How can I add new keys to Python dictionary?
You can add a new key-value pair to a dictionary in Python by using the square brackets []
to access the key you want to add and then assigning it a value using the assignment operator =
. For example:
my_dict = {'a': 1, 'b': 2}
my_dict['c'] = 3
print(my_dict)
This would output:
{'a': 1, 'b': 2, 'c': 3}
You can also use the update()
method to add multiple key-value pairs to a dictionary at once. For example:
my_dict = {'a': 1, 'b': 2}
my_dict.update({'c': 3, 'd': 4})
print(my_dict)
This would output:
{'a': 1, 'b': 2, 'c': 3, 'd': 4}
-
How to check if a string contains a substring in Python?
There are multiple ways to check if a string contains a specific substring. Using in keyword You can use in keyword to check if a string contains a specific substring. The expression will return bo...
Questions -
How to flatten a list in Python?
You can flatten a list in python using the following one-liner: flat_list = [item for sublist in l for item in sublist] In the example above, l is the list of lists that is to be flattened. The pre...
Questions -
What Does the “yield” Keyword Do in Python?
To better understand what yield does, you need first to understand what generator and iterable are. What is iterable When you use a list or list-like structure, you can read the values from the lis...
Questions -
What are metaclasses in Python?
In Python, a metaclass is the class of a class. It defines how a class behaves, including how it is created and how it manages its instances. A metaclass is defined by inheriting from the built-in ...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us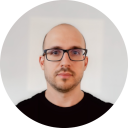
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github