How to Copy Files in Python?
To copy a file in Python, you can use the shutil
module. Here is an example of how you can use the shutil.copy()
function to copy a file:
import shutil
shutil.copy('/path/to/source/file', '/path/to/destination')
This will copy the file from the source location to the destination. If the destination is a directory, the file will be copied into that directory with the same name as the original file. If the destination is a file, it will be overwritten with the contents of the source file.
You can also use the shutil.copy2()
function, which is similar to shutil.copy()
, but also tries to preserve metadata like file permissions, modification times, and flags.
import shutil
shutil.copy2('/path/to/source/file', '/path/to/destination')
If you want to copy the contents of a directory, you can use the shutil.copytree()
function. This function will recursively copy the directory and all its subdirectories and files.
import shutil
shutil.copytree('/path/to/source/directory', '/path/to/destination')
-
What is the difference between str and repr in Python?
In Python, str is used to represent a string in a more readable format, while repr is used to represent a string in an unambiguous and official format. The main difference between the two is that s...
Questions -
How to flatten a list in Python?
You can flatten a list in python using the following one-liner: flat_list = [item for sublist in l for item in sublist] In the example above, l is the list of lists that is to be flattened. The pre...
Questions -
Understanding slicing in Python?
Slicing is a way of extracting a specific part of an array. The syntax is following: mylist[start:end] # items start through end-1 mylist[start:] # items start through the rest of the array ...
Questions -
How to check if a string contains a substring in Python?
There are multiple ways to check if a string contains a specific substring. Using in keyword You can use in keyword to check if a string contains a specific substring. The expression will return bo...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us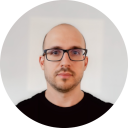
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github