How do I make function decorators and chain them together in Python?
In Python, a decorator is a design pattern to extend the functionality of a function without modifying its code. You can create a decorator function using the @decorator_function
syntax, or by calling the decorator function and passing the decorated function as an argument. For example:
def my_decorator(func):
def wrapper(*args, **kwargs):
print("Before function call")
result = func(*args, **kwargs)
print("After function call")
return result
return wrapper
@my_decorator
def my_function():
print("Inside function")
my_function()
# Output: "Before function call", "Inside function", "After function call"
You can chain decorators together by applying multiple decorators to a single function. The decorators will be applied in the order they are specified, with the innermost decorator being applied first. For example:
def decorator1(func):
def wrapper(*args, **kwargs):
print("Decorator 1")
return func(*args, **kwargs)
return wrapper
def decorator2(func):
def wrapper(*args, **kwargs):
print("Decorator 2")
return func(*args, **kwargs)
return wrapper
@decorator1
@decorator2
def my_function():
print("Inside function")
my_function()
# Output: "Decorator 2", "Decorator 1", "Inside function"
You can also chain decorators together by calling them one after the other.
my_function = decorator1(decorator2(my_function))
It's important to note that the decorator will not be applied when the function is defined, but when it is called.
-
How to Copy Files in Python?
To copy a file in Python, you can use the shutil module. Here is an example of how you can use the shutil.copy() function to copy a file: import shutil shutil.copy('/path/to/source/file', '/path/to...
Questions -
How can I add new keys to Python dictionary?
You can add a new key-value pair to a dictionary in Python by using the square brackets [] to access the key you want to add and then assigning it a value using the assignment operator =. For examp...
Questions -
What is the difference between str and repr in Python?
In Python, str is used to represent a string in a more readable format, while repr is used to represent a string in an unambiguous and official format. The main difference between the two is that s...
Questions -
What Does if name == “main”: Do in Python?
The if __name__ == "main" is a guarding block that is used to contain the code that should only run went the file in which this block is defined is run as a script. What it means is that if you run...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us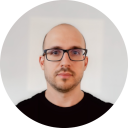
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github