How to convert string into datetime in Python?
To convert a string into a datetime object in Python, you can use the datetime.strptime()
function. This function allows you to specify the format of the input string, and it will return a datetime
object that corresponds to the input string.
Here is an example of how you can use strptime()
to convert a string into a datetime
object:
from datetime import datetime
date_string = "2022-01-25 13:00:00"
date_format = "%Y-%m-%d %H:%M:%S"
date_object = datetime.strptime(date_string, date_format)
print(date_object)
This will output the following datetime
object: 2022-01-25 13:00:00
.
You can also use the dateutil
module to parse strings into datetime
objects. The dateutil.parser.parse()
function is able to parse a wide range of string formats, and it can handle ambiguous strings (e.g. "Jan 1 2022") without the need to specify a format string. Here is an example of how you can use dateutil
to parse a string into a datetime
object:
from dateutil import parser
date_string = "2022-01-25 13:00:00"
date_object = parser.parse(date_string)
print(date_object)
This will output the following datetime
object: 2022-01-25 13:00:00
.
-
How To Color Python Logging Output?
If you are new to logging in Python, please feel free to start with our Introduction to Python logging to get started smoothly. Otherwise, here is how to color python logging output: 🔠Want to cent...
Questions -
How do I make function decorators and chain them together in Python?
In Python, a decorator is a design pattern to extend the functionality of a function without modifying its code. You can create a decorator function using the @decorator_function syntax, or by call...
Questions -
How to access the index in for loops in Python?
In python, if you are enumerating over a list using the for loop, you can access the index of the current value by using enumerate function. my_list = [1,2,3,4,5,6,7,8,9,10] for index, value in enu...
Questions -
How do I list all files in a directory using Python?
To list all files in a directory in Python, you can use the os module and its listdir() function. This function returns a list of all the files and directories in the specified directory. Here's an...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us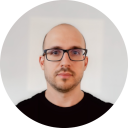
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github