Getting the class name of an instance in Python?
You can use the built-in type()
function to get the class name of an instance in Python. For example:
class MyClass:
pass
my_instance = MyClass()
print(type(my_instance).__name__)
This will output MyClass
.
You can also use the __class__
attribute on the object which returns the class of the instance.
class MyClass:
pass
my_instance = MyClass()
print(my_instance.__class__.__name__)
This will also output MyClass
.
-
Difference between static and class methods in Python?
Class method To create a class method, use the @classmethod decorator. Class methods receive the class as an implicit first argument, just like an instance method receives the instance. The class m...
Questions -
How can I add new keys to Python dictionary?
You can add a new key-value pair to a dictionary in Python by using the square brackets [] to access the key you want to add and then assigning it a value using the assignment operator =. For examp...
Questions -
How do I uppercase or lowercase a string in Python?
To uppercase a string in Python, you can use the upper() method of the string. For example: string = "hello" uppercasestring = string.upper() print(uppercasestring) # prints "HELLO" To lowercase a...
Questions -
What are metaclasses in Python?
In Python, a metaclass is the class of a class. It defines how a class behaves, including how it is created and how it manages its instances. A metaclass is defined by inheriting from the built-in ...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us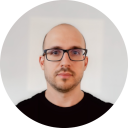
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github