How do I remove a trailing newline in Python?
You can use the rstrip()
method to remove a trailing newline from a string. Here is an example:
s = "Hello, World!\n"
s = s.rstrip()
print(s)
This will print "Hello, World!"
(without the newline).
Alternatively, you can use the strip()
method to remove both leading and trailing whitespace, including newlines. For example:
s = " Hello, World!\n"
s = s.strip()
print(s)
This will also print "Hello, World!"
(without the leading spaces or the newline).
-
How to read a file line-by-line in Python?
To read a file line-by-line in Python, you can use the following approach: with open('file.txt') as f: for line in f: print(line) This will open the file, read each line in the file, an...
Questions -
How to Copy Files in Python?
To copy a file in Python, you can use the shutil module. Here is an example of how you can use the shutil.copy() function to copy a file: import shutil shutil.copy('/path/to/source/file', '/path/to...
Questions -
Importing files from different folder in Python?
In Python, you can use the import statement to import modules or files from different folders. If the file you want to import is in a different folder than the script you are running, you will need...
Questions -
How do I delete a file or folder in Python?
You can use the os module to delete a file or folder in Python. To delete a file, you can use the os.remove() function. This function takes the file path as an argument and deletes the file at that...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us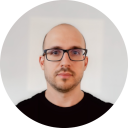
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github