JavaScript developers have more tools than ever for validating data, and picking the right one can shape how you write and maintain your code. Two popular libraries, Joi and Yup, tackle validation well but come from different origins and serve different needs.
Joi emerged from the Hapi.js ecosystem during Node.js’s rise, and it’s long been a go-to for server-side validation. With a rich feature set and rock-solid stability, it’s still trusted in many production backends today.
Yup, inspired by Joi, was purpose-built for the frontend, especially for React. Created by the Formik team, it focuses on making form validation intuitive and readable, with a clean, chainable API that frontend developers love.
Let's look at these two libraries to help you pick the right one for your project.
What is Joi?
Joi started as part of the Hapi.js framework but now stands on its own. It gives you powerful tools to check that your data follows the rules you set.
With Joi, you write validation rules by chaining methods together. This creates a readable way to express what valid data should look like.
Joi works great in Node.js backends, especially with Express, Hapi, and Fastify. It's mature, battle-tested, and has rich features for even the most complex validation needs.
What is Yup?
Yup emerged as a simpler alternative with a focus on form validation. The creators of Formik built it specifically to make React form validation less painful.
Like Joi, Yup uses a chain-based API to define validation rules. It is lightweight and integrates perfectly with React form libraries like Formik and React Hook Form.
It gives clear error messages that help users fix form inputs, making it a go-to for frontend developers.
Joi vs. Yup: a quick comparison
These two libraries approach validation differently, with distinct strengths that fit different use cases. Here's how they stack up:
Feature | Joi | Yup |
---|---|---|
Best for | Server-side and API validation | Frontend and form validation |
Size | Larger, more features | Smaller, more focused |
Error messages | Highly customizable | Simple, form-friendly |
Learning curve | Steeper, more options | Gentler, focused API |
Performance | Optimized for servers | Optimized for browsers |
Framework friends | Express, Hapi, Fastify | React, Formik, React Hook Form |
TypeScript support | Added later | Built with TypeScript in mind |
Schema definition
How you define validation rules plays a significant role in how readable and maintainable your code becomes. Joi and Yup follow similar patterns—but their differences can have a tangible impact on your development experience.
Joi gives you a rich vocabulary for describing data requirements. Its API is comprehensive, with methods for nearly any validation scenario:
const userSchema = Joi.object({
username: Joi.string().alphanum().min(3).max(30).required(),
email: Joi.string().email().required(),
birthYear: Joi.number().integer().min(1900).max(2023)
});
You can validate data against this schema with a simple method call:
const result = userSchema.validate({
username: 'john',
email: 'john@example.com',
birthYear: 1990
});
Joi's approach feels natural in JavaScript and gives you precise control over validation behavior.
Yup takes a similar approach but with a more streamlined API focused on common validation needs:
const userSchema = Yup.object({
username: Yup.string().min(3).max(30).required('Username is required'),
email: Yup.string().email('Invalid email').required('Email is required'),
birthYear: Yup.number().min(1900, 'Invalid year').max(2023, 'Invalid year')
});
Validation with Yup looks like this:
try {
const validData = userSchema.validateSync(userInput);
// use validData
} catch (error) {
// handle validation errors
}
Yup makes it easy to include custom error messages right in the schema definition, which is perfect for form validation where you need to show errors to users.
Validation patterns
Runtime validation directly impacts your app’s error handling strategy—and the way Joi and Yup approach it can shape how you design your code.
Joi's validation doesn't throw errors by default. Instead, it returns an object with the results:
const { error, value } = userSchema.validate(data);
if (error) {
console.error('Validation failed:', error.message);
} else {
// use valid data in value
saveUser(value);
}
This pattern keeps your code flow uninterrupted and gives you explicit control over error handling. You decide what happens when validation fails.
Yup takes a different route with a "throw first" approach:
try {
const validData = userSchema.validateSync(data);
saveUser(validData);
} catch (error) {
console.error('Validation failed:', error.message);
}
This fits well with React's error boundary pattern and makes error handling feel like other JavaScript exceptions.
Yup also offers a safer approach if you prefer not to use try/catch:
const result = userSchema.isValidSync(data);
if (result) {
// data is valid
} else {
// data is invalid
}
For async validation, both libraries offer solutions. Joi has validateAsync()
while Yup has asynchronous versions of its validation methods.
Handling complex validation scenarios
Real-world applications usually require more than just basic field checks—you’ll often need conditional logic, field dependencies, or custom validation rules. Both Joi and Yup can handle these advanced scenarios, but they do it in distinct ways that reflect their design philosophies.
Joi shines with built-in methods for complex rules. Take a look at conditional validation:
const schema = Joi.object({
type: Joi.string().valid('personal', 'business').required(),
// Only required for business accounts
taxId: Joi.when('type', {
is: 'business',
then: Joi.string().required(),
otherwise: Joi.forbidden()
})
});
For custom rules that don't fit the built-in methods, Joi offers the custom()
method:
const schema = Joi.object({
password: Joi.string().required(),
confirm: Joi.string().required()
}).custom((obj, helper) => {
if (obj.password !== obj.confirm) {
return helper.error('passwords.mismatch');
}
return obj;
});
Yup handles complex validation through more generic methods like when()
and test()
:
const schema = Yup.object({
type: Yup.string().oneOf(['personal', 'business']).required(),
taxId: Yup.string().when('type', {
is: 'business',
then: schema => schema.required('Tax ID is required for business accounts'),
otherwise: schema => schema.notRequired()
})
});
For custom validation, Yup uses the test()
method:
const schema = Yup.object({
password: Yup.string().required('Password is required'),
confirm: Yup.string().required('Please confirm your password')
.test('passwords-match', 'Passwords must match', function(value) {
return this.parent.password === value;
})
});
Both libraries can handle complex validation, but Joi often has more built-in methods for common patterns, while Yup favors a more generic approach with fewer specialized methods.
