How to manually raising (throwing) an exception in Python?
To manually raise an exception in Python, use the raise
statement. Here is an example of how to use it:
def calculate_payment(amount, payment_type):
if payment_type != "Visa" and payment_type != "Mastercard":
raise ValueError("Payment type must be Visa or Mastercard")
# Do the calculation using the provided amount and payment type
# ...
try:
calculate_payment(100, "Discover")
except ValueError as e:
print(e)
In this example, the calculate_payment
function raises a ValueError
exception if the payment_type
is not either "Visa" or "Mastercard". The try
-except
block catches the exception and prints the error message.
You can raise any exception type that you want by specifying the exception type as the first argument to the raise
statement. For example, to raise a TypeError
exception, you can use the following code:
raise TypeError("This is a TypeError exception")
Keep in mind that it is generally a good idea to only raise exceptions in exceptional circumstances. In most cases, it is better to use standard Python control structures (such as if
-else
or for
loops) to handle error conditions.
-
How to iterate over Python dictionaries using 'for' loops?
Let’s assume the following dictionary: my_dictionary = { 'key1': 'value1', 'key2': 'value2', 'key3': 'value3' } There are three main ways you can iterate over the dictionary. Iterate ov...
Questions -
How can I add new keys to Python dictionary?
You can add a new key-value pair to a dictionary in Python by using the square brackets [] to access the key you want to add and then assigning it a value using the assignment operator =. For examp...
Questions -
How Execute a Program or Call a System Command in Python?
In Python, you can execute a system command using the os.system. However, subprocess.run is a much better alternative. The official also documentation recommends subprocess.run over the os.system. ...
Questions -
How do I check if a list is empty in Python?
You can check if a list is empty by using the len() function to check the length of the list. If the length of the list is 0, then it is empty. Here's an example: my_list = [] if len(my_list) == 0:...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us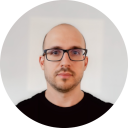
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github