How do I pad a string with zeroes in Python?
You can pad a string with zeros in Python by using the zfill()
method. This method adds zeros to the left of the string until it reaches the specified length.
Here's an example:
s = '123'
padded = s.zfill(5)
print(padded) # 00123
In this example, the string s
is padded with two zeros on the left to reach a length of 5.
-
Convert bytes to a string in Python and vice versa?
To convert a string to bytes in Python, you can use the bytes function. This function takes two arguments: the string to encode and the encoding to use. The default encoding is utf-8. Here is an ex...
Questions -
How do I get a substring of a string in Python?
To get a substring of a string in Python, you can use the string slicing notation, which is string[start:end], where start is the index of the first character of the substring, and end is the index...
Questions -
How do I uppercase or lowercase a string in Python?
To uppercase a string in Python, you can use the upper() method of the string. For example: string = "hello" uppercasestring = string.upper() print(uppercasestring) # prints "HELLO" To lowercase a...
Questions -
How to convert string into datetime in Python?
To convert a string into a datetime object in Python, you can use the datetime.strptime() function. This function allows you to specify the format of the input string, and it will return a datetime...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us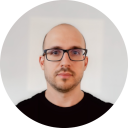
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github