How to read a file line-by-line in Python?
To read a file line-by-line in Python, you can use the following approach:
with open('file.txt') as f:
for line in f:
print(line)
This will open the file, read each line in the file, and then print the line. The with
statement is used to open the file and automatically close it when the block of code inside the with
statement is completed.
If you want to do something more complex with each line, you can use a loop to iterate over the lines in the file and process each line as needed.
with open('file.txt') as f:
for line in f:
# do something with the line
process_line(line)
You can also read all the lines of a file into a list and then process the lines using the list methods.
with open('file.txt') as f:
lines = f.readlines()
# do something with the lines
for line in lines:
process_line(line)
Both of these approaches have the advantage of reading the file one line at a time, which can be useful for very large files that don't fit in memory.
-
How to Copy Files in Python?
To copy a file in Python, you can use the shutil module. Here is an example of how you can use the shutil.copy() function to copy a file: import shutil shutil.copy('/path/to/source/file', '/path/to...
Questions -
How do I list all files in a directory using Python?
To list all files in a directory in Python, you can use the os module and its listdir() function. This function returns a list of all the files and directories in the specified directory. Here's an...
Questions -
Importing files from different folder in Python?
In Python, you can use the import statement to import modules or files from different folders. If the file you want to import is in a different folder than the script you are running, you will need...
Questions -
How do I delete a file or folder in Python?
You can use the os module to delete a file or folder in Python. To delete a file, you can use the os.remove() function. This function takes the file path as an argument and deletes the file at that...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us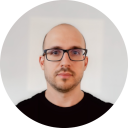
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github