How do I access environment variables in Python?
You can access environment variables in Python using the os
module.
Here's an example:
import os
# Access an environment variable
value = os.environ['VARIABLE_NAME']
# Set an environment variable
os.environ['VARIABLE_NAME'] = 'value'
You can also use the os.getenv
function to get the value of an environment variable. This function takes the name of the environment variable as an argument and returns the value of the variable as a string:
import os
value = os.getenv('VARIABLE_NAME')
If the environment variable is not set, os.getenv
returns None
. You can also specify a default value to be returned if the environment variable is not set:
import os
value = os.getenv('VARIABLE_NAME', 'default_value')
This can be useful if you want to use a default value if the environment variable is not set.
-
How do I get the current time in Python?
There are two ways you can get the current time in python. using the datetime object using the time module Using the datetime object First, you need to import the datetime module. Then by calling t...
Questions -
How Execute a Program or Call a System Command in Python?
In Python, you can execute a system command using the os.system. However, subprocess.run is a much better alternative. The official also documentation recommends subprocess.run over the os.system. ...
Questions -
What Does the “yield” Keyword Do in Python?
To better understand what yield does, you need first to understand what generator and iterable are. What is iterable When you use a list or list-like structure, you can read the values from the lis...
Questions -
What does ** and * do for parameters in Python?
In Python, the * symbol is used to indicate that an argument can be passed to a function as a tuple. The ``** symbol is used to indicate that an argument can be passed to a function as a dictionary...
Questions
Make your mark
Join the writer's program
Are you a developer and love writing and sharing your knowledge with the world? Join our guest writing program and get paid for writing amazing technical guides. We'll get them to the right readers that will appreciate them.
Write for us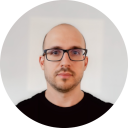
Build on top of Better Stack
Write a script, app or project on top of Better Stack and share it with the world. Make a public repository and share it with us at our email.
community@betterstack.comor submit a pull request and help us build better products for everyone.
See the full list of amazing projects on github