How do I sort a list of dictionaries by a value of the dictionary in Python?
In Python, you can use the sorted()
function to sort a list of dictionaries by a specific value of the dictionary. The sorted()
function takes two arguments: the list to be sorted, and a key function that maps each element of the list to a value that is used for sorting.
For example, if you have a list of dictionaries called my_list
, and you want to sort it by the value of the 'age' key of each dictionary, you can use the following code:
sorted_list = sorted(my_list, key=lambda x: x['age'])
You can also use the itemgetter()
function from the operator
module as the key function, which is more efficient than using a lambda function:
from operator import itemgetter
sorted_list = sorted(my_list, key=itemgetter('age'))
If you want to sort the list in descending order, you can pass the reverse=True
argument to the sorted()
function:
sorted_list = sorted(my_list, key=lambda x: x['age'], reverse=True)
-
What are metaclasses in Python?
In Python, a metaclass is the class of a class. It defines how a class behaves, including how it is created and how it manages its instances. A metaclass is defined by inheriting from the built-in ...
Questions -
How to convert string into datetime in Python?
To convert a string into a datetime object in Python, you can use the datetime.strptime() function. This function allows you to specify the format of the input string, and it will return a datetime...
Questions -
How do I list all files in a directory using Python?
To list all files in a directory in Python, you can use the os module and its listdir() function. This function returns a list of all the files and directories in the specified directory. Here's an...
Questions -
What does ** and * do for parameters in Python?
In Python, the * symbol is used to indicate that an argument can be passed to a function as a tuple. The ``** symbol is used to indicate that an argument can be passed to a function as a dictionary...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us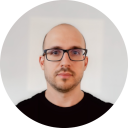
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github