return someFunction()
}
Parcel's production builds include minification, tree-shaking, and code splitting. It automatically handles CSS minification, JavaScript compression, and image optimization. You can also target different browsers:
{
"targets": {
"modern": {
"engines": {
"browsers": "Chrome >= 80"
}
},
"legacy": {
"engines": {
"browsers": "> 0.5%, last 2 versions, not dead"
}
}
}
}
This lets you create multiple versions of your code for different browser targets, optimizing for both modern and legacy browsers.
Vite uses Rollup for its production builds, a mature and highly configurable bundler:
# Create production build
npx vite build
Vite's production builds leverage Rollup's powerful optimization features:
export default defineConfig({
build: {
minify: 'terser',
cssCodeSplit: true,
rollupOptions: {
output: {
manualChunks: (id) => {
if (id.includes('node_modules')) {
return 'vendor'
}
}
}
}
}
})
With Vite, you get fine control over how your application is bundled for production. It supports advanced features like:
- Dynamic import for code splitting
- CSS code splitting to match JavaScript chunks
- Efficient asset handling with hashing and base64 inlining for small files
- Customizable chunk strategies through Rollup's options
- Pre-rendering for static sites
Vite's use of Rollup gives you access to Rollup's plugin ecosystem, allowing for specialized optimizations based on your project's needs.
Asset handling and preprocessing
Modern web apps include diverse assets like styles, images, fonts, and more. How a build tool handles these assets affects both development experience and production optimization.
Parcel automatically processes and transforms many asset types without requiring configuration:
<!-- HTML referencing various assets -->
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" href="./styles.scss">
<link rel="icon" href="./favicon.png">
</head>
<body>
<img src="./images/logo.svg">
<script src="./index.ts"></script>
</body>
</html>
Parcel finds these references, applies the right transformations, and bundles them together. It handles:
- CSS preprocessors like SCSS, Less, and Stylus
- TypeScript and modern JavaScript features
- Image optimization and SVG transformation
- JSON, YAML, and other data formats
- Web Workers and Service Workers
Parcel follows references from HTML, CSS, and JavaScript, including only what's needed. This also enables automatic code splitting at dynamic import points:
// Parcel handles dynamic imports for code splitting
import('./heavy-module').then(module => {
module.doSomething()
})
Vite also supports various asset types but takes a more explicit approach, especially for non-JavaScript assets:
// Explicit imports in Vite
import styles from './style.css' // CSS modules
import sassStyles from './style.scss' // requires sass plugin
import logoUrl from './logo.svg' // returns a URL
import logoComponent from './logo.svg?component' // as Vue component
import workers from './worker?worker' // Web Worker
Vite's handling of assets is more explicit, giving you control over how assets are processed. This approach requires more deliberate importing but makes it clearer what's happening.
For CSS, Vite provides built-in processing features:
export default defineConfig({
css: {
modules: {
// Configure CSS modules
localsConvention: 'camelCase'
},
preprocessorOptions: {
scss: {
// Add global SCSS variables
additionalData: `$primary: #ff0000;`
}
}
}
})
Vite also has specialized handling for different asset types:
- Static assets under
/public
are served and copied as-is - Small imported assets are inlined as base64 URLs
- SVGs can be imported as URLs or components
- WebAssembly, Web Workers, and other specialized formats have dedicated import patterns
Framework integration
Many frontend projects use frameworks like React, Vue, or Svelte. How well a build tool integrates with these frameworks significantly impacts your development experience.
Parcel works with any framework out of the box, detecting and applying appropriate transformations based on file extensions and dependencies:
// React component (works without configuration)
import React from 'react'
import './Button.css'
export function Button({ children, onClick }) {
return (
<button className="button" onClick={onClick}>
{children}
</button>
)
}
Parcel automatically detects React based on JSX and the React dependency, applying the necessary transformations. The same applies to Vue (.vue files) or Svelte (.svelte files).
This zero-config approach extends to framework-specific features:
- React Fast Refresh for improved HMR
- Vue single-file components
- Svelte component compilation
While Parcel's automatic detection is convenient, it sometimes lacks framework-specific optimizations that more specialized tools provide.
