How can I safely create a nested directory in Python?
The most common way to safely create a nested directory in Python is using the pathlib
or os
modules.
Using pathlib
You can create a nested directory in python 3.5 or later using the Path
and mkdir
method.
from pathlib import Path
p = Path('/root/directory/nested_directory')
p.mkdir(parents = True, exist_ok = True)
An important part of the code above is the parents
argument is set to True
. The default value is False
, meaning that if the parent directory (in this case /root/directory
) is not found, an error is thrown. If we set parents to True
, the error won’t be thrown and the non-existing parent directory will be created along with the nested directory.
The exist_ok
arguments ensure that an error won’t be thrown when the directory already exists.
Using os.makedirs
Another possible method is to use os.makedirs
function.
import os
os.makedirs('/root/directory/nested_directory')
The result will be the same as the previous one.
-
How To Color Python Logging Output?
If you are new to logging in Python, please feel free to start with our Introduction to Python logging to get started smoothly. Otherwise, here is how to color python logging output: 🔭 Want to cent...
Questions -
How to use the ternary conditional operator in Python?
The ternary conditional operator is a shortcut when writing simple conditional statements. If the condition is short and both true and false branches are short too, there is no need to use a multi-...
Questions -
What Does the “yield” Keyword Do in Python?
To better understand what yield does, you need first to understand what generator and iterable are. What is iterable When you use a list or list-like structure, you can read the values from the lis...
Questions -
What are metaclasses in Python?
In Python, a metaclass is the class of a class. It defines how a class behaves, including how it is created and how it manages its instances. A metaclass is defined by inheriting from the built-in ...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us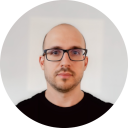
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github