How do I list all files in a directory using Python?
To list all files in a directory in Python, you can use the os
module and its listdir()
function. This function returns a list of all the files and directories in the specified directory.
Here's an example of how you can use listdir()
to list all files in a directory:
import os
# Get the list of all files in a directory
path = '/path/to/dir'
files = os.listdir(path)
# Print the files
for file in files:
print(file)
This will print the names of all the files in the specified directory, including the files in any subdirectories.
If you only want to list the files in the top-level directory and not in any subdirectories, you can use the isdir()
function from the os
module to check if each item in the list is a directory or a file. Here's an example of how you can do this:
import os
# Get the list of all files in the directory
path = '/path/to/dir'
files = os.listdir(path)
# Print the files
for file in files:
# Check if item is a file, not a directory
if not os.path.isdir(os.path.join(path, file)):
print(file)
This will print the names of all the files in the specified directory, but not the names of any subdirectories.
-
How to iterate over Python dictionaries using 'for' loops?
Let’s assume the following dictionary: my_dictionary = { 'key1': 'value1', 'key2': 'value2', 'key3': 'value3' } There are three main ways you can iterate over the dictionary. Iterate ov...
Questions -
What are metaclasses in Python?
In Python, a metaclass is the class of a class. It defines how a class behaves, including how it is created and how it manages its instances. A metaclass is defined by inheriting from the built-in ...
Questions -
How to flatten a list in Python?
You can flatten a list in python using the following one-liner: flat_list = [item for sublist in l for item in sublist] In the example above, l is the list of lists that is to be flattened. The pre...
Questions -
What Does the “yield” Keyword Do in Python?
To better understand what yield does, you need first to understand what generator and iterable are. What is iterable When you use a list or list-like structure, you can read the values from the lis...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us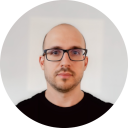
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github