What's the best way to check for type in Python?
The built-in type()
function is the most commonly used method for checking the type of an object in Python. For example, type(my_variable)
will return the type of my_variable
. Additionally, you can use the isinstance()
function to check if an object is an instance of a particular class or a subclass thereof. For example, isinstance(my_variable, int)
will return True
if my_variable
is an instance of the int
class.
Here's an example of using the type()
function:
x = 5
print(type(x)) # Output: <class 'int'>
y = "hello"
print(type(y)) # Output: <class 'str'>
You can also use isinstance()
to check if an object is an instance of a particular class or a subclass thereof. For example,
class A:
pass
class B(A):
pass
x = A()
y = B()
print(isinstance(x, A)) # Output: True
print(isinstance(y, A)) # Output: True
Here x is an instance of class A and y is an instance of class B, which is a subclass of A, so both would return True when we check if they are instances of A.
-
Difference between static and class methods in Python?
Class method To create a class method, use the @classmethod decorator. Class methods receive the class as an implicit first argument, just like an instance method receives the instance. The class m...
Questions -
What are metaclasses in Python?
In Python, a metaclass is the class of a class. It defines how a class behaves, including how it is created and how it manages its instances. A metaclass is defined by inheriting from the built-in ...
Questions -
How to determine the type of an object in Python?
You can use the type() function to determine the type of an object in Python. For example: x = 10 print(type(x)) # Output: <class 'int'> y = "hello" print(type(y)) # Output: <class 'str'> z = [1,...
Questions -
Getting the class name of an instance in Python?
You can use the built-in type() function to get the class name of an instance in Python. For example: class MyClass: pass myinstance = MyClass() print(type(myinstance).name) This will output My...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us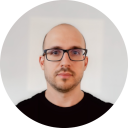
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github