Proper way to declare custom exceptions in modern Python?
In modern Python, the recommended way to declare custom exceptions is to create a new class that inherits from the built-in Exception
class. This class should define any additional attributes or methods that are specific to the exception being created. For example:
class MyException(Exception):
def __init__(self, message):
self.message = message
raise MyException("This is my custom exception.")
It is also common to define custom exceptions by subclassing from the built-in Exception
class or from a more specific built-in exception class such as ValueError
or TypeError
if it's more appropriate.
-
How to manually raising (throwing) an exception in Python?
To manually raise an exception in Python, use the raise statement. Here is an example of how to use it: def calculatepayment(amount, paymenttype): if paymenttype != "Visa" and paymenttype != "M...
Questions -
How To Log Uncaught Exceptions In Python?
For this, you can use the sys.excepthook that allows us to attach a handler for any unhandled exception: Creating a logger logger = logging.getLogger(name) logging.basicConfig(filename='example.log...
Questions -
How to determine the type of an object in Python?
You can use the type() function to determine the type of an object in Python. For example: x = 10 print(type(x)) # Output: <class 'int'> y = "hello" print(type(y)) # Output: <class 'str'> z = [1,...
Questions -
What's the best way to check for type in Python?
The built-in type() function is the most commonly used method for checking the type of an object in Python. For example, type(my_variable) will return the type of my_variable. Additionally, you can...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us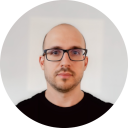
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github