Node.js development often requires working with multiple Node versions across different projects. While Node Version Manager (NVM) is a popular solution, several alternatives provide different approaches to version management, each with their own strengths and limitations.
This article explores these alternatives to help you find the right tool for your development workflow.
Why Node.js version management matters
Managing multiple Node.js versions has become an essential part of modern JavaScript development. Different projects might require specific Node versions for compatibility with dependencies, frameworks, or runtime features. Some legacy projects may need to stay on older, stable Node releases, while new projects might leverage cutting-edge features available only in the latest versions.
Without a version manager, developers face several challenges:
- Uninstalling and reinstalling Node.js repeatedly
- Managing global npm packages across versions
- Ensuring consistent Node.js environments across development teams
- Troubleshooting version-specific issues
Let's examine the top alternatives to NVM and how they address these challenges.
N: The minimalist alternative
N is a simple Node.js version manager created by TJ Holowaychuk, the original creator of Express.js. It focuses on simplicity and speed, making it one of the lightest alternatives to NVM.
Installation
Installing N is straightforward using npm:
npm install -g n
Or via Homebrew on macOS:
brew install n
Basic usage
N's command structure is intentionally minimal:
# Install specific version
n 14.17.0
# Install latest LTS release
n lts
# Install latest stable release
n latest
# Switch between installed versions
n
# Use specific version temporarily in a new shell
n use 16.13.0 some-script.js
Advantages of N
N offers several benefits that make it popular among developers:
- Simplicity: The minimal API is easy to learn and use
- Speed: Faster operation than some other version managers
- No shell modifications: Doesn't require shell script integrations
- Works with npm: Can be installed via npm itself
Configuration example
You can configure N's installation directory by setting the N_PREFIX environment variable:
export N_PREFIX=$HOME/.n
export PATH=$N_PREFIX/bin:$PATH
FNM: Fast Node Manager
Fast Node Manager (FNM) is a relatively new alternative built with performance in mind. Written in Rust, FNM aims to provide the functionality of NVM but with significantly faster operation.
Installation
On macOS, you can install FNM using Homebrew:
brew install fnm
On Linux or Windows, you can use the install script:
curl -fsSL https://fnm.vercel.app/install | bash
Shell setup
After installation, you'll need to add FNM to your shell:
# Add this to load fnm
eval "$(fnm env --use-on-cd)"
This configuration enables automatic version switching based on .nvmrc
or
.node-version
files.
Basic usage
FNM's commands are intuitive for anyone familiar with NVM:
# Install a specific version
fnm install 16.13.0
# Install the latest LTS release
fnm install --lts
# Use a specific version
fnm use 16.13.0
# Set a default version
fnm default 16.13.0
# List installed versions
fnm list
# Create an alias
fnm alias 16.13.0 my-project
For a project that requires Node.js 16, you can create a .node-version
file:
16.13.0
With the --use-on-cd
option enabled, FNM will automatically switch to this
version when navigating to the project directory.
Advantages of FNM
FNM has several compelling features:
- Performance: Significantly faster than NVM due to its Rust implementation
- Cross-platform: Works on macOS, Linux, and Windows
- Compatibility: Supports
.nvmrc
and.node-version
files for easy migration - Minimal dependencies: Distributed as a single binary
Volta: The JavaScript Tool Manager
Volta takes a different approach by managing not just Node.js versions but your entire JavaScript toolchain. It describes itself as the "JavaScript Launcher" that ensures consistent tooling across projects and teams.
Installation
On macOS and Linux, install Volta using the installation script:
curl https://get.volta.sh | bash
On Windows, you can download the installer from the Volta website.
Basic usage
Volta simplifies Node.js version management with a few core commands:
# Install a specific Node.js version
volta install node@14.17.0
# Pin a Node.js version for a project
volta pin node@14.17.0
# Install and pin npm
volta install npm@7.24.0
# Install global tools
volta install yarn
volta install typescript
# List installed tools
volta list
Project-level configuration
When you pin tools to a project, Volta adds them to your package.json
:
{
"name": "my-project",
"version": "1.0.0",
"volta": {
"node": "16.13.0",
"npm": "8.1.0"
}
}
This configuration ensures everyone on the team uses the same versions automatically.
