The TypeScript ecosystem is growing fast; with it comes better, safer alternatives to old-school JavaScript ORMs. Two of the top options right now are Prisma and Drizzle. They both help you work with TypeScript databases, but take very different approaches.
Prisma uses a schema-first method. You define your database structure in a separate file, and Prisma turns that into a fully-typed client you can use in your app. This setup makes your code clean, consistent, and easy to work with, especially with TypeScript’s type checking and autocompletion.
Drizzle flips that around. It uses a code-first approach, so you define your database directly in your TypeScript code. There’s no extra file or generation step. This keeps things lightweight and gives you complete control, all while keeping type safety.
This guide will explain how Prisma and Drizzle work, their strengths, and when to use each so that you can choose the best fit for your TypeScript projects.
What is Prisma?
Prisma changes how you access data by keeping your database schema separate from your app code. Instead of writing everything in TypeScript, you describe your database using the Prisma Schema Language (PSL), a simple, readable format. Prisma uses that schema to generate a TypeScript client for you.
Since launching in 2016, Prisma has become a powerful toolkit built for TypeScript. Its generated client gives you full type safety, smart suggestions, and helpful error messages while you code. This makes your database queries more predictable and way less prone to bugs.
If you want a tool that handles the heavy lifting and makes it easy to work with databases in TypeScript, Prisma is a solid choice.
What is Drizzle?
Drizzle is a newer tool explicitly built for TypeScript. It focuses on speed, simplicity, and giving you complete control over how you define and use your database.
With Drizzle, you write your database schema directly in TypeScript. There’s no code generation or external files. When you update your schema, your TypeScript types update automatically—no extra steps needed.
Drizzle keeps things close to raw SQL. If you know SQL, you’ll feel right at home. Its query builder looks and feels like SQL, but with full type safety. Because it’s so lightweight, Drizzle works incredibly well in serverless apps where speed and bundle size really matter.
Drizzle vs. Prisma: a quick comparison
Selecting between these ORMs impacts both the development workflow and application performance. Each embodies a different philosophy about how developers should interact with databases in TypeScript applications.
The following comparison highlights the key differences to consider:
Feature | Drizzle | Prisma |
---|---|---|
Primary approach | Code-first with TypeScript | Schema-first with Prisma Schema |
Type safety | Native TypeScript inference | Generated TypeScript types |
Schema definition | TypeScript schema builders | Prisma Schema Language (PSL) |
Query building | SQL-like TypeScript API | Fluent method-based API |
Learning curve | Steeper for SQL beginners, natural for SQL experts | Gentler, more abstracted from SQL |
Migration support | Built-in with SQL migrations | Prisma Migrate with declarative migrations |
Relationship handling | Manual relation queries with joins | Built-in relation queries with nested fetching |
Performance | Highly optimized with minimal overhead | More abstraction with some performance tradeoffs |
Raw SQL support | First-class with type inference | Available but less integrated |
TypeScript integration | Zero runtime type checking | Generated client with runtime validation |
Database support | PostgreSQL, MySQL, SQLite, more with drivers | PostgreSQL, MySQL, SQLite, MongoDB, others |
Ecosystem & maturity | Newer, growing ecosystem | Established ecosystem with extensive tooling |
Bundle size | Lightweight, tree-shakable | Larger bundle with more features included |
Query debugging | Direct SQL inspection | Prisma logging and debugging tools |
Query building
The most significant difference between Prisma and Drizzle is how you write database queries. Each takes a very different view of how developers should interact with data.
Prisma hides SQL entirely and gives you a query API focused on working with entities. You write queries using objects that look and feel like regular JavaScript or TypeScript.
This makes it easy to reason about your data, especially if you’re not familiar with SQL:
// Prisma's entity-focused query API
const users = await prisma.user.findMany({
where: {
OR: [
{ email: { contains: 'example.com' } },
{ name: { startsWith: 'A' } }
],
posts: { some: { published: true } }
},
select: {
id: true,
email: true,
_count: { select: { posts: true } }
},
take: 10
});
This kind of query lets you work at a high level. You don’t think about joins or raw SQL—you just describe what you want, and Prisma handles the rest. Behind the scenes, it generates efficient SQL queries for you. For developers who aren’t deeply experienced with databases, this can make querying feel much more approachable and less error-prone.
Drizzle goes in the other direction. It leans into SQL concepts and gives you an API that looks and feels like writing SQL in TypeScript. If you already know SQL, it feels familiar and gives you more control over what’s happening:
// Drizzle's SQL-inspired query API
const users = await db.select({
id: users.id,
email: users.email,
postCount: sql`count(${posts.id})`.as('post_count')
})
.from(users)
.leftJoin(posts, eq(users.id, posts.authorId))
.where(
or(
like(users.email, '%example.com%'),
like(users.name, 'A%')
)
)
.groupBy(users.id, users.email)
.having(gt(sql`count(${posts.id})`, 0))
.limit(10);
This style gives you fine-grained control over the SQL that gets run, while still keeping everything type-safe. For teams or developers who already know SQL well, the learning curve is small, and you get the benefits of strong TypeScript typing without losing visibility into what’s happening under the hood.
