What does ** and * do for parameters in Python?
In Python, the *
symbol is used to indicate that an argument can be passed to a function as a tuple. The ``** symbol is used to indicate that an argument can be passed to a function as a dictionary.
Here's an example of how you might use these symbols in a function definition:
def my_function(arg1, *args, **kwargs):
# arg1 is a required argument
# args is a tuple of optional positional arguments
# kwargs is a dictionary of optional keyword arguments
pass
You can then call the function like this:
my_function(1, 2, 3, 4, 5, keyword1=6, keyword2=7)
This would pass the following values to the function:
arg1 = 1
args = (2, 3, 4, 5)
kwargs = {'keyword1': 6, 'keyword2': 7}
You can use the *
and ``** syntax when calling a function as well. For example:
def my_function(arg1, arg2):
print(arg1)
print(arg2)
my_list = [1, 2]
my_dict = {'arg2': 3}
my_function(*my_list, **my_dict)
This would output the following:
1
3
The *
and `** symbols are used to unpack the values in the **
mylist** and **
mydict** variables, respectively, and pass them as individual arguments to the **
my_function`** function.
-
How to Copy Files in Python?
To copy a file in Python, you can use the shutil module. Here is an example of how you can use the shutil.copy() function to copy a file: import shutil shutil.copy('/path/to/source/file', '/path/to...
Questions -
How to flatten a list in Python?
You can flatten a list in python using the following one-liner: flat_list = [item for sublist in l for item in sublist] In the example above, l is the list of lists that is to be flattened. The pre...
Questions -
Understanding slicing in Python?
Slicing is a way of extracting a specific part of an array. The syntax is following: mylist[start:end] # items start through end-1 mylist[start:] # items start through the rest of the array ...
Questions -
What are metaclasses in Python?
In Python, a metaclass is the class of a class. It defines how a class behaves, including how it is created and how it manages its instances. A metaclass is defined by inheriting from the built-in ...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us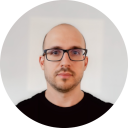
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github