How to upgrade all Python packages with pip?
You can use the pip install
command with the --upgrade
option to upgrade all packages in your Python environment.
Here's the basic syntax:
pip install --upgrade [package1] [package2] ...
To upgrade all packages, you can use the *
wildcard:
pip install --upgrade *
This will upgrade all packages in your environment to the latest version that is compatible with the other packages in your environment.
Alternatively, you can use the pip freeze
command to generate a requirements file that lists all the packages in your environment, and then use the pip install -r
command to upgrade all the packages at once:
pip freeze > requirements.txt
pip install --upgrade -r requirements.txt
This can be especially useful if you have a lot of packages installed, as it allows you to version control your package dependencies and easily replicate your environment on another machine.
-
Convert bytes to a string in Python and vice versa?
To convert a string to bytes in Python, you can use the bytes function. This function takes two arguments: the string to encode and the encoding to use. The default encoding is utf-8. Here is an ex...
Questions -
How do I get the last element of a list in Python?
To get the last element of a list in Python, you can use the negative indexing feature of the list data type. For example: mylist = [1, 2, 3, 4, 5] lastelement = mylist[-1] # lastelement will be 5...
Questions -
How do I parse a string to a float or int in Python?
To parse a string to a float in Python, you can use the float() function. This function takes a string as input and returns a floating point number constructed from it. For example: float('3.14') #...
Questions -
Understanding slicing in Python?
Slicing is a way of extracting a specific part of an array. The syntax is following: mylist[start:end] # items start through end-1 mylist[start:] # items start through the rest of the array ...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us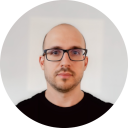
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github