Drizzle also includes a higher-level relational API when you want something simpler for common patterns:
// Drizzle's relational query API
const userWithPosts = await db.query.users.findFirst({
where: eq(users.id, 1),
with: {
posts: {
where: eq(posts.published, true),
limit: 5
}
}
});
These different styles reflect the core philosophies of the two tools. Prisma wants to abstract SQL away and let you work with your data like it’s part of your app logic.
Drizzle brings SQL right into your TypeScript code, giving you direct control with strong typing. Which one fits better depends on how comfortable your team is with SQL and what kind of control you want over your queries.
Schema definition
How you define database schemas with an ORM has a big impact on your overall developer experience. A good schema approach should feel natural, be easy to keep up to date, and work well with TypeScript’s type system.
Prisma and Drizzle take two very different paths here, showing their unique views on how TypeScript should interact with your database.
Prisma uses a schema-first approach with its own language called the Prisma Schema Language (PSL). You describe your database in a separate file, and Prisma turns that into a fully-typed TypeScript client. This setup gives you a clean and structured way to define your data:
datasource db {
provider = "postgresql"
url = env("DATABASE_URL")
}
generator client {
provider = "prisma-client-js"
}
model User {
id Int @id @default(autoincrement())
email String @unique
name String?
posts Post[]
profile Profile?
}
model Post {
id Int @id @default(autoincrement())
title String
published Boolean @default(false)
author User @relation(fields: [authorId], references: [id])
authorId Int
}
Once the schema is in place, Prisma generates a type-safe client you can use in your code:
// Using the generated Prisma client
import { PrismaClient } from '@prisma/client'
const prisma = new PrismaClient()
async function main() {
const user = await prisma.user.create({
data: {
email: 'alice@example.com',
name: 'Alice',
posts: {
create: { title: 'Hello World' }
}
},
include: { posts: true }
})
}
This setup clearly separates your schema from your application code. It gives you a single source of truth and access to tools like Prisma Studio, which lets you explore your data visually. The downside is that you’ll need to run a code generation step every time you change the schema.
Drizzle takes a different approach. Instead of using a separate schema language, it uses TypeScript itself to define your schema. That means your database setup lives right inside your TypeScript code. Drizzle skips code generation entirely and gives you instant feedback when you make changes:
import { pgTable, serial, text, varchar, boolean } from 'drizzle-orm/pg-core';
import { relations } from 'drizzle-orm';
export const users = pgTable('users', {
id: serial('id').primaryKey(),
email: varchar('email', { length: 255 }).notNull().unique(),
name: text('name')
});
export const posts = pgTable('posts', {
id: serial('id').primaryKey(),
title: varchar('title', { length: 255 }).notNull(),
published: boolean('published').default(false),
authorId: serial('author_id').references(() => users.id)
});
// Define relationships
export const usersRelations = relations(users, ({ many }) => ({
posts: many(posts)
}));
With Drizzle, you work directly with these schema objects in your queries:
// Using Drizzle with the defined schema
import { drizzle } from 'drizzle-orm/node-postgres';
import { Pool } from 'pg';
import { users, posts } from './schema';
const db = drizzle(new Pool({ connectionString: process.env.DATABASE_URL }));
async function main() {
// Insert user and get ID
const [user] = await db.insert(users)
.values({ email: 'alice@example.com', name: 'Alice' })
.returning();
// Insert related post
await db.insert(posts)
.values({ title: 'Hello World', authorId: user.id });
// Query with relations
const userWithPosts = await db.query.users.findFirst({
where: (users, { eq }) => eq(users.id, user.id),
with: { posts: true }
});
}
Drizzle relies on the TypeScript compiler to catch schema issues, so your app instantly reflects any schema changes without needing to rebuild or generate anything. This tight feedback loop speeds up development and keeps your setup simple. The trade-off is that you’ll need to handle relationship validation yourself, since Drizzle doesn’t enforce those rules through a separate schema system.
Transaction management
Transactions help make sure your database operations either all succeed or none do. This keeps your data consistent, even when things go wrong. Both Prisma and Drizzle support transactions, but their approaches reflect their overall design.