Advanced example: Development environment setup
For a new team member setting up a development environment, Volta makes the process seamless:
# Clone the repository
git clone https://github.com/company/project.git
cd project
# Install dependencies
npm install
# Volta automatically uses the correct Node and npm versions
# based on the project's package.json
No explicit version switching commands are needed - Volta handles it automatically.
Advantages of Volta
Volta offers several distinct benefits:
- Project-centric: Focuses on per-project tool management
- Team consistency: Ensures all developers use the same toolchain
- Global tools that work: Manages global tools without version conflicts
- Seamless experience: Automatically switches versions when changing directories
- Performance: Built in Rust for speed
asdf: The universal version manager
The asdf version manager takes a unique approach by supporting multiple languages and tools beyond just Node.js. With the right plugin, asdf can manage Ruby, Python, Go, and many other runtimes.
Installation
On macOS, install asdf using Homebrew:
brew install asdf
For other platforms, check the asdf documentation for detailed instructions.
Shell setup
Add asdf to your shell configuration:
. $(brew --prefix asdf)/libexec/asdf.sh
Installing the Node.js plugin
To manage Node.js versions, you need to install the Node.js plugin:
asdf plugin add nodejs
Basic usage
Once the plugin is installed, you can manage Node.js versions:
# Install a specific version
asdf install nodejs 16.13.0
# Set a global version
asdf global nodejs 16.13.0
# Set a local version for the current directory
asdf local nodejs 14.17.0
# List installed versions
asdf list nodejs
# List all available versions
asdf list all nodejs
Project configuration
For project-specific versions, create a .tool-versions
file:
nodejs 16.13.0
yarn 1.22.17
This file configures not just Node.js but any other tools managed by asdf.
Multi-language project example
For a full-stack project using both Node.js and Python:
nodejs 16.13.0
python 3.9.7
This approach ensures consistent language versions across your entire stack.
Advantages of asdf
asdf provides several benefits:
- Universal: Manages multiple languages and tools with a single interface
- Extensible: Plugin system for adding support for new tools
- Consistent workflow: Same commands work across all supported tools
- Active community: Regular updates and wide plugin ecosystem
nodenv: Ruby-inspired Node.js version management
nodenv draws inspiration from the popular rbenv Ruby version manager, applying similar principles to Node.js version management.
Installation
On macOS, install nodenv using Homebrew:
brew install nodenv
Shell setup
Add nodenv to your shell configuration:
eval "$(nodenv init -)"
Basic usage
nodenv uses a straightforward command structure:
# Install a specific version
nodenv install 16.13.0
# Set a global version
nodenv global 16.13.0
# Set a local version for the current directory
nodenv local 14.17.0
# List installed versions
nodenv versions
# Display the current active version
nodenv version
Project configuration
For project-specific Node.js versions, create a .node-version
file:
16.13.0
Installing global packages with nodenv
To install global packages with nodenv, you'll use the nodenv-specific npm:
npm install -g yarn
This installs the package in the current nodenv version's environment.
Advantages of nodenv
nodenv offers several distinct benefits:
- Familiarity: Similar workflow to rbenv for Ruby developers
- Lightweight: Minimal overhead and simple design
- Extensive plugin system: Enhance functionality through plugins
- Independent: Doesn't rely on npm for installation
Docker: Container-based Node.js version isolation
While not a traditional version manager, Docker provides complete environment isolation for Node.js applications. This approach ensures consistency across development, testing, and production environments.
Basic Node.js Docker setup
Create a Dockerfile
for your Node.js application:
FROM node:16.13.0-alpine
WORKDIR /app
COPY package*.json ./
RUN npm install
COPY . .
CMD ["npm", "start"]
Running the container
Build and run your Docker container:
# Build the image
docker build -t my-node-app .
# Run the container
docker run -p 3000:3000 my-node-app
Multi-stage builds for production
For production deployments, you might use a multi-stage build:
# Build stage
FROM node:16.13.0-alpine as build
WORKDIR /app
COPY package*.json ./
RUN npm ci
COPY . .