Vite takes a more explicit approach to framework integration through its plugin system:
import { defineConfig } from 'vite'
import react from '@vitejs/plugin-react'
export default defineConfig({
plugins: [react()]
})
Vite offers official plugins for major frameworks:
- @vitejs/plugin-react for React with Fast Refresh
- @vitejs/plugin-vue for Vue with SFC support
- @vitejs/plugin-svelte for Svelte components
This explicit approach allows for deeper framework integration and optimization. For example, the Vue plugin in Vite provides:
<!-- Vue component with HMR -->
<template>
<div class="counter">
<button @click="increment">Count: {{ count }}</button>
</div>
</template>
<script>
import { ref } from 'vue'
export default {
setup() {
const count = ref(0)
const increment = () => count.value++
return { count, increment }
}
}
</script>
<style scoped>
.counter {
/* Scoped CSS is processed efficiently */
}
</style>
Vite's Vue integration is particularly strong, given its origin as a Vue-specific tool. However, its React, Svelte, and other framework integrations have quickly caught up, providing optimized development experiences for each.
Vite also offers starter templates for various frameworks, setting up projects with best practices:
# Create a new React project with Vite
npm create vite@latest my-react-app -- --template react
This deep framework integration makes Vite especially appealing for projects using modern JavaScript frameworks.
Practical workflows
The daily usage patterns of these build tools show how they fit into different development workflows.
Parcel excels in projects where simplicity and minimal configuration are priorities:
# Simple Parcel workflow
mkdir new-project && cd new-project
npm init -y
npm install --save-dev parcel
npm install react react-dom
# Create basic files
echo '<div id="app"></div><script src="./index.js"></script>' > index.html
# Create React entry point
cat > index.js << EOF
import React from 'react';
import { createRoot } from 'react-dom/client';
const App = () => <h1>Hello Parcel</h1>;
const root = createRoot(document.getElementById('app'));
root.render(<App />);
EOF
# Add script to package.json
# "scripts": { "start": "parcel index.html" }
# Start development
npm start
This workflow takes you from an empty directory to a running React application in minutes, with no configuration files needed. Parcel handles all the transformation and bundling automatically.
Parcel works best for:
- Quick prototyping and small projects
- Teams with varying levels of build tool experience
- Projects where you want to focus on code rather than configuration
- Static sites with simple asset requirements
- Modernizing legacy codebases where zero-config is valuable
Vite shines in projects that benefit from its speed and more explicit configuration approach:
# Modern Vite workflow
npm create vite@latest my-app
cd my-app
npm install
# Customize configuration
# Edit vite.config.js
# Start development
npm run dev
# For production
npm run build
npm run preview
Vite's templates provide well-structured starting points for various frameworks, making it easy to start with best practices in place. Its development server is exceptionally fast, even for large applications, thanks to the ESM-based approach.
Vite works best for:
- Medium to large-scale applications
- Projects using modern JavaScript frameworks
- Teams that value development speed
- Applications with complex build requirements
- Projects that need precise control over the build process
- Progressive Web Apps and other modern web applications
For more complex setups, Vite's explicit configuration provides clarity:
// Typical Vite setup for a React project with TypeScript
import { defineConfig } from 'vite'
import react from '@vitejs/plugin-react'
import { resolve } from 'path'
export default defineConfig({
plugins: [react()],
resolve: {
alias: {
'@': resolve(__dirname, './src')
}
},
server: {
proxy: {
'/api': {
target: 'http://localhost:3000',
changeOrigin: true
}
}
},
build: {
outDir: 'dist',
rollupOptions: {
output: {
manualChunks: {
vendor: ['react', 'react-dom'],
utils: ['lodash', 'date-fns']
}
}
}
}
})
This configuration sets up path aliases, development server proxying, and custom chunk strategies for the production build—all in a single, clear file.
Final thoughts
Parcel and Vite offer different frontend approaches. Parcel is a zero-configuration bundler, ideal for quickly starting projects and prototypes without worrying about tooling.
Vite, using native ES modules and Rollup for production, offers fast development and better control for complex projects. For simple, small to medium projects, Parcel is best, while Vite shines in larger, modern framework-based applications.
Choose Parcel for simplicity or Vite for speed and control. Both tools maximize performance with minimal configuration.
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us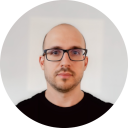
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github