Prisma handles transactions in a way that fits with its entity-focused style. The $transaction
method supports two main patterns depending on what you need:
// Prisma interactive transactions
const result = await prisma.$transaction(async (tx) => {
// Check balance
const account = await tx.account.findUnique({
where: { id: accountId }
});
if (account.balance < amount) {
throw new Error('Insufficient funds');
}
// Update balance
const updatedAccount = await tx.account.update({
where: { id: accountId },
data: { balance: { decrement: amount } }
});
// Log transaction
const txRecord = await tx.transaction.create({
data: { amount, accountId, type: 'withdrawal' }
});
return { updatedAccount, txRecord };
});
You use the same API inside the transaction as you would outside it. This keeps things simple—you don’t have to learn a different way to write queries just because you’re in a transaction. Prisma also supports batch-style transactions, which are helpful when you want to run several independent queries as one atomic operation. That can improve performance by cutting down on round-trips to the database.
Drizzle takes a more SQL-style approach to transactions. You still get a transaction context, but it uses the same SQL-inspired query builder you’d use elsewhere:
// Drizzle transactions
const result = await db.transaction(async (tx) => {
// Check balance
const [account] = await tx.select()
.from(accounts)
.where(eq(accounts.id, accountId))
.limit(1);
if (!account || account.balance < amount) {
throw new Error('Insufficient funds');
}
// Update balance with SQL expression
await tx.update(accounts)
.set({
balance: sql`${accounts.balance} - ${amount}`
})
.where(eq(accounts.id, accountId));
// Log transaction
const [txRecord] = await tx.insert(transactions)
.values({ amount, accountId, type: 'withdrawal' })
.returning();
return { account, txRecord };
});
Drizzle keeps full query control inside the transaction, just like outside of it. If you're already comfortable with SQL, this approach feels natural and gives you the flexibility to write exactly what you need—still with full TypeScript support.
Relationship handling
Handling relationships between tables is one of the more complex parts of working with databases, especially when you're using TypeScript. How Prisma and Drizzle define and use relationships shows their different approaches to ORM design.
Prisma treats relationships as a core feature, built right into both the schema and the client. In the Prisma Schema Language, you define relationships clearly, with ownership and direction spelled out:
model User {
id Int @id @default(autoincrement())
posts Post[] // One-to-many relationship
profile Profile? // One-to-one relationship
}
model Post {
id Int @id @default(autoincrement())
author User @relation(fields: [authorId], references: [id])
authorId Int
}
model Profile {
id Int @id @default(autoincrement())
user User @relation(fields: [userId], references: [id])
userId Int @unique
}
This relationship-first design continues into how you write queries. You can easily create, read, and update related records using a clean, nested syntax:
// Creating with relationships
const user = await prisma.user.create({
data: {
email: 'alice@example.com',
posts: {
create: [{ title: 'Hello World' }]
},
profile: {
create: { bio: 'TypeScript dev' }
}
}
});
// Querying with relationship filters
const users = await prisma.user.findMany({
where: {
posts: {
some: { published: true }
}
}
});
The big advantage of Prisma's system is how much it simplifies complex data operations. You can create related records in a single call, update nested relationships, and keep everything in sync—all while Prisma makes sure your database stays consistent. You don’t need to write multiple queries or manage foreign keys manually.
Drizzle takes a different approach. It defines tables and relationships separately. You use the schema builder for tables, and a separate relations API to describe how those tables are connected:
// Table definitions
export const users = pgTable('users', {
id: serial('id').primaryKey(),
email: text('email').notNull()
});
export const posts = pgTable('posts', {
id: serial('id').primaryKey(),
title: text('title').notNull(),
authorId: integer('author_id').references(() => users.id)
});
// Relationship definitions
export const usersRelations = relations(users, ({ many }) => ({
posts: many(posts)
}));
export const postsRelations = relations(posts, ({ one }) => ({
author: one(users, {
fields: [posts.authorId],
references: [users.id]
})
}));
This setup is more explicit, and it separates structure from relationships. When it comes to queries, Drizzle provides a relational API that lets you load related data in a familiar way:
// Querying with relationships
const userWithPosts = await db.query.users.findFirst({
with: {
posts: {
where: eq(posts.published, true),
limit: 5
}
}
});
However, writing related data in Drizzle requires more manual work. It doesn’t support nested create or update operations like Prisma does. You need to insert or update records one at a time and manage relationships yourself. But when it comes to reading related data, Drizzle offers a clean and flexible API that’s similar in feel to Prisma’s.
The main difference is that Prisma builds relationships deeply into both reads and writes, while Drizzle keeps things more separate and hands-off. Prisma handles more for you, while Drizzle gives you more control and makes you be more explicit. Which one works better depends on how much automation versus manual control your project needs.
Migration support
As your app grows, your database schema needs to change too. Managing those changes safely is important, and both Prisma and Drizzle offer tools for handling migrations—each one reflecting the core ideas behind the tool.
