What does all mean in Python?
In Python, the all()
function returns True
if all elements of an iterable (e.g. list, tuple, etc.) are true, and False
otherwise. For example:
>>> all([True, True, True])
True
>>> all([True, False, True])
False
An empty iterable returns True
.
>>> all([])
True
It can also be used on a generator object.
>>> all(i > 2 for i in [1, 2, 3, 4])
False
It is equivalent to the following code:
>>> def all(iterable):
... for element in iterable:
... if not element:
... return False
... return True
-
How to determine the type of an object in Python?
You can use the type() function to determine the type of an object in Python. For example: x = 10 print(type(x)) # Output: <class 'int'> y = "hello" print(type(y)) # Output: <class 'str'> z = [1,...
Questions -
Getting the class name of an instance in Python?
You can use the built-in type() function to get the class name of an instance in Python. For example: class MyClass: pass myinstance = MyClass() print(type(myinstance).name) This will output My...
Questions -
What's the best way to check for type in Python?
The built-in type() function is the most commonly used method for checking the type of an object in Python. For example, type(my_variable) will return the type of my_variable. Additionally, you can...
Questions -
What Does the “yield” Keyword Do in Python?
To better understand what yield does, you need first to understand what generator and iterable are. What is iterable When you use a list or list-like structure, you can read the values from the lis...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us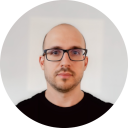
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github