RUN npm run build
# Production stage
FROM node:16.13.0-alpine
WORKDIR /app
COPY --from=build /app/dist ./dist
COPY --from=build /app/node_modules ./node_modules
COPY package*.json ./
CMD ["npm", "run", "start:prod"]
Development with Docker Compose
For development, you might use Docker Compose to set up your environment:
version: '3'
services:
app:
build: .
volumes:
- .:/app
- /app/node_modules
ports:
- "3000:3000"
environment:
- NODE_ENV=development
command: npm run dev
Advantages of Docker
Using Docker for Node.js version management offers:
- Complete isolation: Full environment control, not just Node.js versions
- Production parity: Development environment matches production
- System independence: Works the same across all operating systems
- Team consistency: Everyone uses exactly the same environment
nvm-windows: NVM alternative for Windows
While NVM itself doesn't officially support Windows, nvm-windows provides similar functionality for Windows users.
Installation
Download the installer from the nvm-windows GitHub repository and run it.
Basic usage
nvm-windows uses commands similar to NVM:
# Install a specific version
nvm install 16.13.0
# Use a specific version
nvm use 16.13.0
# List installed versions
nvm list
# Set a default version
nvm alias default 16.13.0
Setting up a project
For projects requiring a specific Node.js version:
# Navigate to your project
cd my-project
# Create a .nvmrc file
echo "16.13.0" > .nvmrc
# Use the version specified in .nvmrc
nvm use
Advantages of nvm-windows
nvm-windows provides several benefits for Windows users:
- Native Windows support: Built specifically for Windows
- Familiar interface: Similar commands to NVM
- MSI installer: Easy installation process
- Active maintenance: Regular updates and fixes
Choosing the right Node.js version manager
With so many options available, how do you choose the right version manager for your needs? Consider these factors:
Performance considerations
If speed is your primary concern:
- FNM and Volta are the fastest options due to their Rust implementations
- N provides good performance with minimal overhead
- NVM and nodenv have more overhead due to their shell integration
Cross-platform requirements
If you work across different operating systems:
- FNM works well on macOS, Linux, and Windows
- Volta supports all major platforms
- asdf works on macOS and Linux
- nvm-windows is Windows-specific
Team collaboration
For teams working on multiple projects:
- Volta's package.json integration makes it excellent for teams
- asdf's .tool-versions supports multi-language projects
- Docker provides the most consistent environment across team members
Migration path from NVM
If you're currently using NVM:
- FNM has the most similar commands and supports .nvmrc files
- Volta requires adding configuration to package.json
- N requires a different command structure
Comparison table
Here's a quick comparison of the main features of each version manager:
Feature | N | FNM | Volta | asdf | nodenv | Docker | nvm-windows |
---|---|---|---|---|---|---|---|
Speed | Fast | Very Fast | Very Fast | Medium | Medium | Slow startup | Medium |
Cross-platform | ✅ | ✅ | ✅ | ✅* | ✅* | ✅ | Windows only |
Auto-switching | ❌ | ✅ | ✅ | ✅ | ✅ | N/A | ✅ |
.nvmrc support | ❌ | ✅ | ❌ | ❌ | ❌ | N/A | ✅ |
Multi-language | ❌ | ❌ | ❌** | ✅ | ❌ | ✅ | ❌ |
Implementation | Bash | Rust | Rust | Bash | Bash | N/A | Go |
- * Limited Windows support
- ** Supports JavaScript tools, not other languages
Final thoughts
Node.js version management is essential for modern JavaScript development, and while NVM has been the long-standing solution, these alternatives offer compelling features that might better suit your workflow. FNM provides speed and compatibility, Volta offers team-oriented toolchain management, asdf extends beyond Node.js to multiple languages, and Docker provides complete environment isolation.
For most developers, FNM offers the best balance of speed, features, and familiarity when migrating from NVM. Teams working on multiple JavaScript projects might benefit most from Volta's approach. If you work with multiple programming languages, asdf provides a unified interface for managing all your runtime versions.
The right choice ultimately depends on your specific needs, team structure, and existing workflow. Many developers even use multiple systems—perhaps Docker for production environments and FNM for local development. The good news is that most of these tools can coexist, allowing you to experiment and find the perfect fit for your development needs.
Meta Description: Discover the best NVM alternatives for Node.js version management, including FNM, Volta, asdf, and Docker, with installation guides and real-world usage examples.
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us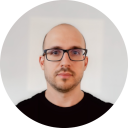
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github