How do I sort a dictionary by key or value?
To sort a dictionary by key in Python, you can use the sorted
function, like this:
d = {"a": 1, "b": 2, "c": 3}
sorted_d = sorted(d.items(), key=lambda x: x[0])
sorted_d
will now be a list of tuples, sorted by the key of each tuple.
If you want to sort the dictionary by value, you can use the following code:
d = {"a": 1, "b": 2, "c": 3}
sorted_d = sorted(d.items(), key=lambda x: x[1])
This will give you a list of tuples, sorted by the value of each tuple.
You can also use the OrderedDict
class from the collections
module to sort a dictionary by key or value. To do this, you can use the sorted
function to create a sorted list of keys or values, and then use the OrderedDict
constructor to create an OrderedDict
from the sorted list.
For example:
from collections import OrderedDict
d = {"a": 1, "b": 2, "c": 3}
sorted_d = OrderedDict(sorted(d.items(), key=lambda x: x[0]))
This will create an OrderedDict
with the keys sorted in ascending order.
You can also use the sort_by_key
and sort_by_value
functions from the dicttoolz
library to sort dictionaries by key or value, respectively.
from dicttoolz import sort_by_key, sort_by_value
d = {"a": 1, "b": 2, "c": 3}
sorted_d = sort_by_key(d)
This will return a new dictionary with the keys sorted in ascending order.
-
How to Copy Files in Python?
To copy a file in Python, you can use the shutil module. Here is an example of how you can use the shutil.copy() function to copy a file: import shutil shutil.copy('/path/to/source/file', '/path/to...
Questions -
Convert bytes to a string in Python and vice versa?
To convert a string to bytes in Python, you can use the bytes function. This function takes two arguments: the string to encode and the encoding to use. The default encoding is utf-8. Here is an ex...
Questions -
How to check if a string contains a substring in Python?
There are multiple ways to check if a string contains a specific substring. Using in keyword You can use in keyword to check if a string contains a specific substring. The expression will return bo...
Questions -
What Does if name == “main”: Do in Python?
The if __name__ == "main" is a guarding block that is used to contain the code that should only run went the file in which this block is defined is run as a script. What it means is that if you run...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us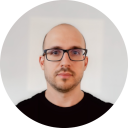
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github