Prisma Migrate uses a declarative system. Your Prisma schema file is the source of truth, and Prisma figures out what needs to change in the database. When you update the schema, you run a command to create a migration:
npx prisma migrate dev --name add_user_roles
That command generates a timestamped SQL file with the changes:
-- Migration: 20230512134523_add_user_roles
-- Generated at: 2023-05-12T13:45:23.000Z
ALTER TABLE "User" ADD COLUMN "role" TEXT NOT NULL DEFAULT 'USER';
This workflow focuses on the final result—you describe how the database should look, and Prisma handles the steps to get there. It fits with Prisma’s bigger goal: let you define data models and relationships, and take care of the rest.
For faster development, Prisma also has a db push
command. It applies schema changes directly to your database without creating a migration file, which is handy when you’re just experimenting or working in development:
npx prisma db push
Drizzle Kit takes a different route, following Drizzle’s code-first mindset. It generates migrations by comparing your current database state to your TypeScript-based schema definitions:
npx drizzle-kit generate
npx drizzle-kit push
This process creates SQL files based on what changed:
-- Migration: 0001_add_user_roles
CREATE TABLE IF NOT EXISTS "drizzle_migrations" (
"id" SERIAL PRIMARY KEY,
"hash" varchar(255) NOT NULL,
"created_at" timestamp DEFAULT now()
);
ALTER TABLE "users" ADD COLUMN IF NOT EXISTS "role" varchar(255) NOT NULL DEFAULT 'USER';
Drizzle’s system keeps everything in your TypeScript codebase. You don’t switch between a schema language and your app—your schema is your code.
That makes everything feel tightly connected, but it also means you need to be a bit more hands-on when managing schema changes.
Type safety and validation
A good TypeScript ORM needs to connect database types with TypeScript types clearly and safely. Prisma and Drizzle take very different paths to make that happen.
Prisma uses a generation-based approach. When you define your schema, you run a command that generates a fully-typed TypeScript client based on that schema:
npx prisma generate
This gives you types that match your database exactly:
// Types available after generation
import { PrismaClient, User, Post } from '@prisma/client'
// Client is fully typed based on schema
const prisma = new PrismaClient()
// TypeScript knows User has id, email, name, posts, etc.
const user: User = await prisma.user.findUnique({
where: { id: 1 }
})
// Error: Property 'nonExistent' does not exist on type 'User'
console.log(user.nonExistent)
Because the types are generated from the schema, Prisma can provide full type safety—even for complex relationships and nested operations. On top of that, Prisma adds runtime validation, so it checks that the data matches the expected shape before it runs the query.
Drizzle skips code generation entirely. Instead, it uses TypeScript’s type inference to figure out types directly from your schema definitions:
import { integer, pgTable, serial, text } from 'drizzle-orm/pg-core';
// Schema definition directly creates TypeScript types
export const users = pgTable('users', {
id: serial('id').primaryKey(),
email: text('email').notNull(),
name: text('name')
});
// Types are inferred without generation step
const [user] = await db.select().from(users).where(eq(users.id, 1));
// TypeScript knows user has id, email, and name
console.log(user.email);
// Error: Property 'nonExistent' does not exist on type...
console.log(user.nonExistent);
Because everything is defined in TypeScript, your types update immediately as your schema changes—no need to run a generate command. This gives you fast feedback during development, which can speed up your workflow. That said, type inference might not always be as complete or automatic for deep nested queries.
Both Prisma and Drizzle let you map custom database types to TypeScript types:
// Prisma custom type mappings
model Post {
id Int @id @default(autoincrement())
metadata Json // Maps to a TypeScript object type
tags String[] // Maps to string array
}
// Drizzle custom type mappings
export const posts = pgTable('posts', {
id: serial('id').primaryKey(),
metadata: jsonb('metadata').$type<{ views: number, likes: number }>(),
tags: array(text('tags'))
});
These different strategies reflect each tool’s overall philosophy. Prisma focuses on complete type safety through generated code, even if that means adding a build step. Drizzle focuses on keeping everything in sync through TypeScript’s type system itself, which makes development faster and simpler—but may require a bit more attention in more complex cases.
Final thoughts
Prisma and Drizzle take different but equally valid approaches to working with databases in TypeScript. Prisma’s schema-first style focuses on a great developer experience, with strong support for relationships and complex data models. Drizzle takes a lighter, code-first path that gives you more control and better performance, especially in apps where raw SQL speed matters.
Choosing between them comes down to what you value more: Prisma’s abstractions and tools, or Drizzle’s simplicity and closer connection to SQL. In practice, many teams use both—Prisma where ease of use is a priority, and Drizzle where performance is critical.
To dive deeper, check out the docs at prisma.io/docs and orm.drizzle.team/docs.
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us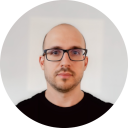
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github