How do I print colored text to the terminal in Python?
You can use ANSI escape codes to print colored text to the terminal in Python. Here is an example of how you can do this:
def colored_text(color, text):
colors = {
"red": "\033[91m",
"green": "\033[92m",
"yellow": "\033[93m",
"blue": "\033[94m",
"purple": "\033[95m",
"cyan": "\033[96m",
"white": "\033[97m"
}
if not color in colors:
raise ValueError("Invalid text color")
return f"{colors[color]}{text}\033[0m"
print(colored_text("red", "This text is red"))
print(colored_text("green", "This text is green"))
print(colored_text("yellow", "This text is yellow"))
print(colored_text("blue", "This text is blue"))
print(colored_text("purple", "This text is purple"))
print(colored_text("cyan", "This text is cyan"))
print(colored_text("white", "This text is white"))
This will print colored text to the terminal. Note that this will only work if the terminal supports ANSI escape codes.
-
How to Copy Files in Python?
To copy a file in Python, you can use the shutil module. Here is an example of how you can use the shutil.copy() function to copy a file: import shutil shutil.copy('/path/to/source/file', '/path/to...
Questions -
How to flatten a list in Python?
You can flatten a list in python using the following one-liner: flat_list = [item for sublist in l for item in sublist] In the example above, l is the list of lists that is to be flattened. The pre...
Questions -
Understanding slicing in Python?
Slicing is a way of extracting a specific part of an array. The syntax is following: mylist[start:end] # items start through end-1 mylist[start:] # items start through the rest of the array ...
Questions -
What does ** and * do for parameters in Python?
In Python, the * symbol is used to indicate that an argument can be passed to a function as a tuple. The ``** symbol is used to indicate that an argument can be passed to a function as a dictionary...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us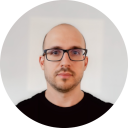
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github