How do I parse a string to a float or int in Python?
To parse a string to a float in Python, you can use the float()
function. This function takes a string as input and returns a floating point number constructed from it. For example:
float('3.14') # returns float 3.14
To parse a string to an integer in Python, you can use the int()
function. This function takes a string as input and returns an integer constructed from it. For example:
int('42') # returns integer 42
Note that these functions will raise a ValueError
if the string cannot be parsed to the desired type.
-
How can I safely create a nested directory in Python?
The most common way to safely create a nested directory in Python is using the pathlib or os modules. Using pathlib You can create a nested directory in python 3.5 or later using the Path and mkdir...
Questions -
How do I concatenate two lists in Python?
You can concatenate two lists in Python by using the + operator or the extend() method. Here is an example using the + operator: list1 = [1, 2, 3] list2 = [4, 5, 6] list3 = list1 + list2 print(list...
Questions -
How to check if a given key already exists in a dictionary in Python?
You can use the in keyword to check if a key exists in a dictionary. For example: my_dict = {'a': 1, 'b': 2, 'c': 3} if 'a' in my_dict: print("Key 'a' exists in the dictionary") if 'd' in my_di...
Questions -
How do I split Python list into equally-sized chunks?
To split a list into equally sized chunks, you can use the grouper function from the itertools module. Here's an example of how you can use it: from itertools import zip_longest def grouper(iterabl...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us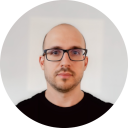
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github