How do I uppercase or lowercase a string in Python?
To uppercase a string in Python, you can use the upper()
method of the string. For example:
string = "hello"
uppercase_string = string.upper()
print(uppercase_string) # prints "HELLO"
To lowercase a string in Python, you can use the lower()
method of the string. For example:
string = "HELLO"
lowercase_string = string.lower()
print(lowercase_string) # prints "hello"
Both upper()
and lower()
are string methods, so they can be called on any string object in Python. They do not modify the original string, but instead return a new string with the uppercase or lowercase version of the original string.
-
How to convert string into datetime in Python?
To convert a string into a datetime object in Python, you can use the datetime.strptime() function. This function allows you to specify the format of the input string, and it will return a datetime...
Questions -
Convert bytes to a string in Python and vice versa?
To convert a string to bytes in Python, you can use the bytes function. This function takes two arguments: the string to encode and the encoding to use. The default encoding is utf-8. Here is an ex...
Questions -
How to check if a string contains a substring in Python?
There are multiple ways to check if a string contains a specific substring. Using in keyword You can use in keyword to check if a string contains a specific substring. The expression will return bo...
Questions -
How do I get a substring of a string in Python?
To get a substring of a string in Python, you can use the string slicing notation, which is string[start:end], where start is the index of the first character of the substring, and end is the index...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us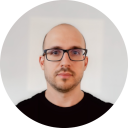
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github