Type safety and integration
If you use TypeScript, how a validation library works with types affects your development experience. Joi and Yup handle TypeScript integration differently.
Joi added TypeScript support after it was already established. It includes type definitions that help you use the Joi API correctly, but you'll still define your data types separately:
// Define schema
const userSchema = Joi.object({
id: Joi.number().required(),
name: Joi.string().required()
});
// Define type separately
interface User {
id: number;
name: string;
}
// Use both in a function
function processUser(data: unknown): User {
const { value } = userSchema.validate(data);
return value as User;
}
This separation means you need to keep your types and schemas in sync manually.
Yup was built with TypeScript in mind and lets you derive types directly from your schemas:
// Define schema once
const userSchema = Yup.object({
id: Yup.number().required(),
name: Yup.string().required()
});
// Get type from schema
type User = Yup.InferType<typeof userSchema>;
// TypeScript knows the shape automatically
function processUser(data: unknown): User {
return userSchema.validateSync(data);
}
This keeps your validation and types in sync automatically. When you change your schema, your TypeScript types update too.
Yup's approach is particularly helpful with React form libraries:
// With React Hook Form
const { register, handleSubmit } = useForm<Yup.InferType<typeof formSchema>>({
resolver: yupResolver(formSchema)
});
For JavaScript projects, this difference doesn't matter. But for TypeScript users, especially those building React apps, Yup's integration can save time and prevent bugs.
Ecosystem and maturity
The community and tools around a library affect how easy it is to use in real projects. Joi and Yup have built different ecosystems that reflect their origins.
Joi has been around since 2012 and has deep roots in the Node.js world. It's battle-tested in production across countless applications. The Joi ecosystem includes:
- Official Express integration through
celebrate
- Native Hapi.js support
- Extensive documentation and community examples
- Many plugins for specialized validation needs
Here's how Joi fits into an Express API:
const { celebrate, Joi, errors } = require('celebrate');
const app = express();
app.post('/users',
celebrate({
body: Joi.object({
name: Joi.string().required(),
email: Joi.string().email().required()
})
}),
createUser
);
app.use(errors());
This mature ecosystem makes Joi a safe choice for server-side validation, especially in Node.js APIs.
Yup emerged from the React world and has built a strong ecosystem around frontend validation:
- First-class support in Formik
- Official integration with React Hook Form
- Growing collection of tutorials and examples
- Strong TypeScript community
Here's how Yup works with Formik:
import { Formik, Form, Field } from 'formik';
import * as Yup from 'yup';
const SignupForm = () => (
<Formik
initialValues={{ email: '', password: '' }}
validationSchema={Yup.object({
email: Yup.string().email('Invalid email').required('Required'),
password: Yup.string().min(8, 'Too short').required('Required')
})}
onSubmit={values => {
// Submit form
}}
>
{/* Form fields */}
</Formik>
);
Yup's ecosystem is newer but growing quickly, especially in the React community where form validation is a common need.
The right choice depends on your project context. For Node.js APIs, Joi's mature ecosystem provides proven solutions. For React forms, Yup's specialized integrations offer a more natural fit.
Performance considerations
Validation runs frequently in most apps, so performance matters—especially at scale or on mobile devices. Joi and Yup have different performance characteristics.
Joi was built for flexibility and completeness. To optimize performance, it offers options like schema caching and early termination:
// Optimize Joi for repeated validations
const schema = Joi.object({
/* schema definition */
}).options({
abortEarly: true, // Stop at first error
cache: true // Enable caching
});
In benchmarks, Joi performs well for complex server-side validations where its comprehensive features justify a slightly larger footprint.
Yup was designed to be lightweight, especially for browser environments. Its smaller bundle size (about 20KB minified) makes it more suitable for frontend applications:
// Optimize Yup validation
const result = schema.validateSync(data, {
abortEarly: true, // Stop at first error
stripUnknown: true // Remove extra fields
});
For most applications, both libraries are fast enough that performance won't be your deciding factor. But if you're building a performance-critical application:
- Choose Joi for complex server-side validation with many rules and conditions
- Choose Yup for browser-based validation where bundle size affects load times
Final thoughts
This article compared Joi and Yup, two strong validation libraries with different strengths. Joi is a great fit for server-side validation in Node.js, while Yup shines in React-based form handling.
For full-stack apps, using both can make sense—Joi on the backend, Yup on the frontend. Their similar APIs make switching between them easy.
Choose the one that fits your stack and validation needs best—both will serve you well.
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us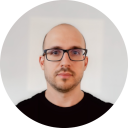
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github